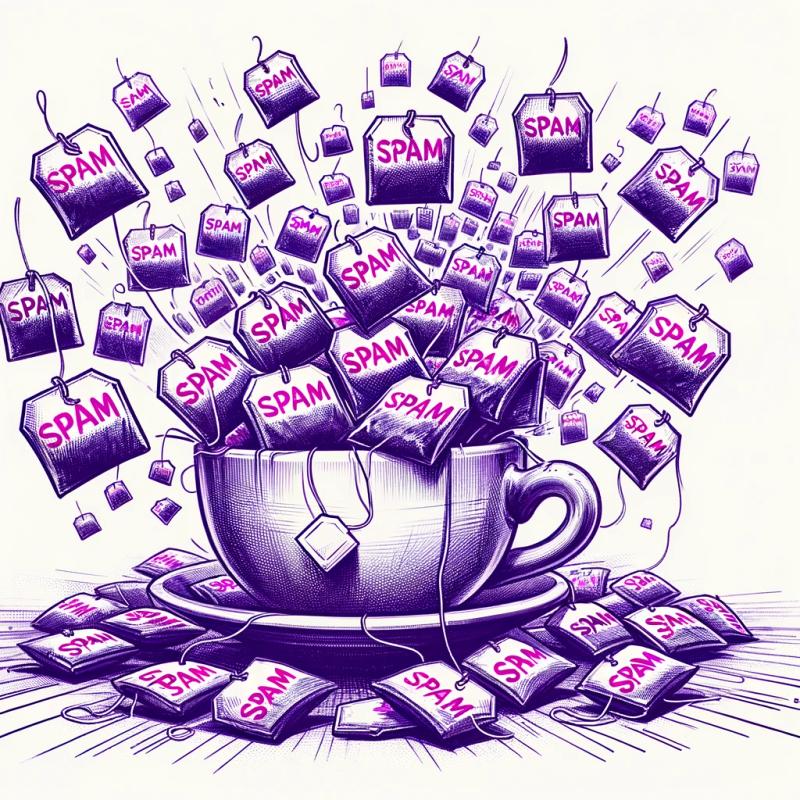
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
a-sync-waterfall
Advanced tools
Readme
Simple, isolated sync/async waterfall module for JavaScript.
Runs an array of functions in series, each passing their results to the next in the array. However, if any of the functions pass an error to the callback, the next function is not executed and the main callback is immediately called with the error.
For browsers and node.js.
npm install a-sync-waterfall
if you’re using node.js.waterfall(tasks, optionalCallback, forceAsync);
callback(err, result1, result2, ...)
it must call on completion. The first
argument is an error (which can be null) and any further arguments will be
passed as arguments in order to the next task.var waterfall = require('a-sync-waterfall');
waterfall(tasks, callback);
var waterfall = require('a-sync-waterfall');
waterfall(tasks, callback);
// Default:
window.waterfall(tasks, callback);
waterfall([
function(callback){
callback(null, 'one', 'two');
},
function(arg1, arg2, callback){
callback(null, 'three');
},
function(arg1, callback){
// arg1 now equals 'three'
callback(null, 'done');
}
], function (err, result) {
// result now equals 'done'
});
/* basic - no arguments */
waterfall(myArray.map(function (arrayItem) {
return function (nextCallback) {
// same execution for each item, call the next one when done
doAsyncThingsWith(arrayItem, nextCallback);
}}));
/* with arguments, initializer function, and final callback */
waterfall([function initializer (firstMapFunction) {
firstMapFunction(null, initialValue);
}].concat(myArray.map(function (arrayItem) {
return function (lastItemResult, nextCallback) {
// same execution for each item in the array
var itemResult = doThingsWith(arrayItem, lastItemResult);
// results carried along from each to the next
nextCallback(null, itemResult);
}})), function (err, finalResult) {
// final callback
});
Hat tip to Caolan McMahon and Paul Miller, whose prior contributions this is based upon. Also Elan Shanker from which this rep is forked
FAQs
Runs a list of async tasks, passing the results of each into the next one
The npm package a-sync-waterfall receives a total of 515,578 weekly downloads. As such, a-sync-waterfall popularity was classified as popular.
We found that a-sync-waterfall demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.