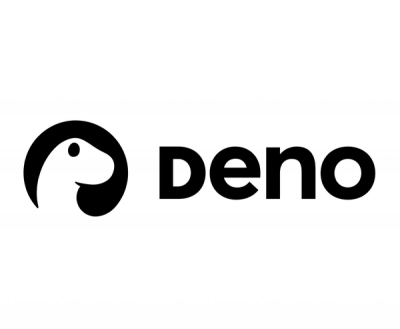
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
abaabil.button
Advanced tools
The Button component is a versatile and accessible button implementation that can be used for various interactive elements in your application.
The Button component is a versatile and accessible button implementation that can be used for various interactive elements in your application.
npm install abaabil.temp.button
Import the Button component from the package:
import Button from 'abaabil.temp.button';
<Button>Click me</Button>
<Button>Click me</Button>
<Button icon="user">Profile</Button>
<Button icon="settings" ariaLabel="Settings" />
<Button actionIcon="arrow-right">Next</Button>
<Button disabled>Unavailable</Button>
<Button as="a" href="/path">Go to Page</Button>
Property | Type | Description | Default | Required |
---|---|---|---|---|
as | 'button' | 'a' | Renders component as button or anchor | 'button' | No |
icon | string | Icon ID to display before text | - | No |
children | ReactNode | Button content | - | No |
actionIcon | string | Icon ID to display after text | - | No |
className | string | Additional CSS classes | - | No |
disabled | boolean | Disables the button | false | No |
isPressed | boolean | Sets aria-pressed state | - | No |
ariaLabel | string | Accessibility label for icon-only buttons | - | No |
The Button component also forwards all other props to the underlying element.
The Button component comes with default styling classes:
max-w-full inline-flex items-center justify-center font-bold tracking-df h-df text-df px-df rounded-df
bg-stable text-on-stable hover:bg-stable-hover focus:bg-stable-focus active:bg-stable-active
bg-stable-disabled text-on-stable-disabled
You can customize the appearance using the className
prop and your own CSS.
The Button component enhances accessibility by:
<button>
or <a>
)aria-label
, aria-pressed
, aria-disabled
)forwardRef
to allow ref forwarding.Icon
component from abaabil.temp.icon
. Ensure this dependency is available in your project.classnames
library for conditional class application.FAQs
Unknown package
The npm package abaabil.button receives a total of 3 weekly downloads. As such, abaabil.button popularity was classified as not popular.
We found that abaabil.button demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.