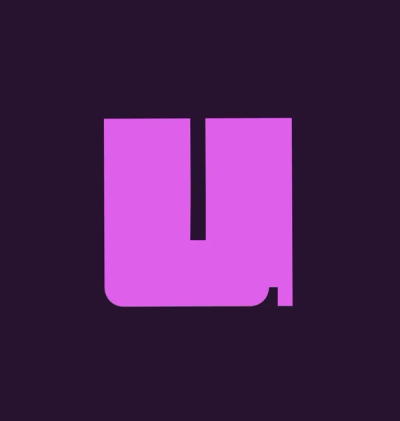
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@vtex/address-form
Advanced tools
A React component that renders VTEX's address forms
$ npm install @vtex/address-form
This component handles the input validation based on the country rules provided. It also calls the Postal Code service to autocomplete the address fields.
It provides an onChangeAddress()
function to the child function.
When a field change its value, it should call the function with an object like so:
onChangeAddress({
street: { value: 'newValueHere' }
})
You can also call it with more than one field:
onChangeAddress({
street: { value: 'newValueHere' },
number: { value: 'newValueHere' }
})
cors
: (default: false
) If the app is running outside the VTEX servers.accountName
: This parameter it's only used when the cors
prop is true
. The account name of the store, to be used by the Postal Code Service.address
: The current address in the shape of AddressShapeWithValidation
rules
: The selected country rulesonChangeAddress
: Callback function to be called when a field has changedchildren
: A callback child functionautoCompletePostalCode
: (default: true
) Should auto complete address when postal code is validAddressContainer.propTypes = {
cors: PropTypes.bool,
accountName: PropTypes.string,
address: AddressShapeWithValidation,
rules: PropTypes.object.isRequired,
onChangeAddress: PropTypes.func.isRequired,
children: PropTypes.func.isRequired,
autoCompletePostalCode: PropTypes.bool,
}
<AddressContainer
address={address}
rules={rules}
onChangeAddress={this.handleAddressChange}
>
{onChangeAddress =>
<YourComponent onChange={onChangeAddress}>
}
</AddressContainer>
Renders a select that shows all the countries options.
Input
: (default: @vtex/address-form/lib/DefaultInput
) A custom React component to render the inputsaddress
: The current address in the shape of AddressShapeWithValidation
shipsTo
: An array of an object of shape { value: String, label: String }
onChangeAddress
: Callback function to be called when a field has changedCountrySelector.propTypes = {
Input: PropTypes.func,
address: AddressShapeWithValidation,
shipsTo: PropTypes.array.isRequired,
onChangeAddress: PropTypes.func.isRequired,
}
<AddressContainer
address={address}
rules={selectedRules}
onChangeAddress={this.handleAddressChange}
>
{onChangeAddress =>
(<div>
<CountrySelector
Input={DefaultInput}
address={address}
shipsTo={shipsTo}
onChangeAddress={onChangeAddress}
/>
</div>
)
}
</AddressContainer>
Renders an address form base on rules of the selected country.
Input
: (default: @vtex/address-form/lib/DefaultInput
) A custom React component to render the inputsaddress
: The current address in the shape of AddressShapeWithValidation
omitPostalCodeFields
: (default: true
) Option to omit or not the fields that are rendered by <PostalCodeGetter/>
omitAutoCompletedFields
: (default: true
) Option to omit or not the fields that were auto completedrules
: The rules of the selected countryonChangeAddress
: Callback function to be called when a field has changedAddressForm.propTypes = {
Input: PropTypes.func,
address: AddressShapeWithValidation,
omitPostalCodeFields: PropTypes.bool,
omitAutoCompletedFields: PropTypes.bool,
rules: PropTypes.object.isRequired,
onChangeAddress: PropTypes.func.isRequired,
}
<AddressContainer
address={address}
rules={selectedRules}
onChangeAddress={this.handleAddressChange}
>
{onChangeAddress =>
(<div>
<AddressForm
Input={DefaultInput}
address={address}
rules={selectedRules}
onChangeAddress={onChangeAddress}
/>
</div>
)
}
</AddressContainer>
Renders a summary of the address.
address
: The current address in the shape of AddressShape
rules
: The rules of the selected countrygiftRegistryDescription
: If the address is from a gift list, pass the description of it herecanEditData
: (default: true
) Boolean that tells if the data is masked, the same property of the orderForm
.onClickMaskedInfoIcon
: Function that handles when the icon of masked info is clickedAddressSummary.propTypes = {
canEditData: PropTypes.bool,
address: AddressShape.isRequired,
rules: PropTypes.object.isRequired,
giftRegistryDescription: PropTypes.string,
onClickMaskedInfoIcon: PropTypes.func,
}
<AddressSummary
address={removeValidation(address)}
rules={selectedRules}
onClickMaskedInfoIcon={this.handleClickMaskedInfoIcon}
/>
Renders the requried components to get the postal code of an address. Some countries you can get the postal code by one simple and direct input, but in other countries we must render some select fields so that the user may select a place that we assign a defined postal code.
Input
: (default: @vtex/address-form/lib/DefaultInput
) A custom React component to render the inputsaddress
: The current address in the shape of AddressShapeWithValidation
rules
: The rules of the selected countryonChangeAddress
: Callback function to be called when a field has changedPostalCodeGetter.propTypes = {
Input: PropTypes.func,
address: AddressShapeWithValidation,
rules: PropTypes.object.isRequired,
onChangeAddress: PropTypes.func.isRequired,
}
<AddressContainer
address={address}
rules={selectedRules}
onChangeAddress={this.handleAddressChange}
>
{onChangeAddress =>
(<div>
<PostalCodeGetter
Input={DefaultInput}
address={address}
rules={selectedRules}
onChangeAddress={onChangeAddress}
/>
</div>
)
}
</AddressContainer>
Renders a summary of the fields that were auto completed by the postal code or by the geolocation.
children
: Node element that can be rendered for the "Edit" elementaddress
: The current address in the shape of AddressShapeWithValidation
rules
: The rules of the selected countryonChangeAddress
: Callback function to be called when a field has changedAutoCompletedFields.propTypes = {
children: PropTypes.node.isRequired,
address: AddressShapeWithValidation,
rules: PropTypes.object.isRequired,
onChangeAddress: PropTypes.func.isRequired,
}
<AddressContainer
address={address}
rules={selectedRules}
onChangeAddress={this.handleAddressChange}
>
{onChangeAddress =>
(<div>
<AutoCompletedFields
address={address}
rules={selectedRules}
onChangeAddress={onChangeAddress}
>
<a className="link-edit" id="force-shipping-fields">
{intl.formatMessage({ id: 'address-form.edit' })}
</a>
</AutoCompletedFields>
</div>
)
}
</AddressContainer>
Important!: The Geolocation Components are recommended to be loaded async using dynamic import.
This component handles the loading of the Google Maps JavaScript Library.
It provides an object with { loading, googleMaps }
to the child function.
loading
: Loading when thegoogleMaps
: Google Maps JavaScript library objectchildren
: A callback child functionapiKey
: The Google Maps API Keylocale
: The user localeGoogleMapsContainer.propTypes = {
children: PropTypes.func.isRequired,
apiKey: PropTypes.string.isRequired,
locale: PropTypes.string.isRequired,
}
<GoogleMapsContainer apiKey={googleMapsAPIKey} locale={locale}>
{({ loading, googleMaps }) => {
...
}
</GoogleMapsContainer>
Renders an input with the Google Maps auto complete feature. When the user selects an option suggested, it fills the address fields using the specified rules defined by the country and its geocoordinates.
Input
: (default: @vtex/address-form/lib/DefaultInput
) A custom React component to render the inputsinputProps
: (default: {}
) An object with props to be passed down to the Input componentrules
: The selected country rulesaddress
: The current address in the shape of AddressShapeWithValidation
onChangeAddress
: Callback function to be called when a field has changedloadingGoogle
: Boolean if the Google Maps JavaScript Library is loadinggoogleMaps
: The Google Maps JavaScript Library objectGeolocationInput.propTypes = {
Input: PropTypes.func,
inputProps: PropTypes.object,
rules: PropTypes.object.isRequired,
address: AddressShapeWithValidation.isRequired,
onChangeAddress: PropTypes.func.isRequired,
loadingGoogle: PropTypes.bool,
googleMaps: PropTypes.object,
}
<GoogleMapsContainer apiKey={googleMapsAPIKey} locale={locale}>
{({ loading, googleMaps }) =>
(<div>
<GeolocationInput
loadingGoogle={loading}
googleMaps={googleMaps}
address={address}
rules={selectedRules}
onChangeAddress={onChangeAddress}
/>
</div>
)
}
</GoogleMapsContainer>
Renders a Google Map with a marker at the point of the address' geocoordinates.
loadingElement
: (default: <div>Loading...</div>
) Node element that is rendered while it's loadingmapProps
: Props passed to the map elementgeoCoordinates
: Address` geocoordinatesrules
: The selected country rulesonChangeAddress
: Callback function to be called when a field has changedloadingGoogle
: Boolean if the Google Maps JavaScript Library is loadinggoogleMaps
: The Google Maps JavaScript Library objectMap.propTypes = {
loadingElement: PropTypes.node,
mapProps: PropTypes.object,
geoCoordinates: PropTypes.array.isRequired,
rules: PropTypes.object.isRequired,
onChangeAddress: PropTypes.func.isRequired,
loadingGoogle: PropTypes.bool,
googleMaps: PropTypes.object,
}
<GoogleMapsContainer apiKey={googleMapsAPIKey} locale={locale}>
{({ loading, googleMaps }) =>
(<div>
{address.geoCoordinates &&
address.geoCoordinates.valid &&
address.geoCoordinates.value.length === 2 &&
<Map
loadingGoogle={loading}
googleMaps={googleMaps}
geoCoordinates={address.geoCoordinates.value}
rules={selectedRules}
onChangeAddress={onChangeAddress}
mapProps={{
style: {
height: '120px',
marginBottom: '10px',
width: '260px',
},
}}
/>}
</div>)}
</GoogleMapsContainer>
address
: An address in the shape of AddressShape
address
: An address in the shape of AddressShapeWithValidation
const address = {
addressId: '10',
addressType: 'residential',
city: null,
complement: null,
country: 'BRA',
geoCoordinates: [],
neighborhood: null,
number: null,
postalCode: null,
receiverName: null,
reference: null,
state: null,
street: null,
addressQuery: null,
}
addValidation(address)
// {
// addressId: { value: '10' },
// addressType: { value: 'residential' },
// city: { value: null },
// complement: { value: null },
// country: { value: 'BRA' },
// geoCoordinates: { value: [] },
// neighborhood: { value: null },
// number: { value: null },
// postalCode: { value: null },
// receiverName: { value: null },
// reference: { value: null },
// state: { value: null },
// street: { value: null },
// addressQuery: { value: null },
// }
address
: An address in the shape of AddressShapeWithValidation
address
: An address in the shape of AddressShape
const address = {
addressId: { value: '10' },
addressType: { value: 'residential' },
city: { value: null },
complement: { value: null },
country: { value: 'BRA' },
geoCoordinates: { value: [] },
neighborhood: { value: null },
number: { value: null },
postalCode: { value: null },
receiverName: { value: null },
reference: { value: null },
state: { value: null },
street: { value: null },
addressQuery: { value: null },
}
removeValidation(address)
// {
// addressId: '10',
// addressType: 'residential',
// city: null,
// complement: null,
// country: 'BRA',
// geoCoordinates: [],
// neighborhood: null,
// number: null,
// postalCode: null,
// receiverName: null,
// reference: null,
// state: null,
// street: null,
// addressQuery: null,
// }
address
: An address in the shape of AddressShapeWithValidation
rules
: The selected country rulesvalid
: A boolean if the address is valid or notaddress
: An address in the shape of AddressShapeWithValidation
with fields validatedconst address = {
addressId: { value: '10' },
addressType: { value: 'residential' },
city: { value: 'Rio de Janeiro' },
complement: { value: null },
country: { value: 'BRA' },
geoCoordinates: { value: [] },
neighborhood: { value: 'Botafogo' },
number: { value: '300' },
postalCode: { value: '22231000' },
receiverName: { value: 'João' },
reference: { value: null },
state: { value: 'RJ' },
street: { value: '' },
addressQuery: { value: null },
}
isValidAddress(address, rules)
// {
// valid: false,
// address: {
// addressId: { value: '10' },
// addressType: { value: 'residential' },
// city: { value: 'Rio de Janeiro' },
// complement: { value: null },
// country: { value: 'BRA' },
// geoCoordinates: { value: [] },
// neighborhood: { value: 'Botafogo' },
// number: { value: '300' },
// postalCode: { value: '22231000' },
// receiverName: { value: 'João' },
// reference: { value: null },
// state: { value: 'RJ' },
// street: { value: '', valid: false, focus: true, reason: 'ERROR_EMPTY_FIELD' },
// addressQuery: { value: null },
// }
// }
validateField(value, name, address, rules)
value
: Value of the fieldname
: Name of the fieldaddress
: An address in the shape of AddressShapeWithValidation
rules
: The selected country rulesAn object with the properties:
valid
: A boolean if the field is valid or notreason
: An error constantconst address = {
addressId: { value: '10' },
addressType: { value: 'residential' },
city: { value: '' },
complement: { value: null },
country: { value: 'BRA' },
geoCoordinates: { value: [] },
neighborhood: { value: '' },
number: { value: '' },
postalCode: { value: '222' },
receiverName: { value: 'João' },
reference: { value: null },
state: { value: '' },
street: { value: '' },
addressQuery: { value: null },
}
validateField('222', 'postalCode', address, rules)
// {
// valid: false,
// reason: 'ERROR_POSTAL_CODE'
// }
This folder provides JSONs of translations commonly used by the Address Form in a flat format.
This folder provides the country rules modules.
Each country has their own set of rules on how an address form should be rendered. They specify:
If a selected country does not exists in the country folder. Use the default.js
FAQs
address-form React component
The npm package @vtex/address-form receives a total of 0 weekly downloads. As such, @vtex/address-form popularity was classified as not popular.
We found that @vtex/address-form demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 97 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.