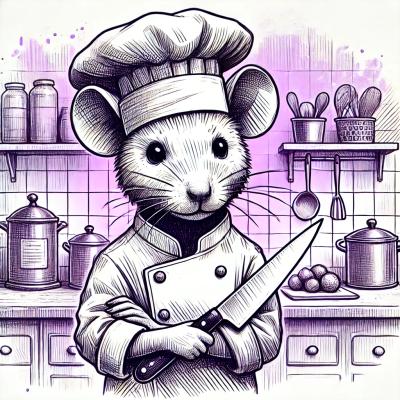
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Filter multiple-streams of incoming objects, and only return objects that are present in all streams.
Node.js Stream to filter multiple-streams of incoming objects, and only return objects that are present in ALL streams.
Install via npm:
$ npm install and-stream
var andStream = require('and-stream');
// No key passed into the factory method, so just uses JSON.stringify for uniqueness
var and = andStream();
and
.on('data', console.log)
.on('end', process.exit);
aStreamOfObjects() // emits objects
.pipe(and.stream());
anotherStreamOfObjects() // emits objects
.pipe(and.stream());
// Only objects that are identical and emitted by both streams will be printed
The and-stream
constructor takes an optional argument which is used to
determine the unique key to identify an object by. If not used, it will
default to using JSON.stringify
to uniquely identify each object, so only
if the exact same object is present on all streams will the objected be
emmitted by the and-stream
.
name
property on the object as the object keyvar andStream = require('and-stream');
// Use the 'name' field as the key for anding object streams
var and = andStream('name');
and
.on('data', console.log)
.on('end', process.exit);
aStreamOfObjects() // emits objects
.pipe(and.stream());
anotherStreamOfObjects() // emits objects
.pipe(and.stream());
// Only objects that have the same .name field on both streams will be printed
var andStream = require('and-stream');
// Use the 'name' field as the key for anding object streams
var and = andStream(function (data) {
var strKey = data.name;
var useKey = new Buffer(strKey, 'utf8').toString('base64');
return useKey;
});
and
.on('data', console.log)
.on('end', process.exit);
aStreamOfObjects() // emits objects
.pipe(and.stream());
anotherStreamOfObjects() // emits objects
.pipe(and.stream());
// Only objects that have the same base64 of the .name field on both streams will be printed
FAQs
Filter multiple-streams of incoming objects, and only return objects that are present in all streams.
The npm package and-stream receives a total of 1 weekly downloads. As such, and-stream popularity was classified as not popular.
We found that and-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.