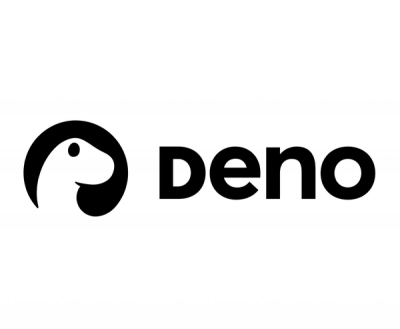
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Abstract library to support a range of styling options for React components.
How to use this library.
The base component, an abstract component with default styles, one which all consumers compose around.
Is usually provided by a third-party library, like Toolkit, or simply defined and consumed directly within an application.
Say we're using a third-party library, like Toolkit, which provides
and styles this reusable Button
component.
function Button({ children, styles }) {
return (
<button className={styles.button}>{children}</button>
);
}
export default style({
button: {
display: 'inline-block',
padding: 5,
},
})(Button);
Now, if I consume this library, how can I customize the styles of this button? What if I want to use class names? Or change the name of existing class names? What about a theme? Etc.
All base components can have their styles overridden by the consumer, like so. This can only be done once in an effort to promote strict isolation and encapsulation. Style overriding can also be disabled all together.
import Button from 'toolkit/components/Button';
Button.setStyles({
button: {
padding: '5px 10px',
fontWeight: 'bold',
},
});
// Or merge with the default styles
Button.mergeStyles({ ... });
And done. We now have a Button
component that is styled to our application.
We can take this a step further, if need be, by wrapping the base component with a custom component.
Say we want to execute some logic, or prepare props, before rendering the base button. We can do this by wrapping the base component with our own component.
import BaseButton from 'toolkit/components/Button';
// Set styles like before
BaseButton.setStyles({ ... });
export default function Button({ children, ...props }) {
// Do something
return (
<BaseButton {...props}>{children}</BaseButton>
);
}
Only adapters that score a star in all categories are supported: https://github.com/MicheleBertoli/css-in-js
style({
foo: 'foo',
bar: 'bar',
})(Component);
.foo {
color: 'red';
display: 'inline';
}
.bar {
color: 'blue';
padding: 5px;
}
import styles from './styles.css';
style(styles)(Component);
style({
foo: {
color: 'red',
display: 'inline',
},
bar: {
color: 'blue',
padding: 5,
},
})(Component);
TODO
FAQs
Aesthetic is a powerful type-safe, framework agnostic, CSS-in-JS library for styling components through the use of adapters.
The npm package aesthetic receives a total of 123 weekly downloads. As such, aesthetic popularity was classified as not popular.
We found that aesthetic demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.