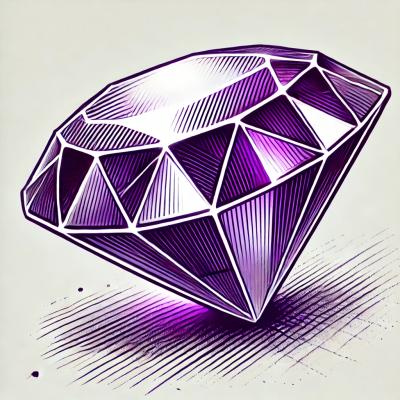
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
This repo contains the ably javascript client libraries, both for the browser and nodejs.
For complete API documentation, see the ably documentation.
npm install ably-js
npm install <git url>
cd </path/to/this/repo>
npm install
For the realtime library:
var realtime = require('ably-js').Realtime;
For the rest-only library:
var rest = require('ably-js').Rest;
Include the Ably library in your HTML:
<script src="https://cdn.ably.io/lib/ably.min.js"></script>
The Ably client library follows Semantic Versioning. To lock into a major or minor verison of the client library, you can specify a specific version number such as http://cdn.ably.io/lib/ably.min-0.8.2.js or http://cdn.ably.io/lib/ably-0.8.2.js for the non-minified version. See https://github.com/ably/ably-js/tags for a list of tagged releases.
For the real-time library:
var realtime = Ably.Realtime;
For the rest-only library:
var rest = Ably.Rest;
All examples assume a client has been created as follows:
// basic auth with an API key
var client = new Ably.Realtime(<key string>)
// using token auth
var client = new Ably.Realtime(<token string>)
Successful connection:
client.connection.on('connected', function() {
# successful connection
});
Failed connection:
client.connection.on('failed', function() {
# failed connection
});
Given:
var channel = client.channels.get('test');
Subscribe to all events:
channel.subscribe(function(message) {
message.name // 'greeting'
message.data // 'Hello World!'
});
Only certain events:
channel.subscribe('myEvent', function(message) {
message.name // 'myEvent'
message.data // 'myData'
});
channel.publish('greeting', 'Hello World!')
channel.history(function(messagesPage) {
messagesPage // PaginatedResult
messagesPage.items // array of Message
messagesPage.items[0].data // payload for first message
messagesPage.items.length // number of messages in the current page of history
messagesPage.next(function(nextPage) { ... }); // retrieves the next page as PaginatedResult
messagesPage.next == undefined // there are no more pages
});
channel.presence.get(function(presencePage) { // PaginatedResult
presencePage.items // array of PresenceMessage
presencePage.items[0].data // payload for first message
presencePage.items.length // number of messages in the current page of members
presencePage.next(function(nextPage) { ... }); // retrieves the next page as PaginatedResult
presencePage.next == undefined // there are no more pages
});
channel.presence.enter('my status', function() {
// now I am entered
});
channel.presence.history(function(messagesPage) { // PaginatedResult
messagesPage.items // array of PresenceMessage
messagesPage.items[0].data // payload for first message
messagesPage.items.length // number of messages in the current page of history
messagesPage.next(function(nextPage) { ... }); // retrieves the next page as PaginatedResult
messagesPage.next == undefined // there are no more pages
});
All examples assume a client and/or channel has been created as follows:
// basic auth with an API key
var client = new Ably.Realtime(<key string>)
// using token auth
var client = new Ably.Realtime(<token string>)
Given:
var channel = client.channels.get('test');
channel.publish('greeting', 'Hello World!')
channel.history(function(messagesPage) {
messagesPage // PaginatedResult
messagesPage.items // array of Message
messagesPage.items[0].data // payload for first message
messagesPage.items.length // number of messages in the current page of history
messagesPage.next(function(nextPage) { ... }); // retrieves the next page as PaginatedResult
messagesPage.next == undefined // there are no more pages
});
channel.presence.get(function(presencePage) { // PaginatedResult
presencePage.items // array of PresenceMessage
presencePage.items[0].data // payload for first message
presencePage.items.length // number of messages in the current page of members
presencePage.next(function(nextPage) { ... }); // retrieves the next page as PaginatedResult
presencePage.next == undefined // there are no more pages
});
channel.presence.history(function(messagesPage) { // PaginatedResult
messagesPage.items // array of PresenceMessage
messagesPage.items[0].data // payload for first message
messagesPage.items.length // number of messages in the current page of history
messagesPage.next(function(nextPage) { ... }); // retrieves the next page as PaginatedResult
messagesPage.next == undefined // there are no more pages
});
client.auth.requestToken(function(err, tokenDetails) {
// tokenDetails is instance of TokenDetails
token_details.token // token string, eg 'xVLyHw.CLchevH3hF....MDh9ZC_Q'
});
var clientUsingToken = new Ably.Rest(token_details.token);
client.auth.createTokenRequest(function(err, tokenRequest) {
tokenRequest.keyName // name of key used to derive token
tokenRequest.clientId // name of a clientId to be bound to the token
tokenRequest.ttl // time to live for token, in ms
tokenRequest.timestamp // timestamp of this request, in ms since epoch
tokenRequest.capability // capability string for this token
tokenRequest.nonce // non-replayable random string
tokenRequest.mac // HMAC of these params, generated with keyValue
client.stats(function(statsPage) { // statsPage as PaginatedResult
statsPage.items // array of Stats
statsPage.next(function(nextPage) { ... }); // retrieves the next page as PaginatedResult
});
client.time(function(time) { ... }); // time is in ms since epoch
To run both the NodeUnit & Karma Browser tests, simply run the following command:
grunt test
Run the NodeUnit test suite
grunt test:nodeunit
Or run just one or more test files
grunt test:nodeunit --test spec/realtime/auth.test.js
Browser tests are run using Karma test runner.
grunt test:karma
Simply open spec/nodeunit.html in your browser to run the test suite with a nice GUI.
Note: If any files have been added or remove, running the task grunt requirejs
will ensure all the necessary RequireJS dependencies are loaded into the browser by updating spec/support/browser_file_list.js
Run the following command to start a local Nodeunit test runner web server
grunt test:webserver
Open your browser to http://localhost:3000. If you are usig a remote browser, refer to https://docs.saucelabs.com/reference/sauce-connect/ for instructions on setting up a local tunnel to your Nodeunit runner web server.
If you would like to run the tests through Karma, then:
Start a Karma server
karma server
You can optionally connect your browser to the server, visit http://localhost:9876/
Click on the Debug button in the top right, and open your browser's debugging console.
Then run the tests against the Karma server. The test:karma:run
command will concatenate the Ably files beforehand so any changes made in the source will be reflected in the test run.
grunt test:karma:run
All tests are run against the sandbox environment by default. However, the following environment variables can be set before running the Karma server to change the environment the tests are run against.
ABLY_ENV
- defaults to sandbox, however this can be set to another known environment such as 'staging'ABLY_REALTIME_HOST
- explicitly tell the client library to use an alternate host for real-time websocket communication.ABLY_REST_HOST
- explicitly tell the client library to use an alternate host for REST communication.ABLY_PORT
- non-TLS port to use for the tests, defaults to 80ABLY_TLS_PORT
- TLS port to use for the tests, defaults to 443Please visit https://support.ably.io/ for access to our knowledgebase and to ask for any assistance.
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)bundle exec rspec
)git push origin my-new-feature
)Copyright (c) 2015 Ably, Licensed under an MIT license. Refer to LICENSE.txt for the license terms.
FAQs
Realtime client library for Ably, the realtime messaging service
The npm package ably receives a total of 19,972 weekly downloads. As such, ably popularity was classified as popular.
We found that ably demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.