Angular Star Rating ⭐⭐⭐⭐⭐
⭐ Angular 1.5 Component written in typescript, based on css only techniques. ⭐



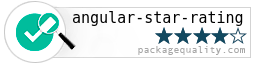
Angular Star Rating is a >1.5 Angular component written in typescript.
It is based on css-sar-rating, a fully featured and customizable css only star rating component written in scss.
DEMO

Features
This module implements all Features from CSS-STAR-RATING.
It also provides callbacks for all calculation functions used in the component as well as all possible event emitters.
Browser support
IE | Firefox | Chrome | Safari | Opera |
---|
11 | 50 | 55 | 10 | 41 |
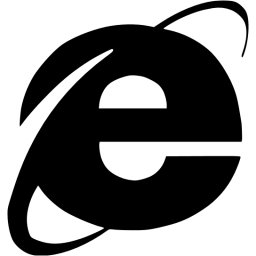 | 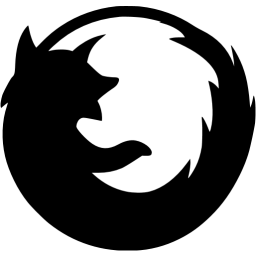 | 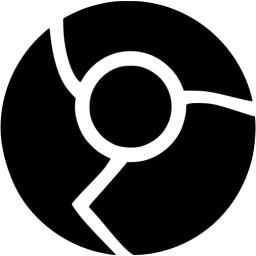 | 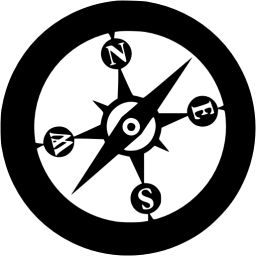 | 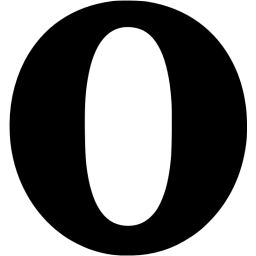 |
Install
Get Angular Star Rating:
- clone & build this repository
- download as .zip
- via npm: by running
$ npm install angular-star-rating
from your console - via bower: by running
$ bower install angular1-star-rating
from your console
Load library
<script src="bower_components/angular-star-rating/dist/index.js"></script>
Inject it into angular
angular.module('myApp', ['star-rating'])
Use it
<star-rating-comp
size="'large'"
rating="3"
text="'Rating:'"
on-update="crtl.onUpdate(rating)">
</star-rating-comp>
Component Properties
@ bindings
id: string (Optional)
The html id attribute of the star rating
Default: undefined
<star-rating-comp id="'my-id'"></star-rating-comp>
< bindings
rating: number (Optional)
The actual star rating value
Default: no
<star-rating-comp rating="3"></star-rating-comp>
showHalfStars: boolean (Optional)
To show half stars or not
Options: true, false
Default: false
<star-rating-comp show-half-stars="true"></star-rating-comp>
numOfStars: number (Optional)
The possible number of stars to choose from
Default: 5
<star-rating-comp num-of-stars="6"></star-rating-comp>
text: string (Optional)
The text next to the stars.
Default: undefined
<star-rating-comp text="'My text!'"></star-rating-comp>
labelPosition: starRatingPosition (Optional)
The position of the label
Options: top, right, bottom, left
Default: left
<star-rating-comp label-position="'top'"></star-rating-comp>
spread: boolean (Optional)
If the start use the whole space or not.
Default: false
<star-rating-comp spread="true"></star-rating-comp>
size: starRatingSizes (Optional)
The height and width of the stars.
Options: small, medium, large
Default: middle
<star-rating-comp size="'small'"></star-rating-comp>
color: starRatingColors (Optional)
Possible color names for the stars.
Options: default, negative, middle, positive
Default: undefined
<star-rating-comp color="'positive'"></star-rating-comp>
disabled: boolean (Optional)
The click callback is disabled, colors are transparent
Default: false
<star-rating-comp disabled="true"></star-rating-comp>
direction: string (Optional)
The direction of the stars and label.
Options: rtl, ltr
Default: rtl
<star-rating-comp direction="'ltr'"></star-rating-comp>
readOnly: boolean (Optional)
The click callback is disabled
Default: false
<star-rating-comp read-only="true"></star-rating-comp>
speed: starRatingSpeed (Optional)
The duration of the animation in ms.
Options: immediately, noticeable, slow
Default: noticeable
<star-rating-comp speed="'slow'"></star-rating-comp>
starType: starRatingStarTypes (Optional)
The type of start resource to use.
Options: svg, icon
Default: svg
<star-rating-comp star-type="'icon'"></star-rating-comp>
getColor: Function (Optional)
Calculation of the color by rating.
Params: rating, number,numOfStars and staticColor
Return: color name
<star-rating-comp get-color="ctrl.getColor(rating, numOfStars, staticColor)"></star-rating-comp>
getHalfStarVisible: Function (Optional)
Calculation for adding the "half" class or not, depending on the rating value.
Params: rating
Return: boolean
<star-rating-comp get-half-star-class="ctrl.getHalfStarClass(rating)" rating="3.2"></star-rating-comp>
& bindings
onClick: Function (Optional)
Callback function for star click event
Params: rating
<star-rating-comp on-click="ctrl.onClick(rating)"></star-rating-comp>
onUpdate: Function (Optional)
Callback function for rating update event
Params: rating
<star-rating-comp on-update="ctrl.onUpdate(rating)"></star-rating-comp>
