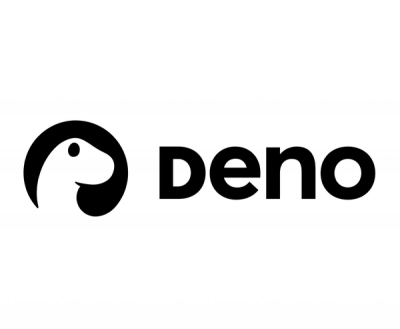
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A simple and type-safe SDK for Aliexpress (system tools, dropshipping and affiliate) APIs.
Aliexpress launched a new open platform to consume their APIs. They fully migrated and upgraded their services from the old Taobao Open Platform to the new Open Platform for International Developers. The issue is, with this update, they have yet to release a Node.js SDK.
This library is an unofficial and simple SDK that can do the following:
# using npm
npm i ae_sdk
# using pnpm
pnpm add ae_sdk
# using yarn
yarn add ae_sdk
To be able to use this SDK, you must first become a developer, follow the steps to register an application, to then retrive your app_key
and app_secret
.
Lastly an access token is required to access the API, follow the steps for the authorization process described in this link. When you get your access token, make sure to pass it the client initialization as session
.
// step 1: import the AE affiliate (`AffiliateClient`) or dropshipping (`DropshipperClient`) client
import { DropshipperClient /* AffiliateClient */ } from "ae_sdk";
// step 2: initilize the client
const client = new DropshipperClient({
app_key: "123",
app_secret: "123456abcdef", // process.env.AE_DS_APP_SECRET
session: "oauth_access_token", // process.env.AE_DS_ACCESS_TOKEN or fetch from DB
});
// step 3: you are all set !
const result = await client.productDetails({
product_id: 1005004043442825,
ship_to_country: "DZ",
target_currency: "USD",
target_language: "en",
});
console.log(result);
If the call was successfull, you will get back the following results.
Inside the
data
object is the API response.
{
ok: true,
data: {
aliexpress_ds_product_get_response: {
result: {...},
rsp_code: 200,
rsp_msg: "Call succeeds",
request_id: "..."
}
}
}
Else if an error occured you will receive the following:
Make sure you to pass the right parameters, but if the problem persits, please contact me
{
ok: false,
message: "..."
}
If you wish to call a different Aliexpress API that is not by the current version. You can do the following:
const result = await client.callAPIDirectly("aliexpress.api.endpoint", {
[string]: string | number | boolean,
});
bytes[]
as a method argument, example: query product using an image.0.4.9
FAQs
A simple SDK for Aliexpress (dropshipping and affiliate) APIs.
The npm package ae_sdk receives a total of 28 weekly downloads. As such, ae_sdk popularity was classified as not popular.
We found that ae_sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.