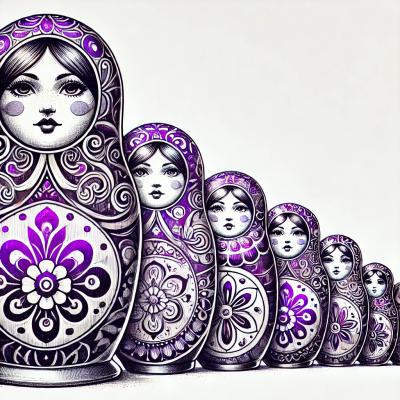
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@vuelidate/core
Advanced tools
@vuelidate/core is a model-based validation library for Vue.js that allows you to define validation rules for your data models. It is designed to be flexible and extensible, making it easy to integrate with your Vue components and manage form validations.
Basic Validation
This code demonstrates how to set up basic validation rules using @vuelidate/core. The `required` and `minLength` validators are imported and applied to the `name` and `password` fields of the form.
```javascript
import { required, minLength } from '@vuelidate/validators';
import useVuelidate from '@vuelidate/core';
export default {
data() {
return {
form: {
name: '',
password: ''
}
};
},
validations() {
return {
form: {
name: { required },
password: { required, minLength: minLength(6) }
}
};
},
setup() {
const v$ = useVuelidate();
return { v$ };
}
};
```
Custom Validators
This code shows how to create a custom validator using @vuelidate/core. The `isEven` validator checks if a number is even and provides a custom error message if it is not.
```javascript
import { helpers } from '@vuelidate/validators';
import useVuelidate from '@vuelidate/core';
const isEven = helpers.withMessage('The value must be even', value => value % 2 === 0);
export default {
data() {
return {
form: {
number: 0
}
};
},
validations() {
return {
form: {
number: { isEven }
}
};
},
setup() {
const v$ = useVuelidate();
return { v$ };
}
};
```
Async Validators
This code demonstrates how to use an asynchronous validator with @vuelidate/core. The `isUniqueUsername` validator checks if a username is unique by making an API call.
```javascript
import { required } from '@vuelidate/validators';
import useVuelidate from '@vuelidate/core';
const isUniqueUsername = async value => {
const response = await fetch(`/api/check-username?username=${value}`);
const data = await response.json();
return data.isUnique;
};
export default {
data() {
return {
form: {
username: ''
}
};
},
validations() {
return {
form: {
username: { required, isUniqueUsername }
}
};
},
setup() {
const v$ = useVuelidate();
return { v$ };
}
};
```
vee-validate is another popular validation library for Vue.js. It provides a declarative way to validate forms and fields, and it integrates well with Vue's template syntax. Compared to @vuelidate/core, vee-validate offers more built-in validation rules and a more declarative approach to form validation.
vuelidate is the predecessor of @vuelidate/core and offers similar functionality. It is also a model-based validation library for Vue.js. The main difference is that @vuelidate/core is a more modern and modular version, providing better performance and flexibility.
vue-formulate is a form and validation library for Vue.js that focuses on simplicity and ease of use. It provides a set of form components with built-in validation rules. Compared to @vuelidate/core, vue-formulate is more opinionated and provides a higher-level abstraction for form handling.
Simple, lightweight model-based validation for Vue.js 2.x & 3.0
Visit Vuelidate Docs for detailed instructions.
You can use Vuelidate just by itself, but we suggest you use it along @vuelidate/validators
, as it gives a nice collection of commonly used
validators.
Vuelidate supports both Vue 3.0 and Vue 2.x
npm install @vuelidate/core @vuelidate/validators
# or
yarn add @vuelidate/core @vuelidate/validators
To use Vuelidate with the Options API, you just need to return an empty Vuelidate instance from setup
.
Your validation state lives in the data
and the rules are in validations
function.
import { email, required } from '@vuelidate/validators'
import { useVuelidate } from '@vuelidate/core'
export default {
name: 'UsersPage',
data: () => ({
form: {
name: '',
email: ''
}
}),
setup: () => ({ v$: useVuelidate() }),
validations () {
return {
form: {
name: { required },
email: { required, email }
}
}
}
}
To use Vuelidate with the Composition API, you need to provide it a state and set of validation rules, for that state.
The state can be a reactive
object or a collection of refs
.
import { reactive } from 'vue' // or '@vue/composition-api' in Vue 2.x
import { useVuelidate } from '@vuelidate/core'
import { email, required } from '@vuelidate/validators'
export default {
setup () {
const state = reactive({
name: '',
emailAddress: ''
})
const rules = {
name: { required },
emailAddress: { required, email }
}
const v$ = useVuelidate(rules, state)
return { state, v$ }
}
}
You can provide global configs to your Vuelidate instance using the third parameter of useVuelidate
or by using the validationsConfig
. These
config options are used to change some core Vuelidate functionality, like $autoDirty
, $lazy
, $scope
and more. Learn all about them
in Validation Configuration.
<script>
import { useVuelidate } from '@vuelidate/core'
export default {
data () {
return { ...state }
},
validations () {
return { ...validations }
},
setup: () => ({ v$: useVuelidate() }),
validationConfig: {
$lazy: true,
}
}
</script>
import { reactive } from 'vue' // or '@vue/composition-api' in Vue 2.x
import { useVuelidate } from '@vuelidate/core'
import { email, required } from '@vuelidate/validators'
export default {
setup () {
const state = reactive({})
const rules = {}
const v$ = useVuelidate(rules, state, { $lazy: true })
return { state, v$ }
}
}
v$
objectinterface ValidationState {
$dirty: false, // validations will only run when $dirty is true
$touch: Function, // call to turn the $dirty state to true
$reset: Function, // call to turn the $dirty state to false
$errors: [], // contains all the current errors { $message, $params, $pending, $invalid }
$error: false, // true if validations have not passed
$invalid: false, // as above for compatibility reasons
// there are some other properties here, read the docs for more info
}
Validation in Vuelidate 2 is by default on, meaning validators are called on initialisation, but an error is considered active, only after a field is dirty, so after $touch()
is called or by using $model
.
If you wish to make a validation lazy, meaning it only runs validations once it a field is dirty, you can pass a { $lazy: true }
property to
Vuelidate. This saves extra invocations for async validators as well as makes the initial validation setup a bit more performant.
const v = useVuelidate(rules, state, { $lazy: true })
If you wish to reset a form's $dirty
state, you can do so by using the appropriately named $reset
method. For example when closing a create/edit
modal, you dont want the validation state to persist.
<app-modal @closed="v$.$reset()">
<!-- some inputs -->
</app-modal>
The validation state holds useful data, like the invalid state of each property validator, along with extra properties, like an error message or extra parameters.
Error messages come out of the box with the bundled validators in @vuelidate/validators
package. You can check how change those them over at
the Custom Validators page
The easiest way to display errors is to use the form's top level $errors
property. It is an array of validation objects, that you can iterate over.
<p
v-for="(error, index) of $v.$errors"
:key="index"
>
<strong>{{ error.$validator }}</strong>
<small> on property</small>
<strong>{{ error.$property }}</strong>
<small> says:</small>
<strong>{{ error.$message }}</strong>
</p>
You can also check for errors on each form property:
<p
v-for="(error, index) of $v.name.$errors"
:key="index"
>
<!-- Same as above -->
</p>
For more info, visit the Vuelidate Docs.
To test the package run
# install dependencies
yarn install
# create bundles.
yarn build
# Create docs inside /docs package
yarn dev
# run unit tests for entire monorepo
yarn test:unit
# You can also run for same command per package
FAQs
Simple, lightweight model-based validation for Vue.js
We found that @vuelidate/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.