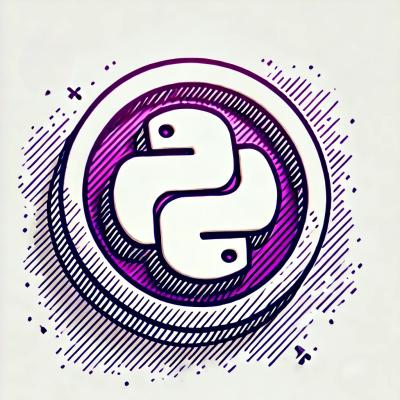
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
alamid-schema
Advanced tools
Extendable mongoose-like schemas for node.js and the browser
If you like mongoose schemas and you want to use them standalone, alamid-schema is the right module for you.
alamid-schema helps you with
Use it on the server to...
Use it on the client to...
var Schema = require("alamid-schema");
var Panda = new Schema("Panda", {
name: String,
age: {
type: Number,
required: true,
writable: false,
readable: true
},
mood: {
type: String,
enum: ["grumpy", "happy"]
},
birthday: Date
});
You can define your schema with concrete values...
var PandaSchema = new Schema({
name: "panda",
age: 12,
friends: {
type: []
}
});
...or with abstract types...
var PandaSchema = new Schema({
name: String,
age: Number,
friends: {
type: Array
}
});
Sometimes you want to extend your Schema and add new properties.
var PandaSchema = new Schema({
name: String,
age: Number,
friends: {
type: Array
}
});
var SuperPanda = PandaSchema.extend("SuperPanda", {
xRay: true,
canFly: {
type: Boolean
}
});
We have a superpanda now... which can fly and has xray eyes! That's basically the same as...
var SuperPanda = new Schema({
name: String,
age: Number,
friends: {
type: Array
},
xRay: true, //added
canFly: { //added
type: Boolean
}
});
Overwriting properties
If you define a property in the schema you are extending with, the extending schema takes precedence.
var Animal = new Schema({
name: String,
age: String
});
var Panda = Animal.extend("Panda", {
age: Number
color: String
});
equals...
var Panda = new Schema("Panda", {
name: String,
age: Number, //overwritten
color: String //added
});
If you need a subset of a schema containing only certain properties, you can use Schema.fields()
.
var Panda = new Schema("Panda", {
name: String,
age: Number,
color: String
});
var SubsetPanda = Panda.fields("name", "age");
equals...
var Panda = new Schema("Panda", {
name: String,
age: Number
});
The validation plugins adds - suprise! - validation support.
var Schema = require("alamid-schema");
Schema.use(require("alamid-schema/plugins/validation"));
var PandaSchema = new Schema({
name: {
type: String,
required: true
},
age: {
type: Number,
min: 9,
max: 99
},
mood: {
type: String,
enum: ["happy", "sleepy"]
},
birthday: Date
});
var panda = {
name: "Hugo",
age: 3,
mood: "happy"
};
PandaSchema.validate(panda, function(validation) {
if(!validation.result) {
console.log(validation);
return;
}
console.log("happy panda");
});
outputs...
{
result: false,
failedFields: {
age: [ 'min' ]
}
}
Included validators:
Writing custom validators:
You can write sync and async validators..
//sync
function oldEnough(age) {
return age > 18 || "too-young";
}
//async
function nameIsUnique(name, callback) {
fs.readFile(__dirname + "/names.json", function(err, names) {
if(err) {
throw err;
}
names = JSON.parse(names);
callback(names.indexOf(name) === -1 || "name-already-taken");
});
}
var PandaSchema = new Schema({
name: {
type: String,
required: true,
validate: nameIsUnique
},
age: {
type: Number,
validate: oldEnough,
max: 99
}
});
var panda = {
name: "hugo",
age: 3,
mood: "happy"
};
PandaSchema.validate(panda, function(validation) {
if(!validation.result) {
console.log(validation.failedFields);
return;
}
console.log("happy panda");
});
outputs...
{
name: [ "name-already-taken" ],
age: [ "too-young" ]
}
Note: validators will be called with this
bound to model
.
Creates a new schema.
Returns a subset with the given keys of the current schema. You may pass an array with keys or just the keys as arguments.
Creates a new schema that inherits from the current schema. Field definitions are merged where appropriate. If a definition conflicts with the parent definition, the child's definition supersedes.
You can define readable and writable fields in the schema. As default every field is read- and writable.
var PandaSchema = new Schema({
id: {
type: Number,
required: true,
readable: true,
writable: false
},
lastModified: {
readable: true,
writable: false
}
};
Validate given model using the schema-definitions.
Callback will be called with a validation object with result
(Boolean) and failedFields
(Object).
FAQs
Extendable mongoose-like schemas for node.js and the browser
The npm package alamid-schema receives a total of 9 weekly downloads. As such, alamid-schema popularity was classified as not popular.
We found that alamid-schema demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.