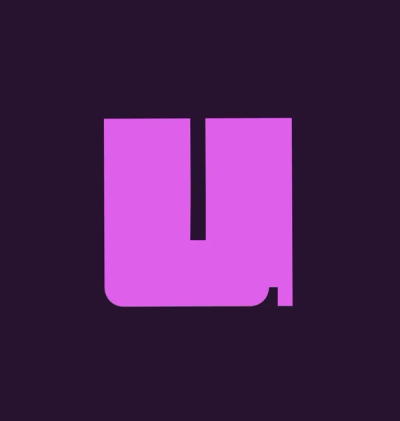
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@zenfs/core
Advanced tools
ZenFS is an in-browser file system that emulates the Node JS file system API and supports storing and retrieving files from various backends. ZenFS also integrates nicely with other tools.
ZenFS is highly extensible, and includes many builtin filesystem backends:
InMemory
: Stores files in-memory. It is a temporary file store that clears when the user navigates away.Overlay
: Mount a read-only file system as read-write by overlaying a writable file system on top of it. Like Docker's overlayfs, it will only write changed files to the writable file system.AsyncMirror
: Use an asynchronous backend synchronously. Invaluable for Emscripten; let your Emscripten applications write to larger file stores with no additional effort!
AsyncMirror
loads the entire contents of the async file system into a synchronous backend during construction. It performs operations synchronous file system and then queues them to be mirrored onto the asynchronous backend.More backends can be defined by separate libraries, so long as they extend they implement ZenFS.FileSystem
. Multiple backends can be active at once at different locations in the directory hierarchy.
ZenFS supports a number of other backends (many are provided as seperate packages under @zenfs
).
For more information, see the API documentation for ZenFS.
npm install @zenfs/core
🛈 The examples are written in ESM. If you are using CJS, you can
require
the package. If running in a browser you can add a script tag to your HTML pointing to thebrowser.min.js
and use ZenFS via the globalZenFS
object.
import fs from '@zenfs/core';
fs.writeFileSync('/test.txt', 'Cool, I can do this in the browser!');
const contents = fs.readFileSync('/test.txt', 'utf-8');
console.log(contents);
A InMemory
backend is created by default. If you would like to use a different one, you must configure ZenFS. It is recommended to do so using the configure
function. Here is an example using the Storage
backend from @zenfs/dom
:
import { configure, fs } from '@zenfs/core';
import { StorageStore } from '@zenfs/dom';
await configure({ backend: StorageStore });
if (!fs.existsSync('/test.txt')) {
fs.writeFileSync('/test.txt', 'This will persist across reloads!');
}
const contents = fs.readFileSync('/test.txt', 'utf-8');
console.log(contents);
You can use multiple backends by passing an object to configure
which maps paths to file systems. The following example mounts a zip file to /zip
, in-memory storage to /tmp
, and IndexedDB storage to /home
(note that /
has the default in-memory backend):
import { configure } from '@zenfs/core';
import { IndexedDB } from '@zenfs/dom';
import { Zip } from '@zenfs/zip';
const zipData = await (await fetch('mydata.zip')).arrayBuffer();
await configure({
'/mnt/zip': {
backend: Zip,
zipData: zipData,
},
'/tmp': 'InMemory',
'/home': IndexedDB,
};
The FS promises API is exposed as promises
.
import { configure, promises } from '@zenfs/core';
import { IndexedDB } from '@zenfs/dom';
await configure({ '/': IndexedDB });
const exists = await promises.exists('/myfile.txt');
if (!exists) {
await promises.write('/myfile.txt', 'Lots of persistant data');
}
ZenFS does not provide a seperate public import for importing promises in its built form. If you are using ESM, you can import promises functions from dist/emulation/promises
, though this may change at any time and is not recommended.
You may have noticed that attempting to use a synchronous function on an asynchronous backend (e.g. IndexedDB) results in a "not supplied" error (ENOTSUP
). If you wish to use an asynchronous backend synchronously you need to wrap it in an AsyncMirror
:
import { configure, fs } from '@zenfs/core';
import { IndexedDB } from '@zenfs/dom';
await configure({
'/': {
backend: 'AsyncMirror',
sync: 'InMemory',
async: IndexedDB,
},
});
fs.writeFileSync('/persistant.txt', 'My persistant data'); // This fails if you configure with only IndexedDB
If you would like to create backends without configure, you may do so by importing the backend and calling createBackend
with it. You can import the backend directly or with backends
:
import { configure, backends, InMemory } from '@zenfs/core';
console.log(backends.InMemory === InMemory); // they are the same
const internalInMemoryFS = await createBackend(InMemory);
⚠ Instances of backends follow the internal ZenFS API. You should never use a backend's methods unless you are extending a backend.
import { configure, InMemory } from '@zenfs/core';
const internalInMemoryFS = new InMemory();
await internalInMemoryFS.ready();
If you would like to mount and unmount backends, you can do so using the mount
and umount
functions:
import { fs, InMemory } from '@zenfs/core';
const internalInMemoryFS = await createBackend(InMemory); // create an FS instance
fs.mount('/tmp', internalInMemoryFS); // mount
fs.umount('/tmp'); // unmount /tmp
This could be used in the "multiple backends" example like so:
import { IndexedDB } from '@zenfs/dom';
import { Zip } from '@zenfs/zip';
await configure({
'/tmp': 'InMemory',
'/home': 'IndexedDB',
};
fs.mkdirSync('/mnt');
const res = await fetch('mydata.zip');
const zipFs = await createBackend(Zip, { zipData: await res.arrayBuffer() });
fs.mount('/mnt/zip', zipFs);
// do stuff with the mounted zip
fs.umount('/mnt/zip'); // finished using the zip
ZenFS exports a drop-in for Node's fs
module (up to the version of @types/node
in package.json), so you can use it for your bundler of preference using the default export.
npm install
npm run build
dist
.Run unit tests with npm test
.
ZenFS is licensed under the MIT License.
FAQs
A filesystem, anywhere
The npm package @zenfs/core receives a total of 7,891 weekly downloads. As such, @zenfs/core popularity was classified as popular.
We found that @zenfs/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.