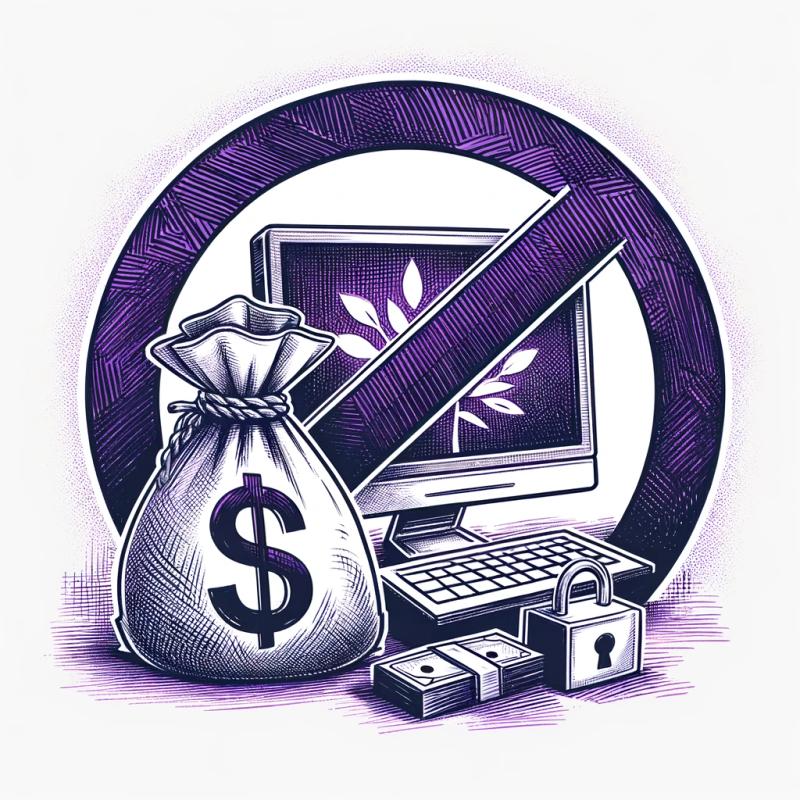
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
@vue/babel-plugin-jsx
Advanced tools
Changelog
1.1.0
2021-09-30
Readme
To add Vue JSX support.
English | 简体中文
Install the plugin with:
npm install @vue/babel-plugin-jsx -D
Then add the plugin to .babelrc:
{
"plugins": ["@vue/babel-plugin-jsx"]
}
Type: boolean
Default: false
transform on: { click: xx }
to onClick: xxx
Type: boolean
Default: false
enable optimization or not. It's not recommended to enable it If you are not familiar with Vue 3.
Type: (tag: string) => boolean
Default: undefined
configuring custom elements
Type: boolean
Default: true
merge static and dynamic class / style attributes / onXXX handlers
Type: boolean
Default: true
Whether to enable object slots
(mentioned below the document) syntax". It might be useful in JSX, but it will add a lot of _isSlot
condition expressions which increase your bundle size. And v-slots
is still available even if enableObjectSlots
is turned off.
Type: string
Default: createVNode
Replace the function used when compiling JSX expressions.
functional component
const App = () => <div>Vue 3.0</div>;
with render
const App = {
render() {
return <div>Vue 3.0</div>;
},
};
import { withModifiers, defineComponent } from "vue";
const App = defineComponent({
setup() {
const count = ref(0);
const inc = () => {
count.value++;
};
return () => (
<div onClick={withModifiers(inc, ["self"])}>{count.value}</div>
);
},
});
Fragment
const App = () => (
<>
<span>I'm</span>
<span>Fragment</span>
</>
);
const App = () => <input type="email" />;
with a dynamic binding:
const placeholderText = "email";
const App = () => <input type="email" placeholder={placeholderText} />;
v-show
const App = {
data() {
return { visible: true };
},
render() {
return <input v-show={this.visible} />;
},
};
v-model
Note: You should pass the second param as string for using
arg
.
<input v-model={val} />
<input v-model={[val, ["modifier"]]} />
<A v-model={[val, "argument", ["modifier"]]} />
Will compile to:
h(A, {
argument: val,
argumentModifiers: {
modifier: true,
},
"onUpdate:argument": ($event) => (val = $event),
});
v-models
Note: You should pass a Two-dimensional Arrays to v-models.
<A v-models={[[foo], [bar, "bar"]]} />
<A
v-models={[
[foo, "foo"],
[bar, "bar"],
]}
/>
<A
v-models={[
[foo, ["modifier"]],
[bar, "bar", ["modifier"]],
]}
/>
Will compile to:
h(A, {
modelValue: foo,
modelModifiers: {
modifier: true,
},
"onUpdate:modelValue": ($event) => (foo = $event),
bar: bar,
barModifiers: {
modifier: true,
},
"onUpdate:bar": ($event) => (bar = $event),
});
custom directive
const App = {
directives: { custom: customDirective },
setup() {
return () => <a v-custom={[val, "arg", ["a", "b"]]} />;
},
};
Note: In
jsx
,v-slot
should be replace withv-slots
const A = (props, { slots }) => (
<>
<h1>{ slots.default ? slots.default() : 'foo' }</h1>
<h2>{ slots.bar?.() }</h2>
</>
);
const App = {
setup() {
const slots = {
bar: () => <span>B</span>,
};
return () => (
<A v-slots={slots}>
<div>A</div>
</A>
);
},
};
// or
const App = {
setup() {
const slots = {
default: () => <div>A</div>,
bar: () => <span>B</span>,
};
return () => <A v-slots={slots} />;
},
};
// or you can use object slots when `enableObjectSlots` is not false.
const App = {
setup() {
return () => (
<>
<A>
{{
default: () => <div>A</div>,
bar: () => <span>B</span>,
}}
</A>
<B>{() => "foo"}</B>
</>
);
},
};
tsconfig.json
:
{
"compilerOptions": {
"jsx": "preserve"
}
}
![]() Ant Design Vue |
![]() Vant |
![]() Element Plus |
Vue Json Pretty |
This repo is only compatible with:
FAQs
Babel plugin for Vue 3 JSX
We found that @vue/babel-plugin-jsx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).