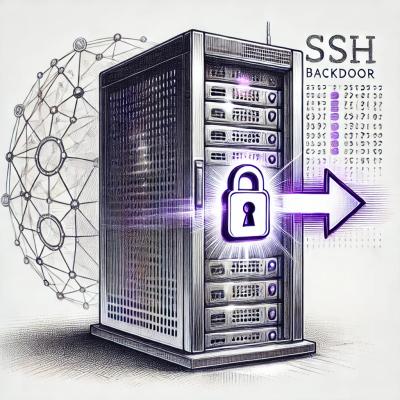
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@xmtp/xmtp-js
Advanced tools
The XMTP SDK bundles the core code libraries, components, tools, documentation, and guides that developers require in order to build client experiences on top of the XMTP protocol and network.
The API revolves around a network Client that allows retrieving and sending messages to other network participants. A Client must be connected to a wallet on startup. If this is the very first time the Client is created, the client will generate a key bundle that is used to encrypt and authenticate messages. The key bundle persists encrypted in local storage using a wallet signature. The public side of the key bundle is also regularly advertised on the network to allow parties to establish shared encryption keys. All this happens transparently, without requiring any additional code.
import { Client } from 'xmtp-js'
import { Wallet } from 'ethers'
// You'll want to replace this with a wallet from your application
const wallet = Wallet.createRandom()
// Create the client with your wallet. This will connect to the XMTP testnet by default
const xmtp = await Client.create(wallet)
// Start a conversation with Vitalik
const conversation = await xmtp.conversations.newConversation(
'0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045'
)
// Load all messages in the conversation
const messages = await conversation.messages()
// Send a message
await conversation.send('gm')
// Listen for new messages in the conversation
for await (const message of conversation.streamMessages()) {
console.log(`[${message.senderAddress}]: ${message.text}`)
}
A Client is created with Client.create(wallet: ethers.Signer): Promise<Client>
that requires passing in a connected Wallet. The Client will request a wallet signature in 2 cases:
The Client will connect to XMTP testnet by default. CreateOptions can be used to override this and other parameters of the network connection.
Note that currently the Client uses browser's local storage, so starting it on a different device or browser and connecting to the same wallet will create a "split identity" situation where only one of the clients will be able to decrypt given incoming message depending on which of the advertised key bundles the sender chose to use. Similarly if local storage is cleared for whatever reason and a new key bundle is created, older messages encrypted with older bundles cannot be decrypted anymore and will cause the client to throw.
import { Client } from 'xmtp-js'
// Create the client with an `ethers.Signer` from your application
const xmtp = await Client.create(wallet)
Most of the time, when interacting with the network, you'll want to do it through conversations
.
import { Client } from 'xmtp-js'
// Create the client with an `ethers.Signer` from your application
const xmtp = await Client.create(wallet)
const conversations = xmtp.conversations
You can get a list of all conversations that have had 1 or more messages exchanged in the last 30 days.
const allConversations = await xmtp.conversations.list()
// Say gm to everyone you've been chatting with
for (const conversation of allConversations) {
console.log(`Saying GM to ${conversation.peerAddress}`)
await conversation.send('gm')
}
You can also listen for new conversations being started in real-time. This will allow applications to display incoming messages from new contacts.
Warning: this stream will continue infinitely. To end the stream you can either break from the loop, or call await stream.return()
const stream = xmtp.conversations.stream()
for await (const conversation of stream) {
console.log(`New conversation started with ${conversation.peerAddress}`)
// Say hello to your new friend
await conversation.send('Hi there!')
// Break from the loop to stop listening
break
}
You can create a new conversation with any Ethereum address on the XMTP network.
const newConversation = await xmtp.conversations.newConversation(
'0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045'
)
To be able to send a message, the recipient must have already started their Client at least once and consequently advertised their key bundle on the network. Messages are addressed using wallet addresses. Message payload is a string but neither the SDK nor the network put any constraints on its contents or interpretation.
const conversation = await xmtp.conversations.newConversation(
'0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045'
)
await conversation.send('Hello world')
You can receive the complete message history by calling conversation.messages()
for (const conversation of await xmtp.conversations.list()) {
// All parameters are optional and can be omitted
const opts = {
// Only show messages from last 24 hours
startTime: new Date(new Date().setDate(new Date().getDate() - 1)),
endTime: new Date(),
}
const messagesInConversation = await conversation.messages(opts)
}
You can listen for any new messages (incoming or outgoing) in a conversation by calling conversation.streamMessages()
.
A successfully received message (that makes it through the decoding and decryption without throwing) can be trusted to be authentic, i.e. that it was sent by the owner of the message.senderAddress
wallet and that it wasn't modified in transit. The message.sent
timestamp can be trusted to have been set by the sender.
The Stream returned by the stream
methods is an asynchronous iterator and as such usable by a for-await-of loop. Note however that it is by its nature infinite, so any looping construct used with it will not terminate, unless the termination is explicitly initiated (by breaking the loop or by an external call to Stream.return()
)
const conversation = await xmtp.conversations.newConversation(
'0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045'
)
for await (const message of conversation.streamMessages()) {
if (message.senderAddress === xmtp.address) {
// This message was sent from me
continue
}
console.log(`New message from ${message.senderAddress}: ${message.text}`)
}
Using xmtp.conversations
hides the details of this, but for the curious this is how sending a message on XMTP works. The first message and first response between two parties is sent to three separate topics:
This is used to establish a shared secret and negotiate a topic to communicate on. Any following messages are sent to the conversation topic only.
The introduction topics allow the participants to reconstruct the list of conversations that they participate(d) in.
The conversation topics carry the contents of the conversations.
A new version of this package will be automatically published whenever there is a merge to the main
branch. Specifically, new GitHub releases and tags will be created, and a new NPM package version will be published. The release version increment type is derived from the commits that were bundled in the merge to main
, using semantic-release commit message conventions.
The table below shows example commits and the resulting release type:
Commit message | Release type |
---|---|
fix(pencil): stop graphite breaking when too much pressure applied | |
feat(pencil): add 'graphiteWidth' option | |
perf(pencil): remove graphiteWidth option BREAKING CHANGE: The graphiteWidth option has been removed. The default graphite width of 10mm is always used for performance reasons. | (Note that the BREAKING CHANGE: token must be in the footer of the commit) |
This is currently configured to use the Angular Commit Message Conventions.
Node
Please make sure you have a Node version compatible with that specified in the root .nvmrc
file. We recommend using nvm
to manage local node versions - find install instructions appropriate for your system here.
FAQs
XMTP client SDK for interacting with XMTP networks.
We found that @xmtp/xmtp-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.