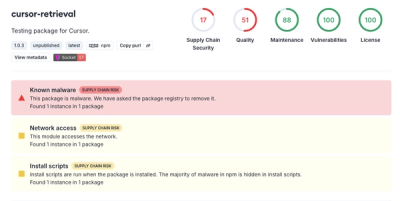
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
This component displays as a tag with an optional link and/or button to remove the given tag.
This component is displayed as a tag with an optional link and/or button to remove it.
Although the ak-tag
component can be used by itself, it works best in conjunction with the
ak-tag-group
component.
Interact with a live demo of the ak-tag component.
npm install ak-tag
The ak-tag
package exports the Tag Skate component. It automatically registers the respective <ak-tag>
web component upon import.
Import the component in your JS resource:
import 'ak-tag';
Now you can use the defined tag in your HTML markup:
<html>
<head>
<script src="bundle.js"></script>
</head>
<body>
<!-- ... -->
<ak-tag text="Jelly bean"></ak-tag>
</body>
</html>
You can also use it within another JS resource:
import Tag from 'ak-tag';
const tag = new Tag();
tag.text = 'Jelly bean';
document.body.appendChild(tag);
This is a standard web component, if you want to use it in your React app, use the Skate.js React integration.
import Tag from 'ak-tag';
import reactify from 'skatejs-react-integration';
const ReactComponent = reactify(Tag, {});
ReactDOM.render(<ReactComponent text="Jelly bean" />, container);
Kind: global class
Emits: beforeRemove
, afterRemove
Properties
string
string
string
Methods
boolean
boolean
Events
Create instances of the component programmatically, or using markup.
HTML Example
<ak-tag text="Cupcake" />
JS Example
import Tag from 'ak-tag';
const tag = new Tag();
tag.text = 'Cupcake';
document.body.appendChild(tag);
string
(Required) The tag text content. This is a required attribute. Omitting it will stop the tag from being rendered. The text passed will be sanitized, e.g. passed HTML will be represented as plain text.
Kind: instance property of Tag
HTML Example
<ak-tag text="Cupcake"></ak-tag>
JS Example
const tag = new Tag();
tag.text = 'Cupcake';
document.body.appendChild(tag); // Shows a tag with the text 'Cupcake'
JS Example
const tag = new Tag();
tag.text = '<script>alert("no no");</script>';
document.body.appendChild(tag); // Shows a tag with the text
// '<script>alert("no no");</script>'
string
(Optional) A target href for the tag text to link to. If this attribute is non-empty, the tag will contain a link to the given URL. The given URL reference will be used as-is and will open in the same window. This attribute implicitly controls isLinked.
Kind: instance property of Tag
HTML Example
<ak-tag text="Cupcake" href="http://www.cupcakeipsum.com/"></ak-tag>
JS Example
const tag = new Tag();
tag.text = 'Cupcake';
tag.href = 'http://www.cupcakeipsum.com/';
document.body.appendChild(tag); // Shows a tag with the text 'Cupcake'
// and a link to cupcakeipsum.com
string
(Optional) The text for the remove button. Implicitly defines that there will be a remove button. This attribute implicitly controls isRemovable.
Kind: instance property of Tag
HTML Example
<ak-tag text="Cupcake" remove-button-text="OMG, I am so full!"></ak-tag>
JS Example
const tag = new Tag();
tag.text = 'Cupcake';
tag.removeButtonText = 'OMG, I am so full!';
document.body.appendChild(tag); // Shows a tag with the text 'Cupcake' and a remove button
boolean
Getter to find out whether the tag is linked. This is implicitly controlled by the href attribute.
Kind: instance method of Tag
Returns: boolean
- Whether the tag is linked or not
JS Example
tag.isLinked(); // Returns true if the tag is linked.
boolean
Getter to find out whether the tag is removable. This is implicitly controlled by the remove-button-text attribute.
Kind: instance method of Tag
Returns: boolean
- Whether the tag is removable or not
JS Example
tag.isRemovable(); // Returns true if the tag is removable.
Allows to programmatically start the tag removal (same as if the user activated the remove button) The removal can be prevented by preventing the Tag#beforeRemove event. The Tag#afterRemove event is fired upon completion. Please note that the tag is not actually removed from the DOM. It is up to the consumer to remove the DOM representation.
Kind: instance method of Tag
Throws:
NotRemovableError
throws an error if invoked on a tag that is not removableEmits: beforeRemove
, afterRemove
JS Example
tag.remove(); // triggers the tag removal
JS Example
import Tag, { events } from 'ak-tag';
const { beforeRemove, afterRemove } = events;
const tag = new Tag();
tag.text = 'Cupcake';
tag.removeButtonText = 'Too much food';
tag.addEventListener(beforeRemove, (e) => {
console.log('Just about to start the remove animation');
// e.preventDefault(); // this would stop the removal process
});
tag.addEventListener(afterRemove, () => {
console.log('Remove animation finished');
document.body.removeChild(tag); // actually remove the DOM representation
});
document.body.appendChild(tag);
JS Example
import Tag, { exceptions } from 'ak-tag';
const { NotRemovableError } = exceptions;
const tag = new Tag();
tag.text = 'Cupcake';
document.body.appendChild(tag);
try {
tag.remove();
} catch(e) {
if (e instanceof NotRemovableError) {
console.error('Could not remove tag');
}
}
This event gets emitted before a tag gets removed (e.g. before the remove animation starts). It is cancelable. If it gets cancelled, the removal is aborted.
Kind: event emitted by Tag
HTML Example
<ak-tag
text="Cupcake"
remove-button-text="No more"
onBeforeRemove={(e) => console.log('Just about to start the remove animation')}
></ak-tag>
JS Example
import { events } from 'ak-tag';
tag.addEventListener(events.beforeRemove, (e) => {
console.log('Just about to start the remove animation');
// e.preventDefault(); // this would stop the removal process
});
This event gets emitted after a tag has been removed (e.g. after the remove animation finished). It is not cancelable.
Kind: event emitted by Tag
HTML Example
<ak-tag
text="Cupcake"
remove-button-text="No more"
onAfterRemove={(e) => console.log('Finished the remove animation')}
></ak-tag>
JS Example
import { events } from 'ak-tag';
tag.addEventListener(events.afterRemove, () => {
console.log('Finished the remove animation');
document.body.removeChild(tag);
});
Kind: global class
Implements: Error
Access: public
This exception gets thrown if a Tag is removed that is not removable.
Let us know what you think of our components and docs, your feedback is really important for us.
Ask a question in our forum.
Check if someone has already asked the same question before.
Are you in trouble? Let us know!
FAQs
This component displays as a tag with an optional link and/or button to remove the given tag.
The npm package ak-tag receives a total of 0 weekly downloads. As such, ak-tag popularity was classified as not popular.
We found that ak-tag demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.