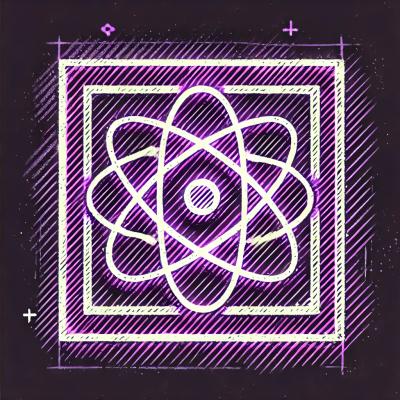
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
amqp-message-bus
Advanced tools
Node.js message bus interface for AMQP servers, such as RabbitMQ.
Node.js message bus interface for AMQP servers, such as RabbitMQ.
$ npm install amqp-message-bus
Create new message bus.
const MessageBus = require('amqp-message-bus');
const bus = new MessageBus({
url: 'amqp://localhost',
encryptionKey: 'keep-it-safe'
});
Connect to AMQP server and subscribe to queue for messages.
await bus.connect();
const unsubscribe = await bus.subscribe('my_queue', (msg, props, done) => {
// process msg + props
console.log(`Received message ${props.messageId} with priority ${props.priority}, published on ${props.timestamp}`);
// call done when ready to remove message from rabbitmq
done();
});
// unsubscribe from queue
await unsubscribe();
// disconnect from bus
await bus.disconnect();
Connect to AMQP server, create queue (if not exists) and send message.
await bus.connect();
await bus.assertQueue('my_queue');
await bus.sendToQueue('my_queue', { foo: 1, bar: 2 });
await bus.disconnect();
Connect to AMQP server, create topic exchange and publish message to route.
await bus.connect();
await bus.assertExchange('my_exchange', 'topic'); // note the 2nd argument (i.e. "topic") used to create a topic exchange
await bus.assertQueue('my_queue');
await bus.bindQueue('my_queue', 'my_exchange', 'route.1'); // note the 3rd argument (i.e. route.1)
await bus.publish('my_exchange', 'route.1', { foo: 1, bar: 2 }); // note the 3rd argument (i.e. route.1)
await bus.disconnect();
Constructs new message bus with the supplied properties.
const bus = new MessageBus({
url: 'amqp://localhost',
encryptionKey: 'keep-it-safe'
});
Connects to AMQP server.
Promise
bus.connect()
.then(() => {
console.log('Connected to rabbitmq');
})
.catch((err) => {
console.error(err);
});
Disconnects from AMQP server.
Promise
bus.disconnect()
.then(() => {
console.log('Disconnected from rabbitmq');
})
.catch((err) => {
console.error(err);
});
Subscribes to the designated queue for incoming messages.
function(msg, props, done)
(required).
Please visit http://www.squaremobius.net/amqp.node/channel_api.html#channel_assertQueue for further info on props
meta-data.
Promise<Function>
The function returned is the unsubscribe()
method.
const listener = (msg, props, done) => {
// do something with msg and props
console.log(JSON.stringify(msg, null, 2));
// call done when you are done with msg to remove from queue
done();
};
bus.subscribe(listener)
.then((unsubscribe) => {
// unsubscribe when ready
return unsubscribe();
})
.catch((err) => {
console.error(err);
});
const unsubscribe = await bus.subscribe((msg, props, done) => {
// do something with msg and props
console.log(JSON.stringify(msg, null, 2));
// call done when you are done with msg to remove from queue
done();
});
// unsubscribe when ready
await unsubscribe();
Sends the supplied message to the designated queue.
UUID v4
)Date.now()
)Promise
bus.sendToQueue('my_queue', {
foo: 'bar'
}, {
type: 'nonsense',
priority: 10
})
.catch((err) => {
console.error(err);
});
await bus.sendToQueue({
foo: 'bar'
}, {
type: 'nonsense',
priority: 10
});
Publishes the supplied message to the designated exchange.
UUID v4
)Date.now()
)Promise
bus.publish('my_exchange', 'route.1', {
foo: 'bar'
}, {
type: 'nonsense',
priority: 10
})
.catch((err) => {
console.error(err);
});
await bus.publish('my_exchange', 'route.1', {
foo: 'bar'
}, {
type: 'nonsense',
priority: 10
});
Unsubscribes the designated consumer.
Promise
bus.unsubscribe('consumer-123')
.catch((err) => {
console.error(err);
});
Unsubscribes all message bus consumers.
Promise
bus.unsubscribeAll()
.catch((err) => {
console.error(err);
});
Source code contributions are most welcome. The following rules apply:
2.1.0 - 2017-11-21
unsubscribeAll()
and deleteExchange()
methods.unsubscribe()
and unsubscribeAll()
in README.md.FAQs
Node.js message bus interface for AMQP servers, such as RabbitMQ.
The npm package amqp-message-bus receives a total of 4 weekly downloads. As such, amqp-message-bus popularity was classified as not popular.
We found that amqp-message-bus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.