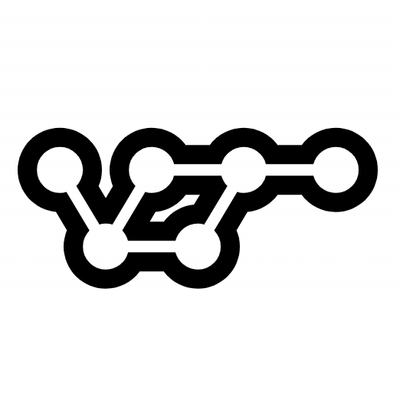
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@weareglow/story-unit
Advanced tools
An Instagram Story inspired component.
Add your markup. Start by creating a single element which will contain each chapter of your story. A chapter can be a video
element or an image
element. Make to include the appropriate video attribtues on your video elements. When using an image element, specify how long the image should be displayed with the data-duration attribute on your img element. Defaults to 1 second. See Below.
<div id="chapters">
<video muted playsinline src="video.mp4"></video>
<img data-duration="2" src="image.jpg" alt="" />
<video muted playsinline src="video.mp4"></video>
<video muted playsinline src="video.mp4" type="video/mp4"></video>
</div>
Now initialize your story by providing it with an options object.
var story = Story({
el: '#chapters',
animate: false // defaults to true. set to false if you want to handle chapter animations yourself.
})
Your story will now have a few methods you can call to get things rolling.
Method | Description |
---|---|
play | begins the story |
end | jumps to end of story |
replay | starts story from beginning |
Now you probably want to know when the story's state changes, right? Get notified of story updates by subscribing to the following events:
Event | Description | Payload |
---|---|---|
start | fires when the story begins playing. | {current, previous} |
update | fires when the story's active chapter changes. | {current, previous} |
last-chapter | fires at the start of the last chapter | {current, previous} |
end | fires when the entire story has completed. | {current, previous} |
skip-to-end | fires when the user skips to the end of the story | {current, previous} |
Each emitted event will send a payload that includes an object with current and previous properties. The current and previous properties each respectively have a ref property which is the DOM element of that chapter. This is useful if you want to handle animations on your own.
story.on('start', function(payload) {
// payload.current.ref
// payload.previous.ref
}
story.on('update', function(payload) {
// payload.current.ref
// payload.previous.ref
}
story.on('last-chapter', function(payload) {
// payload.current.ref
// payload.previous.ref
}
story.on('end', function(payload) {
// payload.current.ref
// payload.previous.ref
}
story.on('skip-to-end', function(payload) {
// payload.current.ref
// payload.previous.ref
}
So you've subscribed to some events...nice! How about allowing a user to initiate some actions themselves like, replaying the story, or skipping to the end? Easy!
var replayBtn = document.getElementById('replay-btn')
var skipBtn = document.getElementById('skip-btn')
replayBtn.addEventListener('click', story.replay.bind(story)) // notice we're binding our this context to the story, important!
skipBtn.addEventListener('click', story.end.bind(story))
You'll notice we have to bind our DOM event listeners this context to the story like story.replay.bind(this)
. This is important, as if you don't, the story won't have access to it's methods and properties.
story.play()
FAQs
Unknown package
The npm package @weareglow/story-unit receives a total of 2 weekly downloads. As such, @weareglow/story-unit popularity was classified as not popular.
We found that @weareglow/story-unit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.