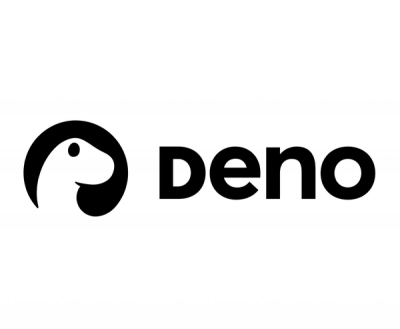
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@workerbase/sdk
Advanced tools
Install with npm:
npm install @workerbase/sdk
You can use the package like this:
import workerbase, { FLEX_DIRECTION } from '@workerbase/sdk';
Workerbase SDK fully supports typescript with .d.ts
files and the types will be maintained in the next releases.
import workerbase from '@workerbase/sdk'
// Doing simple requests
const updateUserName = async (userId) => {
const { firstName } = await workerbase.users.get(userId);
await workerbase.users.update(userId, { firstName: firstName + "v2" });
};
// Working with database items
const createDbAndInsertItems = async () => {
const database = await workerbase.databases.create({
name: "test",
columns: [
{ name: "field1", type: "String" },
{ name: "value", type: "Integer" },
],
});
await database.items.create({ field1: "abc" }, "item1");
await database.items.update("item1", { payload: { value: "5" } });
await database.items.create({ field1: "abcd" }, "item2");
await database.items.delete("item2");
await database.items.query({$or: [{field1: "abc"}, {value: {$gt: 4}}]}, {populate: true});
};
};
// Using list response builtin methods
const getAllUserDepartments = () =>
workerbase.users.list().distinctBy("department");
const getAllUsersWithName = (userLastname) =>
workerbase.users.list().filterBy({ lastName: userLastname });
const getAllUserDepartmentsWithName = (userLastName) =>
workerbase.users
.list()
.filterBy({ lastName: userLastName })
.distinctBy("department");
// Invoking a workerbase Function
const invokeFunction = async (functionId) => {
try {
return await workerbase.functions.invoke(functionId, { some: "data" });
} catch (err) {
console.log(err.logs);
}
};
import workerbase from '@workerbase/sdk'
workerbase.databases.list(\[listConfig\])
workerbase.databases.get(id)
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
Database.items.list(\[listConfig\])
Database.items.get(id)
Database.items.create(value\[,externalId\])
Database.items.createMany(values)
Database.items.update(id,value)
Database.items.delete(id)
Database.items.deleteMany(query)
Database.items.query(query, options)
More information about Database.items.query
can be found here.
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
workerbase.connectors.list(\[listConfig\])
workerbase.connectors.get(id)
workerbase.connectors.create(value)
workerbase.connectors.update(id,value)
workerbase.connectors.delete(id)
workerbase.connectors.trigger(id,payload)
More information about List Configs
can be found here.
Deprecated usage:
workerbase.events.list(\[listConfig\])
workerbase.events.get(id)
workerbase.events.create(value)
workerbase.events.update(id,value)
workerbase.events.delete(id)
workerbase.events.trigger(id,payload)
import workerbase from '@workerbase/sdk'
workerbase.functions.list(\[listConfig\])
workerbase.functions.get(id)
workerbase.functions.deploy(id)
workerbase.functions.invoke(id,payload)
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
workerbase.locations.list(\[listConfig\])
workerbase.locations.get(id)
workerbase.locations.create(value)
workerbase.locations.update(id,value)
workerbase.locations.delete(id)
workerbase.locations.getPartsByLevel(levelId)
workerbase.locations.getLevels()
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
workerbase.media.list(\[listConfig\])
workerbase.media.get(id)
workerbase.media.delete(id)
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
workerbase.roles.list(\[listConfig\])
workerbase.roles.get(id)
workerbase.roles.create(value)
workerbase.roles.update(id,value)
workerbase.roles.delete(id)
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
workerbase.skills.list(\[listConfig\])
workerbase.skills.get(id)
workerbase.skills.create(value)
workerbase.skills.update(id,value)
workerbase.skills.delete(id)
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
workerbase.users.list(\[listConfig\])
workerbase.users.get(id)
workerbase.users.create(value)
workerbase.users.update(id,value)
workerbase.users.delete(id)
More information about List Configs
can be found here.
import workerbase from '@workerbase/sdk'
workerbase.tasks.list(\[listConfig\])
workerbase.tasks.get(taskId)
workerbase.tasks.delete(taskId)
workerbase.tasks.update(taskId,value)
workerbase.tasks.assignTaskToUserId(userId, taskId)
// variables is an object: { var1: 'value1', var2: 'value2' }
workerbase.tasks.updateVariables(taskId,variables)
workerbase.tasks.getTasksByProjectId(projectId)
More information about List Configs
can be found here.
interface ListConfig {
// Starts at 1
page?: number;
// Default is 1000
perpage?: number;
// field to sort on (not supported by every field)
sort?: string;
order?: 'desc' | 'asc';
// fields to select
fields?: string;
}
We added some builtin methods in Workerbase SDK to help you work with the data on the list responses. See the examples above, to see how to use them.
list().filterBy(condition)
Filter the list to keep only the values that match the condition object. Example:
const filteredList = await workerbase.users
.list({
page: 1,
perpage: 10,
sort: 'firstName',
order: 'desc',
fields: 'firstName lastName',
})
.filterBy({ firstName: 'Anna' });
list().distinctBy(field)
Return the list of all distinct values of the specified field. Example:
const getAllUserDepartments = await workerbase.users.list().distinctBy('department');
The Database.items.query()
function allows complex queries to Workerbase databases.
The following example explains a simple first query:
The example is done with the database Employee
.
Employee
database
ID | Name | Birthday | Department | Office | |
---|---|---|---|---|---|
String | String | String | Date | Reference [Department] | Location |
1 | Vannie Doulden | vannie@company.com | 12.11.1988 | 1 | North America / New York |
2 | Marijo Stede | marijo@company.com | 03.06.1994 | 2 | Europe / Munich |
3 | Jade Plante | jade@company.com | 27.02.1990 | 2 | Europe / Munich |
All employees where the birthday is after the 01.01.1989
and the name ends on Stede
should be queried.
const EmployeeDB = async workerbase.databases.get("Employee");
const result = await EmployeeDB.items.query({
Birthday: {
$gt: "01.01.1989"
},
Name: {
$like: "%Stede"
}
})
The result
variable will contain the employee Marijo Stede
.
[
{
"_id": "634fc1e9d64ce49dd554c2db",
"meta": {
"createdAt": "2022-10-19T09:22:49.782Z",
"updatedAt": "2022-10-19T09:27:50.733Z"
},
"database": "634fbc5cd64ce49dd554bdb0",
"payload": {
"Name": "Marijo Stede",
"Email": "marijo@company.com",
"Birthday": "1994-06-03T00:00:00.000Z",
"Department": "634fbc8dd64ce49dd554bde6",
"Office": "634fc1825025ac5347b21ffc"
},
"deleted": false,
"externalId": "2"
}
]
Advanced features of the Database.items.query()
function are described in the sections Query Operators, Pagination, Sorting, Population, Nested Property Queries
Before looking into advanced features we recomment to review the Typings Definition of the query function.
type QueryFunction = (query: Query, options: ListOptions): Promise<DatabaseItem[]>
type Query = Record<QueryOperator, string | number | boolean | Query>;
enum QueryOperator {
EQ = '$eq',
NE = '$ne',
GT = '$gt',
GTE = '$gte',
LT = '$lt',
LTE = '$lte',
IN = '$in',
NIN = '$nin',
EXISTS = '$exists',
AND = '$and',
OR = '$or',
LIKE = '$like',
NOT_LIKE = '$notLike',
}
interface ListOptions {
page?: number; // Starts at 1
perpage?: number;
sort?: string;
order?: 'desc' | 'asc';
populate?: boolean;
}
interface DatabaseItem {
_id: string;
externalId?: string;
payload: ItemPayload;
}
type ItemPayload = Record<string, any>;
The following query operators are supported.
enum QueryOperator {
// "equals"
EQ = '$eq',
// "not equals"
NE = '$ne',
// "greater than"
GT = '$gt',
// "greater than or equals"
GTE = '$gte',
// "less than"
LT = '$lt',
// "less than or equals"
LTE = '$lte',
// "in"
// Expects a value to be in an array of values.
// Example: Get all employees whose name is in an array with values
// "Vannie Doulden" and "Jade Plante".
// Query: { Name: { $in: [ "Vannie Doulden", "Jade Plante" ] } }
IN = '$in',
// "not in"
// Expects a value not in an array of values.
NIN = '$nin',
// "exists"
// Checks whether a value is defined.
// Example: Get all employees where Department is not defined.
// Query: { Department: { $exists: false } }
EXISTS = '$exists',
// "and"
// Expects multiple conditions to be true.
// Example: Get all employees whose name is
// "Jade Plante" and where Department is "Product Management".
// Query:
// { $and: [
// { Name: "Jade Plante" },
// { Department: "Product Management" }
// ]}
AND = '$and',
// "or"
// Expects one of the conditions to be true
// Example: Get all employees where Department is
// either "Engineering" or "Product Management".
// Query
// { $or: [
// { Department: "Engineering" },
// { Department: "Product Management" }
// ]}
OR = '$or',
// "like"
// Expects a column of type string to match a pattern.
// Patterns can include the wildcards "%" and "_":
// => "%" stands for zero or more arbitrary characters.
// => "_" stands for one arbitrary characters.
// Example: Get all employees where the Name starts with "Jade".
// Query: { Name: { $like: "Jade%" } }
LIKE = '$like',
// "not like"
// Expects a column of type string not to match a pattern.
NOT_LIKE = '$notLike',
}
The result of the query function can be paginated with the page
and perpage
properties of the options
parameter.
type QueryFunction = (query: Query, options: ListOptions): Promise<DatabaseItem[]>
interface ListOptions {
page?: number; // Starts at 1
perpage?: number;
sort?: string;
order?: 'desc' | 'asc';
populate?: boolean;
}
Example: If the database Employee
contains 100
items, the last 10 items (91-100) can be requested with the following query.
const EmployeeDB = async workerbase.databases.get("Employee");
const result = await EmployeeDB.items.query({}, { page: 10, perpage: 10 });
Items in the result can be sorted with the sort
and order
properties of the options
parameter.
Example: In order to sort items from the Employee
database by their age starting with the oldest one, the following query has to be run.
const EmployeeDB = async workerbase.databases.get("Employee");
const result = await EmployeeDB.items.query({}, { sort: 'Birthday', order: 'desc' });
Databases Items in Workerbase can have references to other Database Items and to User
, Role
and Location
items in the platform. Those references can be directly included into the result by adding { populate: true }
to the options parameter.
Example: The Department column of the Employee
database is of type Reference
and points to the database Department
. Furthermore the Employee
database has a column Office
which is of type Location
and points to locations in the Workerbase platform.
Employee
database
ID | Name | Birthday | Department | Office | |
---|---|---|---|---|---|
String | String | String | Date | Reference [Department] | Location |
1 | Vannie Doulden | vannie@company.com | 12.11.1988 | 1 | North America / New York |
2 | Marijo Stede | marijo@company.com | 03.06.1994 | 2 | Europe / Munich |
3 | Jade Plante | jade@company.com | 27.02.1990 | 2 | Europe / Munich |
Department
database
ID | Name | Headcount |
---|---|---|
String | String | Number |
1 | Product Management | 10 |
2 | Engineering | 25 |
The employee Vannie Doulden
is queried with the { populate: true }
option, which includes the references Department
and Location
as objects into the result.
When the { populate: true }
option is not set, the IDs
of the references Department
and Location
are added instead of objects to the result.
const EmployeeDB = async workerbase.databases.get("Employee");
const result = await EmployeeDB.items.query({ Name: 'Vannie Doulden' }, { populate: true });
The result
variable will contain the employee Vannie Doulden
with populated references.
[
{
"_id": "634fc1b3d64ce49dd554c24c",
"meta": {
"createdAt": "2022-10-19T09:21:55.713Z",
"updatedAt": "2022-10-19T09:22:24.916Z"
},
"database": "634fbc5cd64ce49dd554bdb0",
"payload": {
"Name": "Vannie Doulden",
"Email": "vannie@company.com",
"Birthday": "1988-11-12T00:00:00.000Z",
"Department": {
"_id": "634fbc85d64ce49dd554bdd1",
"meta": {
"createdAt": "2022-10-19T08:59:49.755Z",
"updatedAt": "2022-10-19T09:00:04.742Z"
},
"database": "634fbc75d64ce49dd554bdc2",
"payload": {
"Name": "Product Management",
"Headcount": 10
},
"deleted": false,
"externalId": "1"
},
"Office": {
"_id": "634fc1905025ac5347b22020",
"externalId": "649f9110-4f8f-11ed-967c-0b922c03bae6",
"name": "North America / New York"
}
},
"deleted": false,
"externalId": "1"
}
]
In order to filter on references of items, conditions on nested properties have to be added to the query
parameter. Nested properties are specified by adding $
in the beginning and end of a path i.e. $Office.Name$
.
Please keep in mind that nested property queries can only be used when
{ populate: true }
is added to theoptions
parameter.
Example: All employees whose Department
has a Headcount
less than 20
should be queried.
The example is based on the data shown in the section Population.
const EmployeeDB = async workerbase.databases.get("Employee");
const result = await EmployeeDB.items.query({ "$Department.Headcount$": { $lt: 20 } }, { populate: true });
The result
variable will contain the employee Vannie Doulden
.
[
{
"_id": "634fc1b3d64ce49dd554c24c",
"meta": {
"createdAt": "2022-10-19T09:21:55.713Z",
"updatedAt": "2022-10-19T09:22:24.916Z"
},
"database": "634fbc5cd64ce49dd554bdb0",
"payload": {
"Name": "Vannie Doulden",
"Email": "vannie@company.com",
"Birthday": "1988-11-12T00:00:00.000Z",
"Department": {
"_id": "634fbc85d64ce49dd554bdd1",
"meta": {
"createdAt": "2022-10-19T08:59:49.755Z",
"updatedAt": "2022-10-19T09:00:04.742Z"
},
"database": "634fbc75d64ce49dd554bdc2",
"payload": {
"Name": "Product Management",
"Headcount": 10
},
"deleted": false,
"externalId": "1"
},
"Office": {
"_id": "634fc1905025ac5347b22020",
"externalId": "649f9110-4f8f-11ed-967c-0b922c03bae6",
"name": "North America / New York"
}
},
"deleted": false,
"externalId": "1"
}
]
Workinstruction steps, buttons, and actions can now be defined with the SDK:
import workerbase from '@workerbase/sdk';
const infoText = workerbase.steps.InfoText({
title: 'Info Text Step',
description: '',
});
infoText.showAsBox({ hideTitleBar: true });
const photoStep = workerbase.steps.InfoPhoto({
title: 'Info Photo Step',
mediaId: '5d9f0653e762e70006ab9ecd',
description: '',
});
const flexStep = workerbase.steps.LayoutFlex({
title: 'Layout Flex Step',
direction: workerbase.FLEX_DIRECTION.COLUMN,
});
const b1 = workerbase.button({ action: workerbase.actions.Close() });
const b2 = workerbase.button({ action: workerbase.actions.Finish() });
flexStep.addButtons([b1, b2]);
const columnFlexGroup = flexStep.addColumn({ flex: 2 });
columnFlexGroup.addStep({ flex: 2, step: infoText });
flexStep.addStep({ flex: 2, step: photoStep });
import workerbase from '@workerbase/sdk';
const close = workerbase.actions.Close();
const finish = workerbase.actions.Finish();
const suspend = workerbase.actions.Suspend();
const nextStepFunctionId = workerbase.actions.NextStepFunctionId('functionId');
const nextActionFunctionId = workerbase.actions.NextActionFunctionId('functionId');
const nextStepId = workerbase.actions.NextStepId('stepId');
const nextTaskId = workerbase.actions.NextTaskId(
'taskId',
// optional values:
{
currentTaskAction: workerbase.CURRENT_TASK_ACTION.FINISH, // default is CURRENT_TASK_ACTION.CLOSE
initialStepId: 'initial step id', // default is undefined
},
);
const nextWorkinstruction = workerbase.actions.NextWorkinstruction(
'workinstructionsId',
// optional values:
{
currentTaskAction: workerbase.CURRENT_TASK_ACTION.FINISH, // default is CURRENT_TASK_ACTION.CLOSE
initialStepId: 'initial step id', // default is undefined
},
);
More information about CURRENT_TASK_ACTION
enum can be viewed here.
The SDK also allows to add buttons. Each button is uniquely characterized by its action, which is a mandatory input parameter.
import workerbase from '@workerbase/sdk';
const finishAction = workerbase.actions.Finish();
const button = workerbase.button({
action: finishAction,
});
In addition, each button can have optional values like text
, icon
, and id
. If any of these values are not provided, the SDK will assign default values automatically. To specify the variables for each button, you can follow the following approach:
import workerbase from '@workerbase/sdk';
const finishAction = workerbase.actions.Finish();
const button = workerbase.button({
action: finishAction,
text: 'Button Text',
icon: workerbase.ACTION_ICONS.CLOSE,
id: 'my id',
});
-> More information on ACTION_ICONS
can be viewed here.
You also have the option to personalize the background color and text color of each button. This can be done through the following methods:
import workerbase from '@workerbase/sdk';
const button = workerbase.button({
action: workerbase.actions.Finish(),
});
// Default is #287AF5;
button.setBackgroundColor('#00FFFF');
// Default is #DEEDFF;
button.setTextColor('#89CFF0');
The SDK provides various steps you can add to the function.
Each step has an optional id
value. If it is not specified, the SDK will automatically generate a value.
The following functions can be called for each step:
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoText({
title: '',
description: '',
});
// Add one button
step.addButton(workerbase.button({ action: workerbase.actions.Close() }));
// Add multiple buttons
const b1 = workerbase.button({ action: workerbase.actions.Suspend() });
const b2 = workerbase.button({ action: workerbase.actions.Finish() });
step.addButtons([b1, b2]);
// Set maxTime:
step.setMaxTime(100);
// Load variables from a function:
step.setLoadVariablesFromUrl('functionId');
You can add rich text support via HTML for each step containing a description
variable:
import workerbase from '@workerbase/sdk';
// H1
const description = '<h1> Hello, World! </h1>';
// H2
const description2 = '<h2> Hello, World! </h2>';
// H3
const description3 = '<h3> Hello, World! </h3>';
// Bold
const description4 = '<strong> Hello, World! </strong>';
// Italic
const description5 = '<em> Hello, World! </em>';
// Underline
const description6 = '<u> Hello, World! </u>';
// Align Left
const description7 = '<div style="text-align: left;"> Hello, World! </div>';
// Align Center
const description8 = '<div style="text-align: center;"> Hello, World! </div>';
// Align Right
const description9 = '<div style="text-align: right;"> Hello, World! </div>';
// More complex descriptions can be built with this:
const description10 =
'<div style="text-align: center;"><h1>Hello, <em>World.</em></h1></div> What a <u>wonderful</u> day.';
Info Text Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoText({
title: '',
description: '',
id: '', // optional
});
Info Text Large Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoTextLarge({
title: '',
description: '',
id: '', // optional
});
Info Qr Code Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoQrCode({
title: '',
payload: '',
id: '', // optional
});
Info Status Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoStatus({
title: '',
description: '',
headline: '',
id: '', // optional
});
// Status screen icon is icon-info.png by default
step.setIcon(workerbase.STATUS_SCREEN_ICONS.SUCCESS);
// Status screen color is grey by default
step.setColor(workerbase.STATUS_SCREEN_COLORS.GREEN);
-> More information on STATUS_SCREEN_COLORS
and STATUS_SCREEN_ICONS
enums can be found here.
Info WebView Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoWebview({
title: '',
url: '',
id: '', // optional
});
Info Photo Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoPhoto({
title: '',
mediaId: '',
description: '',
id: '', // optional
});
// "Show full picture" is true by default.
step.showFullPicture(false);
Info Audio Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoAudio({
title: '',
mediaId: '',
description: '',
id: '', // optional
});
Info Video Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoVideo({
title: '',
mediaId: '',
description: '',
id: '', // optional
});
Info Pdf Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoPdf({
title: '',
mediaId: '',
description: '',
id: '', // optional
});
// Slide mode is enabled (true) by default
step.enableSlideMode();
step.disableSlideMode();
// Default page number is 1
step.setPageNumber(3);
Info Loading Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoLoading({
title: '',
taskAppearingDuration: '',
id: '', // optional
});
Info Location Direction Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InfoLocationDirection({
title: '',
gpsLocationLatitude: '',
gpsLocationLongitude: '',
description: '',
id: '', // optional
});
Input Text Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputText({
title: '',
outputVarName: '',
description: '',
id: '', // optional
});
Input Barcode Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputBarcode({
title: '',
outputVarName: '',
description: '',
id: '', // optional
});
// Auto-Continue is false by default
step.setAutoContinue(true);
// Allow Manual Barcode Input is false by default
step.setAllowManualBarcodeInput(true);
// Regex is an empty array by default
step.addRegex('d');
step.addMultipleRegex(['d', 'code']);
// Regex operations is empty array by default
// Begins, Contains, Ends, Equals, EqualsVar, and Regex functions mimic behavior of Workerbase UI
step.addRegexOperation(workerbase.steps.regex.Begins('abc'));
step.addRegexOperations([
workerbase.steps.regex.Contains('abc'),
workerbase.steps.regex.Ends('abc'),
workerbase.steps.regex.Equals('abc'),
workerbase.steps.regex.EqualsVar('abc'),
workerbase.steps.regex.Regex('abc'),
]);
Input Number Picker Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputNumberPicker({
title: '',
outputVarName: '',
description: '',
id: '', // optional
});
// Integer Digits is 3 by default
step.setIntegerDigits(2);
// Decimal Digits is 0 by default
step.setDecimalDigits(3);
// Default Value Decimal is undefined by default
step.setDefaultValueDecimal('10.000');
Input Number Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputNumber({
title: '',
outputVarName: '',
id: '', // optional
});
// Integer Digits is 3 by default
step.setIntegerDigits(2);
// Decimal Digits is 0 by default
step.setDecimalDigits(3);
// Default Value Decimal is undefined by default
step.setDefaultValueDecimal('10.000');
Input Location Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputLocation({
title: '',
outputVarName: '',
minAccuracyInMeters: 3,
description: '',
id: '', // optional
});
Input Audio Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputAudio({
title: '',
outputVarName: '',
id: '', // optional
});
Input Video Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputVideo({
title: '',
outputVarName: '',
id: '', // optional
});
Input Photo Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputPhoto({
title: '',
outputVarName: '',
id: '', // optional
});
Input Noise Level Step
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputNoiseLevel({
title: '',
outputVarName: '',
measureDurationInSec: 5,
id: '', // optional
});
// Auto Continue is false by default
step.setAutoContinue(true);
// Auto Start Measurement is true by default
step.setAutoStartMeasurement(false);
// Input Required is true by default
step.setInputRequired(false);
// Warning Threshold In Decibel is 0 by default
step.setWarningThresholdInDecibel(200);
For each Input List Step, you can add custom options or load options from a function.
The options input type (options array, or function ID) can be specified with the source
input variable and LIST_OPTIONS_SOURCE
enum. More information about this enum can be found here.
Input Value List Step
Options for this step can be added with
ValueListOption
method:
import workerbase from '@workerbase/sdk';
workerbase.steps.options.ValueListOption({
text: '',
id: '', // optional
action: ... , // optional
custom: ... , // optional
});
The Step can be defined as follows:
import workerbase from '@workerbase/sdk';
// Adding own options
const step = workerbase.steps.InputValueList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.OPTIONS, // set source to OPTIONS
id: '', // optional
});
// Auto Continue is false by default
step.setAutoContinue(true);
// Require Selection is false by default
step.setRequireSelection(true);
// Adding Input Value List Options without actions manually
step.addOption(workerbase.steps.options.ValueListOption({ text: '1' }));
step.addOptions([
workerbase.steps.options.ValueListOption({ text: '2' }),
workerbase.steps.options.ValueListOption({ text: '3' }),
]);
// Add Input Value List Options with actions manually
step.setCustomNextStep(true); // Default is false. Has to be set to true to be able to add option with actions.
step.addOption(
workerbase.steps.options.ValueListOption({
text: '1',
action: workerbase.actions.Close(),
}),
);
// Add custom variables
step.addOption(
workerbase.steps.options.ValueListOption({
text: '1',
action: workerbase.actions.Close(),
custom: { yourVariableName: 'yourVariableValue' },
}),
);
// Adding Input Value List Options from a function
const optionsFromFunction = workerbase.steps.InputValueList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.CONNECTOR, // set source to CONNECTOR
id: '', // optional
});
optionsFromFunction.setLoadOptionsFromUrl('functionId');
Input Index List Step
Options for this step can be added with IndexListOption
method:
import workerbase from '@workerbase/sdk';
workerbase.steps.options.IndexListOption({
text: '',
isHeadline: false,
id: '', // optional
custom: ... , // optional
});
The Step can be defined as follows:
import workerbase from '@workerbase/sdk';
// Adding options manually
const step = workerbase.steps.InputIndexList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.OPTIONS, // set source to OPTIONS
id: '', // optional
});
// Auto Continue is false by default
step.setAutoContinue(true);
// Require Selection is false by default
step.setRequireSelection(true);
// Adding Index List Options manually (no options with actions can be added for this step)
step.addOption(
workerbase.steps.options.IndexListOption({
text: '1',
isHeadline: true,
}),
);
step.addOptions([
workerbase.steps.options.IndexListOption({ text: '2', isHeadline: true }),
workerbase.steps.options.IndexListOption({ text: '3', isHeadline: false }),
]);
// Auto Continue is false by default
step.setAutoContinue(true);
// Require Selection is false by default
step.setRequireSelection(true);
// Add Input Index List Options from a function
const optionsFromFunction = workerbase.steps.InputIndexList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.CONNECTOR, // set source to CONNECTOR
id: '', // optional
});
optionsFromFunction.setLoadOptionsFromUrl('functionId');
Input Tile List Step
Options for this step can be added with TileListOption
method:
import workerbase from '@workerbase/sdk';
workerbase.steps.options.TileListOption({
text: '',
id: '', // optional
action: ... , // optional
custom: ... , // optional
});
The Step can be defined as follows:
import workerbase from '@workerbase/sdk';
// Add options manually
const step = workerbase.steps.InputTileList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.OPTIONS, // set source to OPTIONS
id: '', // optional
});
// Add Tile List Options manually
step.addOption(
workerbase.steps.options.TileListOption({
text: '1',
custom: { test: 'test' }, // optional
}),
);
step.addOptions([
workerbase.steps.options.TileListOption({ text: '2' }),
workerbase.steps.options.TileListOption({ text: '3' }),
]);
// Add Input Value List Options with actions manually
step.setCustomNextStep(true); // Default is false. Has to be set to true to be able to add option with actions.
step.addOption(
workerbase.steps.options.TileListOption({
text: '1',
action: workerbase.actions.Close(),
}),
);
// Adding Tile List Options with actions
step.setCustomNextStep(true); // default is false
step.addOption(
workerbase.steps.options.TileListOption({
text: 'text',
action: workerbase.actions.Suspend(),
custom: { test: 'test' }, // optional
}),
);
// Add Tile List Options from a function
const optionsFromFunction = workerbase.steps.InputTileList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.CONNECTOR, // set source to CONNECTOR
id: '', // optional
});
optionsFromFunction.setLoadOptionsFromUrl('functionId');
Input Checkbox List
Options for this step can be added with CheckboxListOption
method:
import workerbase from '@workerbase/sdk';
workerbase.steps.options.CheckboxListOption({
text: '',
isChecked: false,
id: '', // optional
custom: ... , // optional
});
The Step can be defined as follows:
import workerbase from '@workerbase/sdk';
// Add options manually
const step = workerbase.steps.InputCheckboxList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.OPTIONS, // set source to OPTIONS
id: '', // optional
});
// Auto Continue is false by default
step.setAutoContinue(true);
// Require to select all options
// Default is false
step.setSelectAll(true);
// Add minimum number of options that have to be selected
// Can only be set if selectAll is false.
step.setMinSelect(3); // Default is 0
// Adding Tile Checkbox Options manually (no actions can be added for this list)
step.addOption(
workerbase.steps.options.CheckboxListOption({
text: 'text',
isChecked: false,
custom: { test: 'test' }, // optional
}),
);
step.addOptions([
workerbase.steps.options.CheckboxListOption({
text: '2',
isChecked: false,
}),
workerbase.steps.options.CheckboxListOption({
text: '3',
isChecked: false,
}),
]);
// Add Checkbox List Options from a function
const optionsFromFunction = workerbase.steps.InputCheckboxList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.CONNECTOR, // set source to CONNECTOR
id: '', // optional
});
optionsFromFunction.setLoadOptionsFromUrl('functionId');
Input Button List
Options for this step can be added with ButtonListOption
method:
import workerbase from '@workerbase/sdk';
workerbase.steps.options.ButtonListOption({
text: '',
id: '',
action: workerbase.actions.Finish(),
icon: workerbase.OPTION_ICONS.INFO,
custom: undefined,
});
The step can be defined as follows:
import workerbase from '@workerbase/sdk';
const step = workerbase.steps.InputButtonList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.OPTIONS,
});
// Auto Continue is false by default
step.setAutoContinue(true);
// Require Selection is false by default
step.setRequireSelection(true);
// No photo is displayed by default
step.displayPhoto('mediaId');
// Adding Button List Options manually
step.addOption(
workerbase.steps.options.ButtonListOption({
text: 'text',
custom: { test: 'test' }, // optional
}),
);
step.addOptions([
workerbase.steps.options.ButtonListOption({ text: '2' }),
workerbase.steps.options.ButtonListOption({ text: '3' }),
]);
// Adding Button List Options with actions
step.setCustomNextStep(true); // default is false
step.addOption(
workerbase.steps.options.ButtonListOption({
text: 'text',
action: workerbase.actions.Suspend(),
custom: { test: 'test' }, // optional
}),
);
// Adding Button List Options with custom icon
step.addOption(
workerbase.steps.options.ButtonListOption({
text: 'text',
custom: { test: 'test' }, // optional
icon: workerbase.OPTION_ICONS.INFO, // optional
}),
);
// Add Button List Options from a function
const optionsFromFunction = workerbase.steps.InputButtonList({
title: '',
outputVarName: '',
source: workerbase.LIST_OPTIONS_SOURCE.CONNECTOR, // set source to CONNECTOR
id: '', // optional
});
optionsFromFunction.setLoadOptionsFromUrl('functionId');
-> More information on OPTION_ICONS
enum can be found here.
We added the possibility to define a custom step, consisting of multiple other steps. This can be done via the FlexStep
.
You can add a single step, or a group of steps to the FlexStep
:
import workerbase from '@workerbase/sdk';
// Define Info Text Step
const infoText = workerbase.steps.InfoText({
title: 'Info Text Step Title',
description: 'This is the description of the step',
});
// Defaults of showAsBox is false, and hideTitleBar is true
infoText.enableShowAsBox(); // showAsBox is now true, and hideTitleBar false
// You can additionally set hideTitleBar to true
infoText.enableShowAsBox({ hideTitleBar: true }); // showAsBox and hideTitleBar are true now
// Define Info Photo Step
const photoStep = workerbase.steps.InfoPhoto({
title: '',
mediaId: '5d9f0653e762e70006ab9ecd',
description: 'This is the description of the photo',
});
const largeText = workerbase.steps.InfoTextLarge({
title: 'Info Text Large',
description: 'Description',
});
// Define Flex Step with two buttons
const flexStep = workerbase.steps.LayoutFlex({
title: 'My Layout Flex Step',
direction: workerbase.FLEX_DIRECTION.COLUMN,
});
const b1 = workerbase.button({ action: workerbase.actions.Close() });
const b2 = workerbase.button({ action: workerbase.actions.Finish() });
flexStep.addButtons([b1, b2]);
// Add FlexGroup placing steps in column direction
const columnFlexGroup = flexStep.addColumn({ flex: 2 });
// Add previously defined Info Text Step to column flex group
columnFlexGroup.addStep({ flex: 2, step: infoText });
// Add FlexGroup placing steps in row direction
const rowFlexGroup = flexStep.addRow({ flex: 2 });
// Add previously defined Info Large Text Step to row flex group
rowFlexGroup.addStep({ flex: 2, step: largeText });
// Add previously defined Info Photo Step to Flex Step
flexStep.addStep({ flex: 2, step: photoStep });
-> More information on FLEX_DIRECTION
enum can be found here.
enum STATUS_SCREEN_ICON {
SUCCESS,
INFO,
ERROR,
}
enum STATUS_SCREEN_COLORS {
GREY,
RED,
GREEN,
}
enum LIST_OPTIONS_SOURCE {
CONNECTOR,
OPTIONS,
}
enum ACTION_ICONS {
CONFIRM,
CLOSE,
QUESTION_YELLOW,
REPEAT,
WARNING_RED_CLEAR_BG,
WARNING_RED,
WARNING_YELLOW,
WARNING,
REFRESH,
INFO,
INFO_BLUE,
BACK,
NEXT,
CONFIRM_ENCIRCLED,
CONFIRM_ENCIRCLED_GREEN,
PLUS,
MINUS,
INSTRUCTIONS,
LIST,
HOME,
}
enum OPTION_ICONS {
INFO,
ERROR,
SUCCESS,
SUPPORT,
}
enum FLEX_DIRECTION {
ROW,
COLUMN,
}
enum CURRENT_TASK_ACTION {
FINISH,
CLOSE,
SUSPEND,
}
FAQs
Documentation is accessible here: https://workerbase.zendesk.com/hc/en-us/articles/14805311093277-Workerbase-SDK-for-JavaScript
The npm package @workerbase/sdk receives a total of 5 weekly downloads. As such, @workerbase/sdk popularity was classified as not popular.
We found that @workerbase/sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.