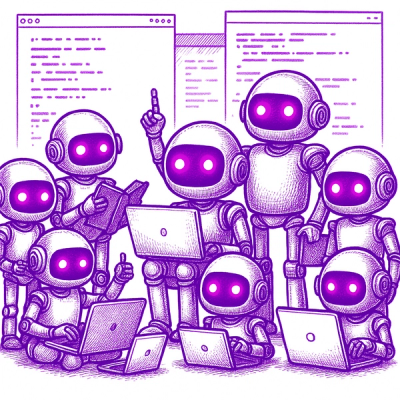
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
angular-observer
Advanced tools
Observe mutations to a scalar, object or array.
Features:
emit(), on()
, one()
, once()
, off()
are all supportedangular
.module('app')
.component('myComponent', {
controller: function($observe) {
// Assign observe to run on each Angular update cycle
this.doCheck = $watch.cycle;
// Watch something
this.myObj = {foo: 'Foo!', bar: 'Bar!', baz: 'Baz!'};
$observe(this, 'myObj')
.on('change', _=> console.log('Object changed in some way'))
.on('key', (key, newVal, oldVal) => console.log('Key', key, 'Changed', oldVal, '=>', newVal))
// Other events: keyDelete, keyAdd
},
});
angular-observer
as a module in your main angular.module()
call.angular-observer.js
file or rolling into your minifier / webpack / concat process of choice.$observe
as a depdenency to any controller you wish to use it in.For a more complex example see the demo directory.
The $observe()
call is compatible with Angulars $watch()
, $watchGroup()
and $watchCollection()
with minimal changes.
Since $observe()
can mix-and-match these approches it is also possible that we can support hybrid observers such as deep, multi-collection watching.
You can use any of the following patterns:
$observe(this, path, callback)
$observe(this, path).on('change', callback)
Deep watching can be acomplished with any of the following:
$observe(this, path, callback, true)
$observe(this, path, {deep: true}).on('change', callback)
$observe.deep(this, path, callback)
$observe.deep(this, path).on('change', callback)
Path can already be an array in a $observe call so any of the usage patterns available with a regular $observe()
call will all work.
Watching a collection with $observe()
is essencially just specifying that the depth = 2
(watch only the immediate array indexes AND the keys of the sub-object).
Any of the following patterns should work:
$observe(this, path, callback, 2)
$observe(this, path, {deep: 2}).on('change', callback)
$observe.deep(this, path, callback, 2)
$observe.deep(this, path, 2).on('change', callback)
The below API repersents the developer-facing functionality. For a full list of functions, methods and variables please read the source code JSDoc comments instead.
The main Observer worker. Calling this function factory with a scope and a path will register an observer worker against it. Any changes will then fire events.
controller: function($observe) {
$observe(this, 'myAmazingObject')
.on('change', _=> console.log('Object changed in some way'))
// Tell Angular to run all checks each digest cycle
$ctrl.$doCheck = $observe.checkAll;
},
See the Events section for what events can be listened for.
This returns an Observable.
Callback is optional, if provided it will be automatically bound with Observable.on('change', CALLBACK)
.
Config is an optional object of options to configure $observe's behaviour. If config
is a number it will be assumed that {deep: CONFIG}
was specified.
Option | Type | Default | Description |
---|---|---|---|
deep | true OR Number | 1 | The maximum depth to iterate when watching a target. If the value is true all levels are examined |
root | true OR String | true | If a string is specified all paths used in event emitters are made relative to the one specified, if true the relative path is calculated from the provided paths only if a single path was specified (this replicates the default behaviour of Angular) |
An alias function for $observeProvider.checkAll()
.
Runs a check on the observe target and fires any events.
You probably don't need to interact with this method directly. Instead use $ctrl.$doCheck = $observe.checkAll
to tell Angular to run all checks on each digest cycle.
An alias function for $observeProvider.checkAll()
.
Fetch the object being observed or a path within it.
Destroy the observer and deregister it with $observeProvider
so it no longer receieves updates.
If called with no path this function returns an array of all modified paths within the object. If given a specific path to examine this function returns a boolean indicating if that path has been modified.
Run a callback on every item within the current object.
The overseeing observer system. This also allows management of any registered observer processes.
Run check()
on all registed $observe
objects. This is also accessable as $observe.checkAll()
.
The following events can be attached to any Observable instance:
Event | Parameters | Description |
---|---|---|
change | (newValue) | Emitted if any part of the observable target changes |
destroy | () | Emitted when the observer is destroyed |
key | (key, newValue) | Emitted if the observable target is an object and any of the top level only key values change |
path | (path, newValue) | Emitted if any deeply nested paths change within the observable |
postChange | (newValue) | Emitted after all other keys have finished before the next injection stage |
postInject | (newValue) | Emitted after the object has been 'sealed' again before the next check cycle |
finally | () | Emitted after all other hooks have been called |
$observe(scope, path, config)
$observe([scope], path, callback)
config.deep = true
$observe(scope, path, [callback], depth)
config.depth = Boolean | Number
Observer.ignore(path...)
FAQs
Observe mutations to a scalar, object or array in Angular
The npm package angular-observer receives a total of 1 weekly downloads. As such, angular-observer popularity was classified as not popular.
We found that angular-observer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.