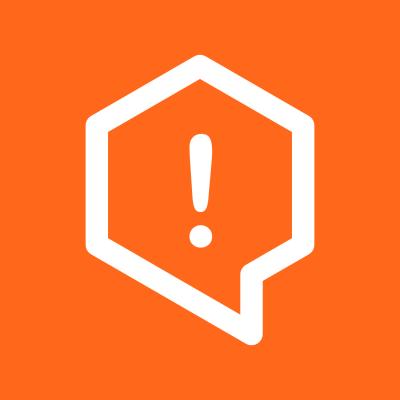
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@vuedx/template-ast-types
Advanced tools
A collection of utility functions for Vue template AST traversal, transformation, assertion and creation.
npm add @vuedx/template-ast-types
Create AST Node
Signature:
declare function createSimpleExpression(
content: SimpleExpressionNode['content'],
isStatic: SimpleExpressionNode['isStatic'],
loc?: SourceLocation,
isConstant?: boolean,
): SimpleExpressionNode
Parameter | Type | Description |
---|---|---|
content | SimpleExpressionNode['content'] | - |
isStatic | SimpleExpressionNode['isStatic'] | - |
loc | SourceLocation | - |
isConstant | boolean | - |
Find the parent element node.
Signature:
declare function findParentNode(
ast: RootNode,
node: Node,
): ElementNode | undefined
Parameter | Type | Description |
---|---|---|
ast | RootNode | - |
node | Node | - |
Find a child (element, component, text, interpolation, or comment) node containing the given position.
Signature:
declare function findTemplateChildNodeAt(
ast: RootNode,
position: number,
mode?: 'start' | 'end',
): SearchResult
Parameter | Type | Description |
---|---|---|
ast | RootNode | - |
position | number | - |
mode | 'start' | 'end' | Open/close range comparison mode: • undefined - position in [start, end] • 'start' — position in [start, end) • 'end' - position in (start, end] |
Get all child (element, component, text, interpolation, or comment) nodes contained in given range. (partial overlaps are ignored)
Signature:
declare function findTemplateChildrenInRange(
ast: RootNode,
start: number,
end: number,
): Node[]
Parameter | Type | Description |
---|---|---|
ast | RootNode | - |
start | number | - |
end | number | - |
Find the deepest node containing the given position.
Signature:
declare function findTemplateNodeAt(
ast: RootNode,
position: number,
): SearchResult
Parameter | Type | Description |
---|---|---|
ast | RootNode | - |
position | number | - |
Find the deepest node containing the given position.
Signature:
declare function findTemplateNodeInRange(
ast: RootNode,
start: number,
end: number,
mode?: 'start' | 'end',
): SearchResult
Parameter | Type | Description |
---|---|---|
ast | RootNode | - |
start | number | - |
end | number | - |
mode | 'start' | 'end' | Open/close range comparison mode: • undefined - position in [start, end] • 'start' — position in [start, end) • 'end' - position in (start, end] |
Get all nodes contained in given range. (partial overlaps are ignored)
Signature:
declare function findTemplateNodesInRange(
ast: RootNode,
start: number,
end: number,
): Node[]
Parameter | Type | Description |
---|---|---|
ast | RootNode | - |
start | number | - |
end | number | - |
Checks if it is an AST AttributeNode.
Signature:
declare function isAttributeNode(node: unknown): node is AttributeNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST CommentNode.
Signature:
declare function isCommentNode(node: unknown): node is CommentNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST ComponentNode.
Signature:
declare function isComponentNode(node: unknown): node is ComponentNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST DirectiveNode.
Signature:
declare function isDirectiveNode(node: unknown): node is DirectiveNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST ElementNode.
Signature:
declare function isElementNode(node: unknown): node is ElementNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST InterpolationNode.
Signature:
declare function isInterpolationNode(node: unknown): node is InterpolationNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is Vue template AST Node.
Signature:
declare function isNode(node: unknown): node is Node
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST PlainElementNode.
Signature:
declare function isPlainElementNode(node: unknown): node is PlainElementNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST RootNode.
Signature:
declare function isRootNode(node: unknown): node is RootNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST ExpressionNode.
Signature:
declare function isSimpleExpressionNode(
node: unknown,
): node is SimpleExpressionNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is a valid JavaScript identifers.
Signature:
declare function isSimpleIdentifier(content: string): boolean
Parameter | Type | Description |
---|---|---|
content | string | - |
Checks if it is an AST TemplateNode.
Signature:
declare function isTemplateNode(node: unknown): node is TemplateNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Checks if it is an AST TextNode.
Signature:
declare function isTextNode(node: unknown): node is TextNode
Parameter | Type | Description |
---|---|---|
node | unknown | - |
Convert template AST to template code.
Signature:
declare function stringify(
node: Node | Node[],
options?: Partial<StringifyOptions>,
): string
Parameter | Type | Description |
---|---|---|
node | Node | Node[] | - |
options | Partial<StringifyOptions> | - |
A general AST traversal utility with both prefix and postfix handlers, and a state object. Exposes ancestry data to each handler so that more complex AST data can be taken into account.
Signature:
declare function traverse<T>(
node: Node,
handlers: TraversalHandler<T> | TraversalHandlers<T>,
state?: T,
): void
Parameter | Type | Description |
---|---|---|
node | Node | - |
handlers | TraversalHandler | TraversalHandlers | - |
state | T | - |
An abortable AST traversal utility. Return false (or falsy value) to stop traversal.
Signature:
declare function traverseEvery<T>(
node: Node,
enter: (node: Node, ancestors: TraversalAncestors, state: T) => boolean,
state?: any,
ancestors?: TraversalAncestors,
): void
Parameter | Type | Description |
---|---|---|
node | Node | - |
enter | (node: Node, ancestors: TraversalAncestors, state: T) => boolean | - |
state | any | - |
ancestors | TraversalAncestors | - |
A faster AST traversal utility. It behaves same as [traverse()] but there is no ancestory data.
Signature:
declare function traverseFast<T = any>(
node: object,
enter: (node: Node, state: T, stop: () => void) => void,
state?: T,
): void
Parameter | Type | Description |
---|---|---|
node | object | - |
enter | (node: Node, state: T, stop: () => void) => void | - |
state | T | - |
interface SearchResult {
ancestors: TraversalAncestors
node: Node | null
}
interface StringifyOptions {
directive: 'shorthand' | 'longhand'
indent: number
initialIndent: number
replaceNodes: Map<Node, Node | null>
}
interface TraversalHandlers<T> {
enter?: TraversalHandler<T>
exit?: TraversalHandler<T>
}
This package is part of VueDX project, maintained by Rahul Kadyan. You can 💖 sponsor him for continued development of this package and other VueDX tools.
FAQs
Helper functions for Vue template AST
The npm package @vuedx/template-ast-types receives a total of 6,903 weekly downloads. As such, @vuedx/template-ast-types popularity was classified as popular.
We found that @vuedx/template-ast-types demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.