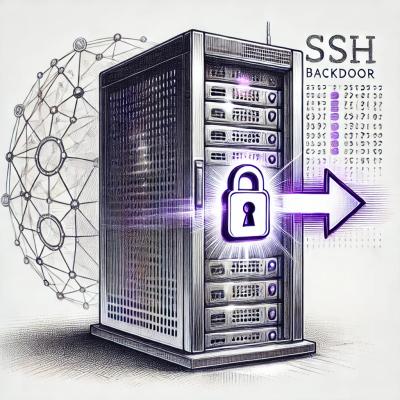
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Automation Framework for Testing (AFT) package supporting JavaScript unit, integration and functional testing
the Automated Functional Testing (AFT) library provides a framework for creating Functional Test Automation that needs to integrate with external systems and can be used for post-deployment verification testing, end-user acceptance testing, end-to-end testing as well as high-level integration testing scenarios. it enables test execution flow control and reporting as well as streamlined test development for JavaScript and TypeScript test automation by integrating with common test framworks as well as external test and defect tracking systems (via a robust plugin structure).
describe('Sample Test', () => {
it('can perform a demonstration of AFT-Core', async () => {
let feature: FeatureObj = new FeatureObj();
/**
* the `should(expectation, options)` function
* checks any specified `ITestCaseHandlerPlugin`
* and `IDefectHandlerPlugin` implementations
* to ensure the test should be run. It will then
* report to any `ILoggingPlugin` implementations
* with a `TestResult` indicating the success,
* failure or skipped status
*/
await should(() => expect(feature.performAction()).toBe('result of action'),
{
testCases: ['C1234'],
description: 'expect that performAction will return \'result of action\''
});
});
});
the above results in the following console output if the expectation does not return false or throw an exception:
5:29:55 PM PASS - [expect_that_performAction_will_return_result_of_action] C1234 Passed -
in more complex scenarios you can perform multiple actions inside the expectation like in the following example:
describe('Sample Test', () => {
it('can perform a more complex demonstration of AFT-Core', async () => {
let feature: FeatureObj = new FeatureObj();
/**
* the passed in expectation can accept a `TestWrapper` which can be used
* during more complex actions
*/
await should(async (tw) => {
await tw.logger.step('about to call performAction');
let result: string = feature.performAction();
await tw.logger.info(`result of performAction was '${result}'`);
expect(result).toBe('result of action');
await tw.logger.trace('successfully executed expectation');
},
{
testCases: ['C2345', 'C3344'],
description: 'more complex expectation actions'
});
});
});
which would output the following logs
5:29:55 PM STEP - [more_complex_expectation_actions] 1: about to call performAction
5:29:55 PM INFO - [more_complex_expectation_actions] result of performAction was 'result of action'
5:29:56 PM TRACE - [more_complex_expectation_actions] successfully executed expectation
5:29:56 PM PASS - [more_complex_expectation_actions] Passed C2345 -
5:29:56 PM PASS - [more_complex_expectation_actions] Passed C3344 -
the AFT-Core package on it's own contains some helpers for testing, but the actual benefit comes from the plugins. Because the above logging will also send to any registered logging plugins, it becomes easy to create loggers that send to any external system such as TestRail or to log results to Elasticsearch. Additionally, before running any expectation passed to a should(expectation, options)
function, AFT will confirm if the expectation should actually be run based on the results of a query to any supplied ITestCaseHandlerPlugin
implementations and a subsequent query to any supplied IDefectHandlerPlugin
implementations.
to create a logging plugin you simply need to implment the ILoggingPlugin
interface in a class with a constructor accepting no arguments. Then, in your aftconfig.json
add the following (where your ILoggingPlugin
implementations are contained in files at ./relative/path/to/logging-plugin1.ts
and /full/path/to/logging-plugin2.ts
):
{
"logging": {
"plugins": [
"./relative/path/to/logging-plugin1",
"/full/path/to/logging-plugin2"
],
"level": "info"
}
}
NOTE: if the plugins are referenced as an external npm packages you may leave off the path and just reference by package name
export class ExternalLogger implements ILoggingPlugin {
name: string = 'externallogger';
async level(): Promise<TestLogLevel> {
let levelStr: string = await TestConfig.getValueOrDefault('external-logging-level', TestLogLevel.warn.name);
return TestLogLevel.parse(levelStr);
}
async enabled(): Promise<boolean> {
let enabledStr: string = await TestConfig.getValueOrDefault('external-logging-enabled', 'false');
return enabledStr.toLocaleLowerCase() == 'true';
}
async log(level: TestLogLevel, message: string): Promise<void> {
/* perform some external logging action */
}
async logResult(result: TestResult): Promise<void> {
/* log the TestResult to an external system */
}
async finalise(): Promise<void> {
/* perform any cleanup */
}
}
the purpose of an ITestCaseHandlerPlugin
is to provide execution control over any expectations by way of supplied Test IDs. to specify an implementation of the plugin to load you can add the following to your aftconfig.json
(where the plugin can be found in a file called plugin.ts
at relative path ./path/to
):
{
"testCaseManager": {
"pluginName": "./path/to/plugin"
}
}
if no plugin is specified then external Test Case Management integration will be disabled and expectations will be executed without checking their status before execution
NOTE: if the plugin is referenced as an external npm package you may leave off the path and just reference by package name
export class MockTestCaseHandlerPlugin implements ITestCaseHandlerPlugin {
name: string = 'testrail-test-case-handler-plugin';
private testRailApi: TestRailClient;
constructor(): void {
this.testRailApi = new TestRailClient();
}
async enabled(): Promise<boolean> {
return true;
}
async getTestCase(testId: string): Promise<ITestCase> {
return await testRailApi.getCaseById(testId);
}
async findTestCases(searchTerm: string): Promise<ITestCase[]> {
return await testRailApi.searchByTerm(searchTerm);
}
async shouldRun(testId: string): Promise<IProcessingResult> {
let c: ITestCase = await this.getTestCase(testId);
if (c.status == TestStatus.Untested) {
return {obj: c, success: true, message: 'test has not yet been run'};
}
return {obj: c, success: false, message: 'test already has a result'};
}
}
the purpose of an IDefectHandlerPlugin
is to provide execution control over any expectations by way of supplied Test IDs referenced in an external ticket tracking system like Bugzilla or Jira. to specify an implementation of the plugin to load you can add the following to your aftconfig.json
(where the plugin can be found in a file called plugin.ts
at relative path ./path/to
):
{
"defectManager": {
"pluginName": "./path/to/plugin"
}
}
if no plugin is specified then external Defect Management integration will be disabled and expectations will be executed without checking their status before execution, however if a Defect Management plugin is specified, the execution of any expectations passed into a should(expectation, options)
function will be halted if any non-closed defects are found when searching for defects that contain reference to the specified Test IDs
NOTE: if the plugin is referenced as an external npm package you may leave off the path and just reference by package name
export class MockDefectHandlerPlugin implements IDefectHandlerPlugin {
name: string = 'bugzilla-defect-handler-plugin';
private bugzillaApi: BugzillaClient;
constructor(): void {
this.bugzillaApi = new BugzillaClient();
}
async enabled(): Promise<boolean> {
return true;
}
async getDefect(defectId: string): Promise<IDefect> {
return await bugzillaApi.getDefectById(defectId);
}
async findDefects(searchTerm: string): Promise<IDefect[]> {
return await bugzillaApi.searchByTerm(searchTerm);
}
}
FAQs
Automation Framework for Testing (AFT) package supporting JavaScript unit, integration and functional testing
The npm package aft-core receives a total of 348 weekly downloads. As such, aft-core popularity was classified as not popular.
We found that aft-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.