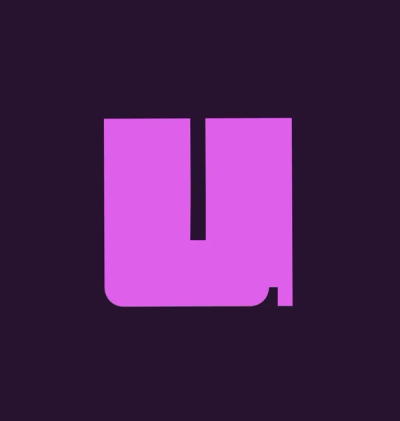
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
allure-playwright
Advanced tools
The allure-playwright package integrates Allure reporting capabilities with Playwright, a popular end-to-end testing framework. It allows you to generate detailed and visually appealing test reports, capturing various aspects of your test execution such as steps, attachments, and test results.
Generate Allure Reports
This feature allows you to generate Allure reports by annotating your Playwright tests with labels and steps. The code sample demonstrates how to add labels and steps to a basic test.
const { test } = require('@playwright/test');
const { allure } = require('allure-playwright');
test('basic test', async ({ page }) => {
allure.label('feature', 'Login');
allure.label('severity', 'critical');
await page.goto('https://example.com');
allure.step('Navigate to example.com');
await page.click('text=Login');
allure.step('Click on Login button');
// Add more steps and assertions
});
Attach Screenshots
This feature allows you to attach screenshots to your Allure reports. The code sample demonstrates how to take a screenshot during a test and attach it to the report.
const { test } = require('@playwright/test');
const { allure } = require('allure-playwright');
test('screenshot test', async ({ page }) => {
await page.goto('https://example.com');
const screenshot = await page.screenshot();
allure.attachment('Screenshot', screenshot, 'image/png');
});
Attach Logs
This feature allows you to attach logs to your Allure reports. The code sample demonstrates how to attach log data to the report.
const { test } = require('@playwright/test');
const { allure } = require('allure-playwright');
test('log test', async ({ page }) => {
await page.goto('https://example.com');
const logs = 'Some log data';
allure.attachment('Logs', logs, 'text/plain');
});
The jest-allure package integrates Allure reporting with Jest, another popular testing framework. It provides similar functionalities such as generating detailed test reports, attaching screenshots, and adding steps. However, it is specifically designed for use with Jest rather than Playwright.
The wdio-allure-reporter package integrates Allure reporting with WebdriverIO, a testing framework for Node.js. It offers similar functionalities such as generating test reports and attaching screenshots, but it is designed for use with WebdriverIO.
Allure framework integration for Playwright Test framework
Use your favorite node package manager to install the package:
npm i -D allure-playwright
Add allure-playwright
as the reporter in the Playwright configuration file:
import { defineConfig } from '@playwright/test';
export default defineConfig({
reporter: "allure-playwright",
});
Or, if you want to use more than one reporter:
import { defineConfig } from '@playwright/test';
export default defineConfig({
reporter: [["line"], ["allure-playwright"]],
});
Or pass the same values via the command line:
npx playwright test --reporter=line,allure-playwright
When the test run completes, the result files will be generated in the ./allure-results
directory. If you want to use another location, provide it via the resultsDir
reporter option (see below).
[!NOTE] You need Allure Report to generate and open the report from the result files. See the installation instructions for more details.
Generate Allure Report:
allure generate ./allure-results -o ./allure-report
Open Allure Report:
allure open ./allure-report
Learn more about Allure Playwright from the official documentation at https://allurereport.org/docs/playwright/.
Use following options to configure Allure Playwright:
Option | Description | Default |
---|---|---|
resultsDir | The path of the results folder. | ./allure-results |
detail | Hide the pw:api and hooks steps in report. | true |
suiteTitle | Use test title instead of allure.suite() . | true |
links | Allure Runtime API link templates. | undefined |
environmentInfo | A set of key-value pairs to display in the Environment section of the report | undefined |
categories | An array of category definitions, each describing a category of defects | undefined |
Here is an example of the reporter configuration:
import { defineConfig } from '@playwright/test';
import os from "node:os";
export default defineConfig({
reporter: [
[
"allure-playwright",
{
detail: true,
resultsDir: "my-allure-results",
suiteTitle: false,
links: {
link: {
urlTemplate: "https://github.com/allure-framework/allure-js/blob/main/%s",
},
issue: {
urlTemplate: "https://github.com/allure-framework/allure-js/issues/%s",
nameTemplate: "ISSUE-%s",
},
},
environmentInfo: {
OS: os.platform(),
Architecture: os.arch(),
NodeVersion: process.version,
},
categories: [
{
name: "Missing file errors",
messageRegex: /^ENOENT: no such file or directory/,
},
],
},
],
],
});
More details about Allure Playwright configuration are available at https://allurereport.org/docs/playwright-configuration/.
FAQs
Allure Playwright integration
We found that allure-playwright demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.