Zenvia SDK for Node.js
This SDK for Node.js was created based on the Zenvia API.

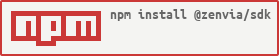

Table of Contents
Changelog
2.4.4
2.4.3
- Added
- Card content to Telegram
- Replyable text content to Telegram
2.4.2
- Fixed
- NPM publishing for 2.4.X versions
2.4.1
- Added
- Card content to Instagram
- Carousel content to Instagram
2.4.0
- Added
- Card content
- Carousel content
- Replyable text content
2.3.0
- Added
- Email content
- Email channel
- Google Business Messages (GBM) channel
- Telegram channel
- Attribute fileName to file content
2.2.0
- Added
- Support to custom request headers
2.1.1
2.1.0
2.0.0
Features
Prerequisites
Installation
npm install @zenvia/sdk
Basic Usage
var zenvia = require('@zenvia/sdk');
import * as zenvia from '@zenvia/sdk';
const client = new zenvia.Client('YOUR_API_TOKEN');
const whatsapp = client.getChannel('whatsapp');
const content = new zenvia.TextContent('some text message here');
whatsapp.sendMessage('sender-identifier', 'recipient-identifier', content)
.then(response => {
})
.catch(error => {
});
try {
const response = await whatsapp.sendMessage('sender-identifier', 'recipient-identifier', content);
} catch (error) {
}
Getting Started
Examples not listed on this section can be found here.
Sending your first message
The content types that can be sent are:
Name | Description |
---|
TextContent | Used to send text messages to your customer. |
FileContent | Used to send file messages to your customer. |
LocationContent | Used to send location messages to your customer. |
ContactsContent | Used to send contacts messages to your customer. |
TemplateContent | Used to send template messages to your customer. |
EmailContent | Used to send e-mail messages to your customer. |
CardContent | Used to send card messages to your customer. |
CarouselContent | Used to send carousel messages to your customer. |
ReplyableTextContent | Used to send replyable text messages to your customer. |
The channels that can be used to send the content are:
Channel | TextContent | FileContent | LocationContent | ContactsContent | TemplateContent | EmailContent | CardContent | CarouselContent | ReplyableTextContent |
---|
SMS | X | | | | X | | | | |
RCS | X | X | | | X | | X | X | X |
WhatsApp | X | X | X | X | X | | | | |
Facebook | X | X | | | | | X | X | X |
Instagram | X | X | | | | | X | X | X |
Email | | | | | | X | | | |
GBM | X | X | | | | | X | X | X |
Telegram | X | X | | | | | X | | X |
Use the sendMessage
method to messages to your customers.
const client = new Client('YOUR_API_TOKEN');
const sms = client.getChannel('sms');
const content = new TextContent('some text message');
const response = await sms.sendMessage('sender-identifier', 'recipient-identifier', content);
The response can be an IMessage
object when successful or an IError
object when an error occurs.
Sending a message batch
Content can be sent as a batch. In other words, sending a message with one or more content to one or multiple contacts. You'll need to send a file and comply with the required fields for each type of batch
The following channels support the following contents to be sent as a batch:
Channel | TextContent | TemplateContent |
---|
SMS | X | |
WhatsApp | | X |
Use the sendMessageBatch
method to send a batched content to your customers.
const client = new Client('YOUR_API_TOKEN');
const smsBatch = {
name: 'My first SMS batch',
channel: 'sms',
message: {
from: 'sender-identifier',
contents: [
{
type: 'text',
text: 'first text message',
},
{
type: 'text',
text: 'second text message',
},
],
},
columnMapper: {
"recipient_header_name": "recipient_number_column",
"name": "recipient_name_column",
"protocol": "protocol_column",
},
};
const batch = client.sendMessageBatch('./path/file.csv', smsBatch);
You may choose to send the content as a string or an array of strings instead of an array of objects. For that, you need to instanciate the WhatsAppMessageBatch
class to send a batched WhatsApp template message or SmsMessageBatch
class when sending a batched SMS text message.
Additionally, instead of sending a file you can send the contents of the file as a stream for both WhatsApp and SMS message batches.
import { Readable } from 'stream';
const client = new Client('SOME_TOKEN');
const contents = [
'a whatsapp template id',
'another whatsapp template id',
];
const columnMapper = {
"recipient_header_name": "recipient_number_column",
"name": "recipient_name_column",
"protocol": "protocol_column",
};
const whatsAppBatch = new WhatsAppMessageBatch(
'My first WhatsApp batch',
'sender-identifier',
contents,
columnMapper,
);
const readStream = Readable.from("telefone\n5511999999999");
const batch = client.sendMessageBatch(readstream, whatsAppBatch);
The response can be an IBatch
object when successful or an IError
object when an error occurs.
Subscribe for messages
Use the createSubscription
method to create a MessageSubscription
object for message subscriptions.
const client = new Client('YOUR_API_TOKEN');
const subscription = new MessageSubscription({
url: 'https://your-webhook.company.com'
},
{
channel: 'sms'
});
const response = await client.createSubscription(subscription);
The response can be an ISubscription
object when successful or an IError
object on errors.
Subscribe for message status
Use the createSubscription
method to create a MessageStatusSubscription
object for message status subscriptions.
const client = new Client('YOUR_API_TOKEN');
const subscription = new MessageStatusSubscription({
url: 'https://your-webhook.company.com'
},
{
channel: 'sms'
});
const response = await client.createSubscription(subscription);
The response can be an ISubscription
object when successful or an IError
object when an error occurs.
Receiving message events and message status events
Use the WebhookController
class to create your webhook so you can receive message events and message status events. The default port is 3000
.
If you inform the client
, url
, and channel
fields, a subscription will be created, unless a subscription matching these configuration already exists.
In the messageEventHandler
field you will receive the message events and in the messageStatusEventHandler
field you will receive the message status events.
const client = new Client(process.env.ZENVIA_API_TOKEN);
const webhook = new WebhookController({
messageEventHandler: (messageEvent) => {
console.log('Message event:', messageEvent);
},
messageStatusEventHandler: (messageStatusEvent) => {
console.log('Message status event:', messageStatusEvent);
},
client,
url: 'https://my-webhook.company.com',
channel: 'whatsapp',
});
webhook.init();
To receive events running the example on your machine, we suggest ngrok.
Getting message reports
To get information on how many messages you've sent or have received during a period of time, use the getEntries
method to list IReportMessagesEntry
objects as shown below.
const client = new Client('YOUR_API_TOKEN');
const reportClient = client.getMessagesReportClient();
const response = await reportClient.getEntries({
startDate: '2020-01-10',
endDate: '2020-01-15',
});
The response can be an array of IReportMessagesEntry
objects when successful or an IError
object when an error occurs.
Getting flow reports
In order to get information about the current state of sessions (executions) of flows in a period of time, use the getEntries
method to list IReportFlowEntry
objects as shown below.
const client = new Client('YOUR_API_TOKEN');
const reportClient = client.getFlowReportClient();
const response = await reportClient.getEntries({ startDate: '2020-01-10' });
The response can be an array of IReportFlowEntry
objects when successful or an IError
object when an error occurs.
Listing your templates
You can execute CRUD operations on templates. For example, use the listTemplates
method to list an ITemplate
object.
const client = new Client('YOUR_API_TOKEN');
const response = await client.listTemplates();
The response will be an array of ITemplate
object.
Contributing
Pull requests are always welcome!
Please consult the Contributors' Guide for more information on contributing.
License
MIT