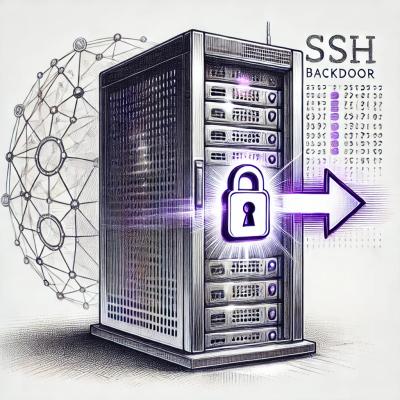
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
angular-local-storage-ci-dev
Advanced tools
An Angular module that gives you access to the browsers local storage
An Angular module that gives you access to the browsers local storage
A forked version for our project CI, add new features such as expiry support for localStorage and so on to meet our demand.
$ npm install angular-local-storage-ci-dev
var angularLocalStorage = require('angular-local-storage-ci-dev');
angular.module('app', [
angularLocalStorage
]);
##Configuration
###setPrefix
You could set a prefix to avoid overwriting any local storage variables from the rest of your app
Default prefix: ls.<your-key>
myApp.config(function (localStorageServiceProvider) {
localStorageServiceProvider
.setPrefix('yourAppName');
});
###setStorageType
You could change web storage type to localStorage or sessionStorage
Default storage: localStorage
myApp.config(function (localStorageServiceProvider) {
localStorageServiceProvider
.setStorageType('sessionStorage');
});
###setStorageCookie
Set cookie options (usually in case of fallback)
cookieOptions: options
cookieOptions.path the web path the cookie represents. default: '/'
myApp.config(function (localStorageServiceProvider) {
localStorageServiceProvider
.setStorageCookie(45, '<path>');
});
###setNotify
Configure whether events should be broadcasted on $rootScope for each of the following actions:
setItem , default: true
, event "LocalStorageModule.notification.setitem"
removeItem , default: false
, event "LocalStorageModule.notification.removeitem"
myApp.config(function (localStorageServiceProvider) {
localStorageServiceProvider
.setNotify(true, true);
});
###Configuration Example Using all together
myApp.config(function (localStorageServiceProvider) {
localStorageServiceProvider
.setPrefix('newci')
.setStorageType('sessionStorage')
.setNotify(true, true)
});
##API Documentation
##isSupported
Checks if the browser support the current storage type(e.g: localStorage
, sessionStorage
).
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
if(localStorageService.isSupported) {
//...
}
//...
});
###getStorageType
Returns: String
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
var storageType = localStorageService.getStorageType(); //e.g localStorage
//...
});
###set
Directly adds a value to local storage.
If local storage is not supported, use cookies instead.
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function submit(key, val) {
// expiry: milliseconds, this value will be expired in `expiry` milliseconds, if not set, and `localStorageService.expiry.alwaysExpire` is true, it will expire in the default expiry time.
// expireTimeStamp: TimeStamp, this value will be expired after this absolute time.
return localStorageService.set(key, val, expiry, expireTimeStamp);
}
//...
});
###get
Directly get a value from local storage.
If local storage is not supported, use cookies instead.
Returns: value from local storage
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function getItem(key) {
// expiry: Number, if this value hasn't been wrapped by us, we will wrap them by using this expiry time.
return localStorageService.get(key, expiry);
}
//...
});
###keys
Return array of keys for local storage, ignore keys that not owned.
Returns: value from local storage
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
var lsKeys = localStorageService.keys();
//...
});
###remove
Remove an item(s) from local storage by key.
If local storage is not supported, use cookies instead.
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function removeItem(key) {
return localStorageService.remove(key);
}
//...
function removeItems(key1, key2, key3) {
return localStorageService.remove(key1, key2, key3);
}
});
###clearAll
Remove all data for this app from local storage.
If local storage is not supported, use cookies instead.
Note: Optionally takes a regular expression string and removes matching.
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function clearNumbers(key) {
return localStorageService.clearAll(/^\d+$/);
}
//...
function clearAll() {
return localStorageService.clearAll();
}
});
###bind
Bind $scope key to localStorageService.
Usage: localStorageService.bind(scope, property, value[optional], key[optional])
key: The corresponding key used in local storage
Returns: deregistration function for this listener.
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
localStorageService.set('property', 'oldValue');
$scope.unbind = localStorageService.bind($scope, 'property');
//Test Changes
$scope.update = function(val) {
$scope.property = val;
$timeout(function() {
alert("localStorage value: " + localStorageService.get('property'));
});
}
//...
});
<div ng-controller="MainCtrl">
<p>{{property}}</p>
<input type="text" ng-model="lsValue"/>
<button ng-click="update(lsValue)">update</button>
<button ng-click="unbind()">unbind</button>
</div>
###deriveKey
Return the derive key
Returns String
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
localStorageService.set('property', 'oldValue');
//Test Result
console.log(localStorageService.deriveKey('property')); // ls.property
//...
});
###length
Return localStorageService.length, ignore keys that not owned.
Returns Number
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
var lsLength = localStorageService.length(); // e.g: 7
//...
});
##Cookie
Deal with browser's cookies directly.
##cookie.isSupported
Checks if cookies are enabled in the browser.
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
if(localStorageService.cookie.isSupported) {
//...
}
//...
});
###cookie.set
Directly adds a value to cookies.
Note: Typically used as a fallback if local storage is not supported.
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function submit(key, val) {
return localStorageService.cookie.set(key, val);
}
//...
});
Cookie Expiry Pass a third argument to specify number of days to expiry
localStorageService.cookie.set(key,val,10)
sets a cookie that expires in 10 days.
###cookie.get
Directly get a value from a cookie.
Returns: value from local storage
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function getItem(key) {
return localStorageService.cookie.get(key);
}
//...
});
###cookie.remove
Remove directly value from a cookie.
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function removeItem(key) {
return localStorageService.cookie.remove(key);
}
//...
});
###cookie.clearAll
Remove all data for this app from cookie.
Returns: Boolean
myApp.controller('MainCtrl', function($scope, localStorageService) {
//...
function clearAll() {
return localStorageService.cookie.clearAll();
}
});
FAQs
An Angular module that gives you access to the browsers local storage
The npm package angular-local-storage-ci-dev receives a total of 0 weekly downloads. As such, angular-local-storage-ci-dev popularity was classified as not popular.
We found that angular-local-storage-ci-dev demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.