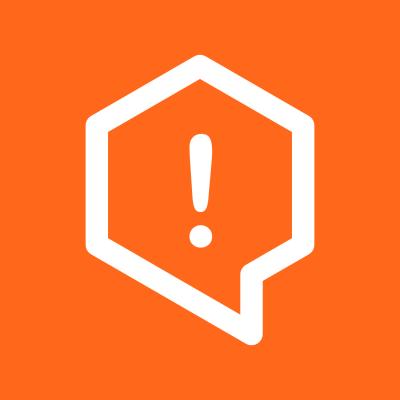
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@wework/floormap.gl
Advanced tools
[](https://circleci.com/gh/WeConnect/floormap.gl/tree/develop) [,
size: {
width: 500,
height: 500
},
backgroundColor: 'grey',
antialias: true,
pixelRatio: 2
})
// draw a simple mesh
renderer.draw({
id: '001',
tags: ['hello'],
type: 'MESH',
style: {
color: 'rgb(155, 255, 55)',
side: 'FRONT',
outline: {
color: 'rgb(255, 0, 0)',
only: false
}
},
points: [
{ x: -1, y: -1 },
{ x: 1, y: -1 },
{ x: 1, y: 1 },
{ x: -1, y: 1 }
],
interactable: true,
extrude: 0,
position: { x: 0, y: 0, z: 0 },
rotation: { x: 0, y: 0, z: 0 },
scale: { x: 10, y: 10, z: 10 }
},
false,
(success) => {
console.log(success)
},
(error) => {
console.log(e)
})
</script>
</body>
</html>
link the floormap.gl package in your node application
yarn link '@wework/floormap.gl'
or download the package directly from the npm
yarn add '@wework/floormap.gl'
Example usage in a react application
import React, { Component } from 'react'
import FloorMapGL from '@wework/floormap.gl'
class FloorMap extends Component {
componentDidMount() {
this.renderer = new FloorMapGL({
target: this.mount,
size: {
width: 500,
height: 500
},
backgroundColor: 'grey',
antialias: true,
pixelRatio: 2
})
this.renderer.draw(
{
id: '001',
tags: ['hello'],
type: 'MESH',
style: {
color: 'rgb(155, 255, 55)',
side: 'FRONT',
outline: {
color: 'rgb(255, 0, 0)',
only: false
}
},
points: [
{ x: -1, y: -1 },
{ x: 1, y: -1 },
{ x: 1, y: 1 },
{ x: -1, y: 1 }
],
interactable: true,
extrude: 0,
position: { x: 0, y: 0, z: 0 },
rotation: { x: 0, y: 0, z: 0 },
scale: { x: 10, y: 10, z: 10 }
},
false,
(success) => {
console.log(success)
},
(error) => {
console.log(e)
}
)
}
render() {
return (
<div
ref={(mount) => {
this.mount = mount
}}
/>
)
}
}
export default FloorMap
We have created sample applications that make use of the floormap.gl library, you may run it by entering the command below
yarn start:demo
import FloorMapGL from '@wework/floormap.gl'
var renderer = new FloorMapGL({
target: domElement,
size: {
width: 720,
height: 480
},
backgroundColor: 'black',
antialias: true,
pixelRatio: 2,
camera: {
maxPolarAngle: 90,
enableRotate: true,
zoomLevel: 1
},
groundPlane: true
})
Name | Type | Description |
---|---|---|
target | object | domElement, it is required |
size | sizeObject | window size of the renderer |
backgroundColor | string | background color of the renderer, default is 'black' |
antialias | boolean | Options to smooth the jagged line, default is true |
pixelRatio | number | The rendering resolution, default is 2 |
camera | cameraObject | camera settings |
groundPlane | boolean | invisible ground plane for the mouse interaction, default is false |
Name | Type | Description |
---|---|---|
width | number | the width of the renderer window, by default is 700 |
height | number | the height of the renderer window, by default is 400 |
Name | Type | Description |
---|---|---|
zoomLevel | number | zoom level of the camera view, default is 1 |
enableRotate | boolean | to enable to control and rotate the camera view angle, default is true |
maxPolarAngle | number | max horizontal rotation of the camera in degree, default is 90 . If you set it to 0 it will disable the camera to rotate in 3D view |
Draw the list of renderObjects onto the screen
.draw(renderObjects: array, transparentUpdate: boolean, onSuccess: function, onError: function)
Parameters | Type | Description |
---|---|---|
arrayOfParams | array | array of RenderObjects |
transparentUpdate | boolean | flag to enable clearing and re-render the scene again for transparent object |
onSuccess | function | callback function when successfully render this draw call |
onError | function | callback function when there is an error occur in this draw call |
// create your renderObjects and push into your array first
const renderObjects = []
renderObjects.push({
id: '001',
tags: '',
type: 'MESH',
style: {
color: 'rgb(155, 255, 55)',
side: 'FRONT',
outline: {
color: 'rgb(255, 0, 0)',
only: false
}
},
points: [{ x: -1, y: -1 }, { x: 1, y: -1 }, { x: 1, y: 1 }, { x: -1, y: 1 }],
interactable: true,
visible: true,
extrude: 0,
position: { x: 0, y: 0, z: 0 },
rotation: { x: 0, y: 0, z: 0 },
scale: { x: 1, y: 1, z: 1 },
draw: true
})
// draw all the renderObjects using .draw
renderer.draw(
renderObjects,
false,
(success) => {
console.log(success)
},
(errors) => {
console.log(errors)
}
)
// to update the renderObjects in the future
// using the same .draw() api to update the specific renderObject by id
renderer.draw(
[
{
id: '001',
style: {
color: 'rgb(155, 255, 55)'
},
position: { x: 10, y: 0, z: 0 }
}
],
false,
(success) => {
console.log(success)
},
(errors) => {
console.log(errors)
}
)
Update the size of the renderer window
.updateWindowDimension({ width: Number, height: Number })
Parameters | Type | Description |
---|---|---|
width | number | new width for the renderer window |
height | number | new height for the renderer window |
renderer.updateWindowDimension({ width, height })
Set the camera panning position
.setCameraViewPoint({ position: { x: Number, y: Number, z: Number } })
renderer.setCameraViewPoint({
position: { x: 2, y: 0, z: 5 }
})
Set the camera rotation view angle
.setCameraViewAngle({ polarAngle: Number, azimuthAngle: Number })
Parameters | Type | Description |
---|---|---|
polarAngle | number | rotation angle in 3d view, default is 0 degree |
azimuthAngle | number | rotation angle in 2d view, default is 0 degree |
renderer.setCameraViewAngle({ polarAngle: 45, azimuthAngle: -45 })
Set the maximum camera rotation view angle
.setMaxCameraViewAngle({ maxPolarAngle: Number, maxAzimuthAngle: Number })
Parameters | Type | Description |
---|---|---|
maxPolarAngle | number | how far you can rotate in 3d view, default is 90 degree |
maxAzimuthAngle | number | how far you can rotate in 2d view, default is 360 degree |
renderer.setMaxCameraViewAngle({ maxPolarAngle: 90, maxAzimuthAngle: 360 })
renderer.setMaxCameraViewAngle({ maxPolarAngle: 0 }) // Here is one way to disable 3D view
Get all the renderObjects id by tags
.getIdsByTags(tags: Array)
renderer.getIdsByTags(['tag1', 'tag2'])
Adjust the renderer camera view frustum to fit the content space / given drawn boundaries
.fitContent(params: Object)
Parameters | Type | Description |
---|---|---|
id | string | renderObject id, default is the whole renderScene boundary |
padding | number | add the padding for the camera view, default is 0 |
points | array | custom boundary shape, optional |
this.renderer.fitContent()
this.renderer.fitContent({ padding: 50 })
this.renderer.fitContent({ id: '07-124', padding: 50 })
this.renderer.fitContent({ points: points, padding: 50 })
.createInstancedMesh(renderObject: object)
renderer.createInstancedMesh({
id: 'MESH_GROUP_1',
mesh: {
style: {
color: 'rgb(155, 255, 55)',
side: 'FRONT',
outline: {
color: 'rgb(255, 0, 0)',
width: 0.2,
only: false
}
},
points: [...],
extrude: 5
},
})
.addToInstancedMesh(params: object)
renderer.addToInstancedMesh({
id: 'UUID',
instancingId: 'MESH_GROUP_1',
style: {
color: 'rgb(100,101,102)'
},
position: { x: 0, y: 0, z: 0 },
rotation: { z: 0 },
scale: { x: 1, y: 1, z: 1 }
})
.drawInstancedMesh(params: object)
renderer.drawInstancedMesh({ id: 'MESH_GROUP_1' })
.removeInstancedMesh(id: string)
renderer.removeInstancedMesh('MESH_GROUP_1')
subscribe to a renderer event
.addEventListener(eventName: String, callback: Function)
renderer.addEventListener(renderer.Events.ONMOUSEOVER, mouseEnterCallback)
renderer.addEventListener(renderer.Events.ONMOUSEOUT, mouseLeaveCallback)
renderer.addEventListener(renderer.Events.ONCLICK, onClickCallback)
unsubscribe to a renderer event
.removeEventListener(eventName: String, callback: Function)
renderer.removeEventListener(renderer.Events.ONMOUSEOVER, mouseEnterCallback)
renderer.removeEventListener(renderer.Events.ONMOUSEOUT, mouseLeaveCallback)
renderer.removeEventListener(renderer.Events.ONCLICK, onClickCallback)
unsubscribe all the renderer event listeners
.removeAllEventListeners()
remove specific renderObject by id
.remove(id: String)
renderer.remove(id)
remove all the renderObjects in the renderer
.removeAll()
renderer.removeAll()
Name | Value | Description |
---|---|---|
ONCLICK | 'onclick' | when the user select the interactable renderObject by clicking/touching |
ONHOVER | 'onmousehover' | when the user mouse hovering the interactable renderObject |
ONMOUSEOVER | 'onmouseover' | when moving the mouse pointer onto a renderObject |
ONMOUSEOUT | 'onmouseout' | when moving the mouse pointer out of a renderObject |
ONMOUSEMOVE | 'onmousemove' | when moving the mouse pointer |
ONRENDER | 'onrender' | when .draw api successfully sent all the data to the gpu to render |
ONERROR | 'onerror' | when floormap.gl renderer encounters any internal errors |
ONTEXTURELOAD | 'ontextureload' | when texture is loaded and sent to the gpu |
Payload | Type | Description |
---|---|---|
id | string | id of the renderObject |
point | object | intersection point in the world space, x y z value |
mousePos | object | the mouse position in screen space, x y value |
Payload | Type | Description |
---|---|---|
id | string | id of the renderObject |
point | object | intersection point in the world space, x y z value |
mousePos | object | the mouse position in screen space, x y value |
Payload | Type | Description |
---|---|---|
id | string | id of the renderObject |
point | object | intersection point in the world space, x y z value |
mousePos | object | the mouse position in screen space, x y value |
Payload | Type | Description |
---|---|---|
id | string | id of the renderObject |
point | object | intersection point in the world space, x y z value |
mousePos | object | the mouse position in screen space, x y value |
Payload | Type | Description |
---|---|---|
id | string | id of the renderObject |
point | object | intersection point in the world space, x y z value |
mousePos | object | the mouse position in screen space, x y value |
Payload | Type | Description |
---|---|---|
frameTime | number | the time taken to complete this draw call |
memory | object | gpu memory info |
render | object | render info |
renderObjects | array | list of renderObjects to render in the draw call |
Payload | Type | Description |
---|---|---|
error | object | Error() object |
Payload | Type | Description |
---|---|---|
frameTime | number | the time taken to complete this draw call |
memory | object | gpu memory info |
render | object | render info |
renderObjects | array | list of renderObjects to render in the draw call |
Parameters | Type | Description |
---|---|---|
id | string | id of the renderObject required |
tags | array | renderObject tags |
type | string | renderObject type, 'MESH', 'SPRITE', 'TEXT' or 'LINE' required |
style | meshStyleObject | style of the renderObject |
points | array | points to construct a shape required |
geometryTranslate | transformObject | translation for the geometry in object space |
interactable | boolean | interaction of this object disable or enable |
visible | boolean | visibility flag |
extrude | number | extrude level for the mesh |
position | transformObject | position of the renderObject |
rotation | transformObject | rotation of the renderObject |
scale | transformObject | scale of the renderObject |
{
id: '001', // required
tags: ['level 3'],
type: 'MESH',
style: {
color: 'rgb(155, 255, 55)',
side: 'FRONT',
texture: {
img: '',
repeat: 0.5
}
outline: {
color: 'rgb(255, 0, 0)',
width: 0.2,
only: false
}
},
points: [
{ x: -1, y: -1 },
{ x: 1, y: -1 },
{ x: 1, y: 1 },
{ x: -1, y: 1 }
],
geometryTranslate: {x: -1, y: -1, z: 0},
interactable: true,
visible: true,
extrude: 2,
position: { x: 0, y: 0, z: 0 },
rotation: { x: 0, y: 0, z: 0 },
scale: { x: 1, y: 1, z: 1 }
}
Parameters | Type | Description |
---|---|---|
id | string | id of the renderObject required |
tags | array | renderObject tags |
type | string | renderObject type, 'MESH', 'SPRITE', 'TEXT' or 'LINE' required |
style | textStyleObject | style of the renderObject |
text | string | text to draw on the screen required |
interactable | boolean | interaction of this object disable or enable |
visible | boolean | visibility flag |
position | transformObject | position of the renderObject |
scalar | number | text size |
{
id: '002',
tags: ['nothing'],
type: 'TEXT',
position: { x: 0, y: 0, z: 0 },
scalar: 1.0,
center: { x: 0.5, y: 0.5 },
style: {
color: 'rgb(255, 255, 255)',
fontFamily: 'Arial, Helvetica, sans-serif',
textAlign: 'center',
fontWeight: 'normal', // normal, bold, bolder, lighter
fontStyle: 'italic' // normal, italic, oblique
},
text: 'hello world',
interactable: false,
visible: true
}
Parameters | Type | Description |
---|---|---|
id | string | id of the renderObject required |
tags | array | renderObject tags |
type | string | renderObject type, 'MESH', 'SPRITE', 'TEXT' or 'LINE' required |
position | transformObject | position of the renderObject |
style | textStyleObject | style of the renderObject |
interactable | boolean | interaction of this object disable or enable |
visible | boolean | visibility flag |
{
id: '003',
tags: ['level 3'],
type: 'SPRITE',
position: { x: 2, y: 0 },
center: { x: 0.5, y: 0.5 },
style: {
color: 'rgb(255, 255, 255)',
img: 'https://cdn.spacemob.co/img/meeting_room@2x.png',
width: 'auto',
height: 'auto',
maxWidth: 3,
maxHeight: 3
},
interactable: false,
visible: true
}
Parameters | Type | Description |
---|---|---|
id | string | id of the renderObject required |
tags | array | renderObject tags |
type | string | renderObject type, 'MESH', 'SPRITE', 'TEXT' or 'LINE' required |
lineWidth | number | the width of the line |
points | array | points for constructing the line required |
position | transformObject | position of the renderObject |
style | lineStyleObject | style of the renderObject |
interactable | boolean | interaction of this object disable or enable |
visible | boolean | visibility flag |
{
id: '004',
tags: ['level 3'],
type: 'LINE',
lineWidth: 1.0,
points: [
{ x: 0, y: 0 },
{ x: 100, y: 0 },
{ x: 100, y: 90 },
{ x: 200, y: 20 }
],
style: {
color: 'rgb(255, 0, 255)',
opacity: 0.7
},
interactable: false,
visible: true
}
Parameters | Type | Description |
---|---|---|
x | number | set value in x-axis |
y | number | set value in y-axis |
z | number | set value in z-axis |
Parameters | Type | Description |
---|---|---|
color | string | color of the object |
side | string | 'FRONT', 'BACK' or 'DOUBLE', default is 'FRONT' |
texture | number | texture setting of the renderObject |
texture.img | string | img path or url |
texture.repeat | number | how many times to repeat the image on the object |
outline | number | outline setting of the renderObject |
outline.color | number | outline color of the object |
outline.only | number | if true then will be outline only object |
Parameters | Type | Description |
---|---|---|
color | string | color of the text |
fontFamily | string | default is 'Arial, Helvetica, sans-serif' |
textAlign | number | text alignment, 'center', 'left' or 'right', default is 'center' |
fontWeight | string | 'normal', 'bold', 'bolder' or 'lighter', default is 'normal' |
fontStyle | number | 'normal', 'italic' or 'oblique', default is 'normal' |
Parameters | Type | Description |
---|---|---|
color | string | color of the sprite |
img | string | image path or url |
width | number or string | width of the sprite, default is 'auto' |
height | number or string | height of the sprite, default is 'auto' |
maxWidth | number | maximum width of the sprite |
maxHeight | number | maximum height of the sprite |
Parameters | Type | Description |
---|---|---|
color | string | color of the line |
opacity | number | transparency of the line |
FAQs
[](https://circleci.com/gh/WeConnect/floormap.gl/tree/develop) [![Coverage Status](https://coveralls.io/repos/github
We found that @wework/floormap.gl demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 39 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.