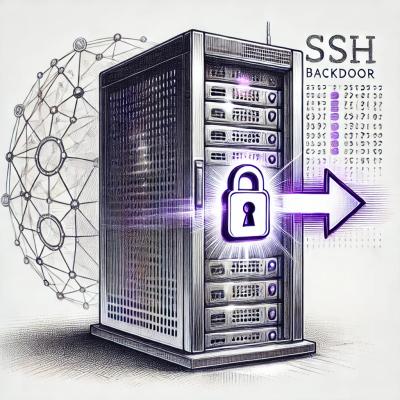
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@yaireo/tagify
Advanced tools
Want to simply convert an input field into a tags element, in a easy customizable way, with good performance and smallest code footprint? You are in the right place my friend.
Transforms an input field or a textarea into a Tags component, in an easy, customizable way, with great performance and tiny code footprint.
hellip
by giving max-width
to the tag
element in your CSS
Simply run gulp
in your terminal, from the project's path (Gulp should be installed first).
Source files are this path /src/
Output files, which are automatically generated using Gulp, are in /dist/
;
The rest of the files are most likely irrelevant.
Lets say this is your markup, and you already have a value set on the input (which was pre-filled by data from the server):
<input name='tags' placeholder='write some tags' value='foo, bar,buzz'>
<textarea name='tags' pattern=".{3,}" placeholder='write some tags'>foo, bar,buzz</textarea>
What you need to do to convert that nice input into "tags" is simply select your input/textarea and run tagify()
:
// vanilla component
var input = document.querySelector('input[name=tags]'),
tagify = new Tagify( input );
// with settings passed
tagify = new Tagify( input, {
duplicates: true,
whitelist: ['foo', 'bar'],
callbacks: {
add : onAddTag // calls an imaginary "onAddTag" function when a tag is added
}
});
// listen to custom tags' events such as 'add' or 'remove' (see full list below).
// listeners are chainable
tagify.on('remove', onTagRemoved)
.on('add', onTagAdded);
function onTagRemoved(e){
console.log(e, e.detail);
// remove listener after first tag removal
tagify.off('remove', onTagRemoved);
}
function onTagAdded(e){
// do whatever
}
The value of the Tagify component can be accessed like so:
var tagify = new Tagify(...);
console.log( tagify.value )
// [{"value":"tag1"}, {"value":"tag2"}, ...]
If the Tags were added with custom properties, the value output might look something like this:
tagify.value
// [{ "value":"tag1", "class":"red", "id":1}, ...]
The below example will populate the Tags component with 2 tags, each with specific attributes & values.
the addTags
method accepts an Array of Objects with any key/value, as long as the value
key is defined.
<input placeholder="add tags">
```javascript
var allowedTags = [
{
"value" : "apple",
"data-id" : 3,
"class" : 'color-green'
},
{
"value" : "orange",
"data-id" : 56,
"class" : 'color-orange'
},
{
"value" : "passion fruit",
"data-id" : 17,
"class" : 'color-purple'
},
{
"value" : "banana",
"data-id" : 12,
"class" : 'color-yellow'
},
{
"value" : "paprika",
"data-id" : 25,
"class" : 'color-red'
}
];
var input = document.querySelector('input'),
tagify = new Tagify(input,
whitelist : allowedTags
);
// Add the first 2 tags from the "allowedTags" Array
tagify.addTags( allowedTags.slice(0,2) )
The above will prepend a "tags" element before the original input element:
<tags class="tagify">
<tag readonly="true" class="color-red" data-id="8" value="strawberry">
<x></x><div><span title="strawberry">strawberry</span></div>
</tag>
<tag readonly="true" class="color-darkblue" data-id="6" value="blueberry">
<x></x><div><span title="blueberry">blueberry</span></div>
</tag>
<div contenteditable data-placeholder="add tags" class="tagify--input"></div>
</tags>
<input placeholder="add tags">
The suggestions selectbox is shown is a whitelist Array of Strings or Objects was passed in the settings when the Tagify instance was created. Suggestions list will only be rendered if there were at least two sugegstions found. Matching suggested values is case-insensetive. The selectbox dropdown will be appended to the document's "body" element and will be positioned under the element. Using the keyboard arrows up/down will highlight an option from the list, and hitting the Enter key to select.
It is possible to tweak the selectbox dropdown via 2 settings:
var input = document.querySelector('input'),
tagify = new Tagify(input,
whitelist : ['aaa', 'aaab', 'aaabb', 'aaabc', 'aaabd', 'aaabe', 'aaac', 'aaacc'],
dropdown : {
classname : "color-blue",
enabled : 3,
maxItems : 5
}
);
Will render:
<div class="tagify__dropdown" style="left: 993.5px; top: 106.375px; width: 616px;">
<div class="tagify__dropdown__item" value="aaab">aaab</div>
<div class="tagify__dropdown__item" value="aaabb">aaabb</div>
<div class="tagify__dropdown__item" value="aaabc">aaabc</div>
<div class="tagify__dropdown__item" value="aaabd">aaabd</div>
<div class="tagify__dropdown__item" value="aaabe">aaabe</div>
</div>
$('[name=tags]')
.tagify()
.on('add', function(e, tagName){
console.log('added', tagName)
});
Name | Info |
---|---|
destroy | Reverts the input element back as it was before Tagify was applied |
removeAllTags | Removes all tags and resets the original input tag's value property |
addTags | Accepts a String (word, single or multiple with a delimiter) or an Array of Objects (see above) |
removeTag | Removes a specific tag (argument is the tag DOM element to be removed. see source code.) |
Name | Info |
---|---|
add | A tag has been added |
remove | A tag has been removed |
duplicate | A tag has been added and found to be a duplicate of existing one |
maxTagsExceed | Number of tags exceeds the allowed quantity and the exceed tags were denied (removed) |
blacklisted | A tag which is in the blacklist has been added and denied (removed) |
notWhitelisted | A tag which is not in the whitelist has been added and denied (removed) |
Name | Type | Default | Info |
---|---|---|---|
delimiters | String | "," | [regex] split tags by any of these delimiters. Example: ", |
pattern | String | null | Validate input by REGEX pattern (can also be applied on the input itself as an attribute) Ex: /[1-9]/ |
duplicates | Boolean | false | (flag) should duplicate tags be allowed or not |
enforceWhitelist | Boolean | false | should ONLY use tags allowed in whitelist |
autocomplete | Boolean | true | tries to autocomplete the input's value while typing (match from whitelist) |
whitelist | Array | [] | an array of tags which only they are allowed |
blacklist | Array | [] | an array of tags which aren't allowed |
addTagOnBlur | Boolean | true | automatically adds the text which was inputed as a tag when blur event happens |
callbacks | Object | {} | exposed callbacks object to be triggered on events: 'add' / 'remove' tags |
maxTags | Number | Infinity | max number of tags |
dropdown.enabled | Number | 2 | minimum characters to input which shows the suggestions list dropdown |
dropdown.maxItems | Number | 10 | maximum items to show in the suggestions list dropdown |
dropdown.classname | String | "" | custom class name for the dropdown suggestions selectbox |
FAQs
lightweight, efficient Tags input component in Vanilla JS / React / Angular [super customizable, tiny size & top performance]
The npm package @yaireo/tagify receives a total of 63,401 weekly downloads. As such, @yaireo/tagify popularity was classified as popular.
We found that @yaireo/tagify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.