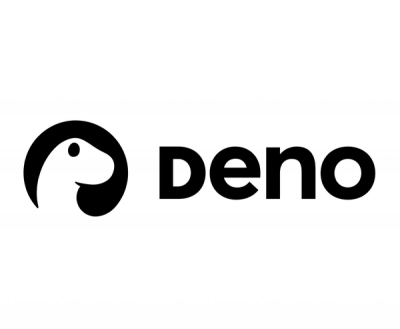
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@walletconnect/sign-client
Advanced tools
@walletconnect/sign-client is a JavaScript client library for WalletConnect, a protocol that enables secure connections between decentralized applications (dApps) and wallets. This package allows developers to integrate WalletConnect functionality into their applications, enabling features such as session management, message signing, and transaction handling.
Initialize Client
This code demonstrates how to initialize the WalletConnect SignClient with a project ID and relay URL. This is the first step to start using the WalletConnect functionalities.
const { SignClient } = require('@walletconnect/sign-client');
async function initClient() {
const client = await SignClient.init({
projectId: 'your_project_id',
relayUrl: 'wss://relay.walletconnect.org'
});
console.log('Client initialized:', client);
}
initClient();
Create Session
This code demonstrates how to create a new session with the WalletConnect client. It specifies the required namespaces, methods, chains, and events, and then waits for the session to be approved.
async function createSession(client) {
const { uri, approval } = await client.connect({
requiredNamespaces: {
eip155: {
methods: ['eth_sendTransaction', 'personal_sign'],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
}
}
});
console.log('Session URI:', uri);
const session = await approval();
console.log('Session approved:', session);
}
createSession(client);
Sign Message
This code demonstrates how to sign a message using the WalletConnect client. It sends a request to sign a message and logs the signed message.
async function signMessage(client, session) {
const message = 'Hello, WalletConnect!';
const result = await client.request({
topic: session.topic,
chainId: 'eip155:1',
request: {
method: 'personal_sign',
params: [message, session.accounts[0]]
}
});
console.log('Signed message:', result);
}
signMessage(client, session);
Send Transaction
This code demonstrates how to send a transaction using the WalletConnect client. It constructs a transaction object and sends it, then logs the result.
async function sendTransaction(client, session) {
const tx = {
from: session.accounts[0],
to: '0xRecipientAddress',
data: '0x',
value: '0x0',
gas: '0x5208'
};
const result = await client.request({
topic: session.topic,
chainId: 'eip155:1',
request: {
method: 'eth_sendTransaction',
params: [tx]
}
});
console.log('Transaction result:', result);
}
sendTransaction(client, session);
Web3.js is a collection of libraries that allow you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket. It provides functionalities for sending transactions, interacting with smart contracts, and more. Compared to @walletconnect/sign-client, Web3.js is more focused on direct interaction with Ethereum nodes and smart contracts, while @walletconnect/sign-client is focused on connecting dApps with wallets via the WalletConnect protocol.
Ethers.js is a library for interacting with the Ethereum blockchain and its ecosystem. It provides a complete and compact library for sending transactions, interacting with smart contracts, and more. Similar to Web3.js, Ethers.js is more focused on direct blockchain interactions, whereas @walletconnect/sign-client is specialized in facilitating connections between dApps and wallets using WalletConnect.
Web3Modal is a library that allows developers to easily integrate multiple wallet providers into their dApps. It supports WalletConnect, MetaMask, and other popular wallets. While Web3Modal provides a broader range of wallet integrations, @walletconnect/sign-client is specifically designed for WalletConnect protocol, offering more specialized features for WalletConnect sessions and interactions.
Sign Client for WalletConnect Protocol
This library provides a Sign Client for WalletConnect v2.0 Protocol for both Dapps and Wallets. Integration will differ from the perspective of each client as the Proposer and Responder, respectively. It's compatible with NodeJS, Browser and React-Native applications (NodeJS modules required to be polyfilled for React-Native)
Check out documentation here.
Also available quick start for Dapps and for Wallets
Apache 2.0
FAQs
Sign Client for WalletConnect Protocol
The npm package @walletconnect/sign-client receives a total of 503,150 weekly downloads. As such, @walletconnect/sign-client popularity was classified as popular.
We found that @walletconnect/sign-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.