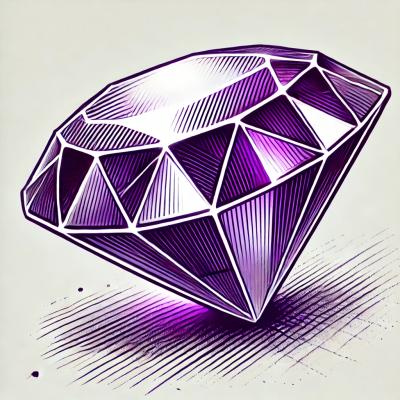
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@wordpress/deprecated
Advanced tools
Deprecation utility for WordPress. Logs a message to notify developers about a deprecated feature.
Install the module
npm install @wordpress/deprecated --save
This package assumes that your code will run in an ES2015+ environment. If you're using an environment that has limited or no support for ES2015+ such as lower versions of IE then using core-js or @babel/polyfill will add support for these methods. Learn more about it in Babel docs.
The deprecated
action is fired with three parameters: the name of the deprecated feature, the options object passed to deprecated, and the message sent to the console.
Example:
import { addAction } from '@wordpress/hooks';
function addDeprecationAlert( message, { version } ) {
alert( `Deprecation: ${ message }. Version: ${ version }` );
}
addAction( 'deprecated', 'my-plugin/add-deprecation-alert', addDeprecationAlert );
Logs a message to notify developers about a deprecated feature.
Usage
import deprecated from '@wordpress/deprecated';
deprecated( 'Eating meat', {
version: 'the future',
alternative: 'vegetables',
plugin: 'the earth',
hint: 'You may find it beneficial to transition gradually.',
} );
// Logs: 'Eating meat is deprecated and will be removed from the earth in the future. Please use vegetables instead. Note: You may find it beneficial to transition gradually.'
Parameters
string
: Name of the deprecated feature.?Object
: Personalisation options?string
: Version in which the feature will be removed.?string
: Feature to use instead?string
: Plugin name if it's a plugin feature?string
: Link to documentation?string
: Additional message to help transition away from the deprecated feature.Object map tracking messages which have been logged, for use in ensuring a message is only logged once.
Type
Object
FAQs
Deprecation utility for WordPress.
The npm package @wordpress/deprecated receives a total of 82,244 weekly downloads. As such, @wordpress/deprecated popularity was classified as popular.
We found that @wordpress/deprecated demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 23 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.