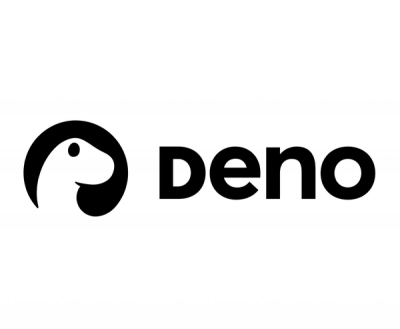
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@viamrobotics/prime-core
Advanced tools
@viamrobotics/prime-core
@viamrobotics/prime-core
is a collection of core Svelte components.
Add PRIME core using your package manager of choice:
pnpm add --save-dev @viamrobotics/prime-core
Install Tailwind. In the tailwind.config.js
, add the components to the content and include the theme:
import { theme } from '@viamrobotics/prime-core/theme';
import { plugins } from '@viamrobotics/prime-core/plugins';
/** @type {import('tailwindcss').Config} */
export default {
content: [
'./src/**/*.{html,js,svelte,ts}',
'./node_modules/@viamrobotics/prime-core/**/*.{ts,svelte}',
],
theme,
plugins,
};
Import the stylesheet. If you are using SvelteKit, you can do this in src/routes/+layout.svelte
.
import '@viamrobotics/prime-core/prime.css';
You can now use the components in your app:
<script lang="ts">
import { Badge } from '@viamrobotics/prime-core';
</script>
<Badge
variant="green"
label="Active"
/>
The playground can be used during development but is not used outside of the package.
pnpm install
pnpm -C packages/core dev
To lint and typecheck:
pnpm -C packages/core check # check svelte and lint
pnpm -C packages/core check-svelte # check svelte only
pnpm -C packages/core check-lint # check lint only with prettier and eslint
pnpm -C packages/core format # format with prettier
To test with vitest:
pnpm -C packages/core test # run once
pnpm -C packages/core test:watch # watch tests
For easier readability, we try to use a standard ordering for component composition. These are not strict rules, but more a guideline to follow. Implementation specifics may force you to go outside this guideline.
<!-- svelte options: https://svelte.dev/docs/special-elements#svelte-options -->
<svelte:options immutable />
<script
lang="ts"
context="module"
>
// exported types
export type MyType = 'thing' | 'other-thing';
</script>
<script lang="ts">
// external imports
import { onMount, createEventDispatcher } from 'svelte';
// internal imports
import { someLibraryFunction } from '$lib';
import { someSiblingFunction } from './sibling';
// prop declarations
/** A doc string for prop explaining what it does. */
export let prop: MyType | undefined = undefined;
// event dispatchers and other hooks
const dispatcher = createEventDispatcher<{
/** A doc string for the event handler. */
click: null; // void event
/** A doc string for the event handler. */
primitive: string | number | boolean; // simple primitive values
/** A doc string for the event handler. */
object: { id: string; next: number; }; // complex values
/** A doc string for the event handler. */
native: Event // native events (for pure atoms)
}>()
// internal fields
let someString = '';
// reactive variables
$: isThing = prop === 'thing';
// complex reactive variables
let counter = 0;
$: if (isThing) {
counter = someString.length;
if (counter > 10) {
counter = 10;
}
}
// single-line functions
const doSomething = () => someLibraryFunction();
// multi-line functions
const doSomethingElse = () => {
const shouldDoSomething = someString !== '';
someSiblingFunction(shouldDoSomething);
}
// reactive statements
$: {
someString = prop ? 'whoa' : 'no';
}
// lifecycle hooks
onMount(() => ...);
</script>
<!-- Your layout -->
<div class="border-black">
<!--
all slots should be named if there are multiple; otherwise a single slot
can be the default `<slot />`
-->
<slot name="title" />
<slot name="content" />
</div>
<style>
/* custom styles */
</style>
We keep tests in a __tests__
directory that is a sibling of the code being tested. These directories should only contain tests and test components.
Some components require test components to render slotted children, due to limitations with rendering slots using @testing-library
.
See:
FAQs
## Getting started
The npm package @viamrobotics/prime-core receives a total of 79 weekly downloads. As such, @viamrobotics/prime-core popularity was classified as not popular.
We found that @viamrobotics/prime-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.