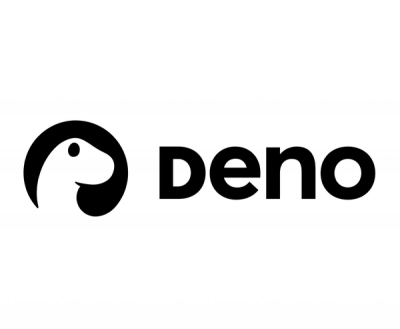
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
afrikit-mobile
Advanced tools
Welcome to Afrikit Mobile! A design system for React Native and Expo projects, providing a cohesive set of UI components and utilities.
Install Afrikit Mobile into your project by running:
npm install afrikit-mobile
# or if you prefer yarn
yarn add afrikit-mobile
This will automatically install all the required dependencies, including Expo, Nativewind, and Tailwind.
Afrikit Mobile exports various ready-to-use components. Here’s an example of using one of them:
// App.tsx
import React from 'react';
import { FloatingButton } from 'afrikit-mobile';
const App = () => {
return (
<FloatingButton onPress={() => console.log('Pressed')} backgroundColor="blue" />
);
};
export default App;
Afrikit Mobile provides multiple components:
import { FloatingButton, IconButton, TopBarTitle } from 'afrikit-mobile';
const App = () => {
return (
<>
<TopBarTitle title="Welcome to Afrikit" />
<FloatingButton onPress={() => console.log('Pressed')} backgroundColor="red" />
<IconButton onPress={() => alert('Icon clicked!')} icon="star" />
</>
);
};
export default App;
Afrikit Mobile integrates seamlessly with Tailwind for styling. You can extend your Tailwind config as needed:
// tailwind.config.js
const afrikitConfig = require('afrikit-mobile/tailwind.config');
module.exports = {
...afrikitConfig,
theme: {
extend: {
colors: {
primary: '#FF6B6B', // Custom color
},
},
},
};
Afrikit also includes custom tokens for colors, spacing, and border radii, which are already added to the theme. Use them like this:
theme: {
colors: {
...afrikitConfig.theme.colors, // Add Afrikit colors
},
spacing: {
...afrikitConfig.theme.spacing, // Add Afrikit spacing tokens
},
borderRadius: {
...afrikitConfig.theme.borderRadius, // Add Afrikit's border radius tokens
},
}
Got ideas for improvements or found a bug? Submit an issue or open a pull request in the GitHub repo.
This project is licensed under the MIT License. See the LICENSE.md file for more details.
Afrikit Mobile is here to save your time, improve your app's UI, and bring consistency to your design workflow. Now, get back to doing what you do best: building awesome apps, and leave the design heavy-lifting to us! 💪
FAQs
Unknown package
The npm package afrikit-mobile receives a total of 12 weekly downloads. As such, afrikit-mobile popularity was classified as not popular.
We found that afrikit-mobile demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.