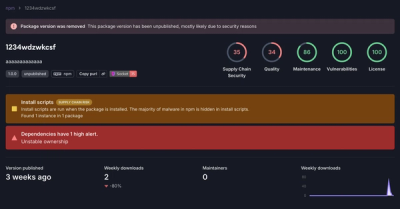
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@vonage/element-f
Advanced tools
A functional shim to custom element definition.
npm i @vonage/element-f
In order to define a custom-element, you only need one definition function:
import elementF from "@voange/element-f";
const MyElement = elementF(function(){
// --- Your logic goes here --
});
To tap into lifecycle events, this function can use the "life" event emitter:
const MyElement = elementF(function(life){
life.on('connected', ()=> console.log(`I'm Alive!`));
});
The following events are thrown:
connected
- Fired upon connectedCallback
disconnected
- Fired upon disconnectedCallback
attribute
- Fired when an observed attribute changes.To observe attributes, just add their list to elementF
call:
const MyElement = elementF(function(life){
life.on('attribute', ({ name, oldValue, newValue })=> {
// name can be "one" or "two"
});
}, ["one", "two"]);
To define a custom element using the standard class notation:
class MyButton extends HTMLElement {
constructor(){
super();
console.log(`I'm alive!`);
}
static get observedAttributes(){
return ['disabled'];
}
attributeChangedCallback(name, oldValue, newValue) {
this.classList.toggle('disabled', newValue);
}
connectedCallback() {
this.innerHTML = "<b>I'm an x-foo-with-markup!</b>";
}
}
Defining the same element using element-f would look like this:
const MyButton = elementF(function(life){
life.on('connected', ()=> {
this.innerHTML = "<b>I'm an x-foo-with-markup!</b>";
});
life.on('attribute', ({ name, newValue, oldValue })=> {
this.classList.toggle('disabled', newValue);
});
console.log(`I'm alive!`);
}, ['disabled']);
FAQs
A functional shim to custom element definition
The npm package @vonage/element-f receives a total of 5 weekly downloads. As such, @vonage/element-f popularity was classified as not popular.
We found that @vonage/element-f demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 14 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.