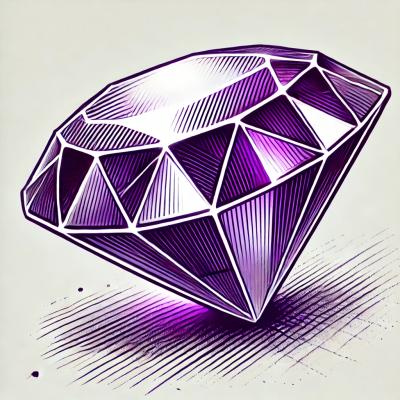
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
angular-star-rating
Advanced tools
Angular Star Rating is a Angular 2+ component made with ❤.
It is based on best practice UX/UI methods, accessibility in mind and an eye for details.
In love with reactive forms the component is easy to control over the keybord.
It is a perfect fit for all angular projects with ⭐'s.
To keep it as flexible as possible a major part of the logic is based on css only techniques. The component simple applies the state depending css modifiers.
IE | Firefox | Chrome | Safari | Opera |
---|---|---|---|---|
>11 | >50 | >55 | >10 | >41 |
![]() | ![]() | ![]() | ![]() | ![]() |
Fully featured this component is provided with:
Css | Angular 1 | Angular | Ionic 1 |
---|---|---|---|
![]() | ![]() | ![]() | ![]() |
Css Star Rating | Angular1 Star Rating | Angular Star Rating | Ionic1 Star Rating |
Get Angular Star Rating:
$ npm install angular-star-rating --save
from your consoleAs a precondition we consider you have your .angular-cli.json
setup with an assets folder so that the ./src/assets
folder is included in the bundled version.
But this is given by default if you use the angular-cli to setup your project.
Now you can import your library in your angular application by running:
$ npm install angular-star-rating --save
or
$ npm install angular-star-rating@2.0.0-rc.5 --save
for a specific version.
Create an assets
folder under your ./src
folder and copy the images from your ./node_modules/assets/images
folder into ./src/assets/images
.
In your app.module.ts
import the StarRatingModule
to your Angular AppModule
:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
// IMPORT YOUR LIBRARY
import { StarRatingModule } from 'angular-star-rating';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
// SPECIFY YOUR LIBRARY AS AN IMPORT
StarRatingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Once your library is imported, you can use the components in your Angular application:
<!-- You can now use your library component in app.component.html -->
<h1>
{{title}}
</h1>
<!-- USE COMPONENT HERE-->
<star-rating-comp [starType]="'svg'" [rating]="2.63"></star-rating-comp>
If something is not working check if
star-rating.icons.svg
is present in your ./src/assets/images
and loadedimport {Component} from "@angular/core";
import {IStarRatingOnClickEvent, IStarRatingOnRatingChangeEven, IStarRatingIOnHoverRatingChangeEvent} from "angular-star-rating/src/star-rating-struct";
@Component({
selector: 'my-events-component',
template: `
<star-rating-comp [starType]="'svg'"
[hoverEnabled]="true"
(onClick)="onClick($event)"
(onRatingChange)="onRatingChange($event)"
(onHoverRatingChange)="onHoverRatingChange($event)">
</star-rating-comp>
<p>onHoverRatingChangeResult: {{onHoverRatingChangeResult | json}}</p>
<p>onClickResult: {{onClickResult | json}}</p>
<p>onRatingChangeResult: {{onRatingChangeResult | json}}</p>
`
})
export class MyEventsComponent {
onClickResult:IStarRatingOnClickEvent;
onHoverRatingChangeResult:IStarRatingIOnHoverRatingChangeEvent;
onRatingChangeResult:IStarRatingOnRatingChangeEven;
onClick = ($event:IStarRatingOnClickEvent) => {
console.log('onClick $event: ', $event);
this.onClickResult = $event;
};
onRatingChange = ($event:IStarRatingOnRatingChangeEven) => {
console.log('onRatingUpdated $event: ', $event);
this.onRatingChangeResult = $event;
};
onHoverRatingChange = ($event:IStarRatingIOnHoverRatingChangeEvent) => {
console.log('onHoverRatingChange $event: ', $event);
this.onHoverRatingChangeResult = $event;
};
}
<!-- app.component.html-->
<my-event-component></my-event-component>
As easy as it could be. Just apply the formControlName
attribute to the star rating component.
//my.component.ts
import {Component} from "@angular/core";
import {FormGroup, FormControl} from "@angular/forms";
@Component({
selector: 'my-form-component',
template: `
<form [formGroup]="form">
<star-rating-comp [starType]="'svg'" formControlName="myRatingControl" ></star-rating-comp>
<pre>{{ form.value | json }}</pre>
</form>
`
})
export class MyFormComponent {
form = new FormGroup({
myRatingControl: new FormControl('')
});
}
<!-- app.component.html-->
<my-form-component></my-form-component>
labelVisible: string (Optional)
The visibility of the label text.
Default: true
<star-rating-comp [labelVisible]="false"></star-rating-comp>
labelText: string (Optional)
The text next to the stars.
Default: undefined
<star-rating-comp [labelText]="'My text!'"></star-rating-comp>
labelPosition: starRatingPosition (Optional)
The position of the label
Options: top, right, bottom, left
Default: left
<star-rating-comp [labelPosition]="'top'"></star-rating-comp>
starType: starRatingStarTypes (Optional)
The type of start resource to use.
Options: svg, icon
Default: svg
<star-rating-comp [starType]="'icon'"></star-rating-comp>
staticColor: starRatingColor (Optional)
Possible color names for the stars.
Options: default, negative, ok, positive
Default: undefined
<star-rating-comp [staticColor]="'positive'"></star-rating-comp>
size: starRatingSizes (Optional)
The height and width of the stars.
Options: small, medium, large
Default: medium
<star-rating-comp [size]="'small'"></star-rating-comp>
space: starRatingStarSpace (Optional)
If the start use the whole space or not.
Options: no, between, around
Default: no
<star-rating-comp [space]="'around'"></star-rating-comp>
speed: starRatingSpeed (Optional)
The duration of the animation in ms.
Options: immediately, noticeable, slow
Default: noticeable
<star-rating-comp [speed]="'slow'"></star-rating-comp>
direction: string (Optional)
The direction of the stars and label.
Options: rtl, ltr
Default: rtl
<star-rating-comp [direction]="'ltr'"></star-rating-comp>
disabled: boolean (Optional)
The click callback is disabled, colors are transparent
Default: false
<star-rating-comp [disabled]="true"></star-rating-comp>
rating: number (Optional)
The actual star rating value
Default: no
<star-rating-comp [rating]="3"></star-rating-comp>
step: number (Optional)
The step interval of the control
Default: 1
<star-rating-comp [step]="0.5"></star-rating-comp>
numOfStars: number (Optional)
The possible number of stars to choose from
Default: 5
<star-rating-comp [numOfStars]="6"></star-rating-comp>
showHalfStars: boolean (Optional)
To show half stars or not
Options: true, false
Default: false
<star-rating-comp [showHalfStars]="true"></star-rating-comp>
readOnly: boolean (Optional)
The click callback is disabled
Default: false
<star-rating-comp [readOnly]="true"></star-rating-comp>
id: string (Optional)
The html id attribute of the star rating
Default: undefined
<star-rating-comp [id]="'my-id'"></star-rating-comp>
getColor: Function (Optional)
Calculation of the color by rating.
Params: rating, number,numOfStars and staticColor
Return: color name
<star-rating-comp [getColor]="ctrl.getColor(rating, numOfStars, staticColor)"></star-rating-comp>
getHalfStarVisible: Function (Optional)
Calculation for adding the "half" class or not, depending on the rating value.
Params: rating
Return: boolean
<star-rating-comp [getHalfStarClass]="ctrl.getHalfStarClass(rating)" [rating]="3.2"></star-rating-comp>
onClick: Function (Optional)
Callback function for star click event
Params: $event
<star-rating-comp (onClick)="ctrl.onClick($event)"></star-rating-comp>
onRatingChange: Function (Optional)
Callback function for rating change event
Params: $event
<star-rating-comp (onRatingChange)="ctrl.onRatingChange($event)"></star-rating-comp>
hoverEnabled: boolean (Optional)
An or disable hover interaction.
Default: false
Potions: true, false
<star-rating-comp [hoverEnabled]="true"></star-rating-comp>
onHoverRatingChange: Function (Optional)
Callback function for hoverRating change event
Params: $event
<star-rating-comp [hoverEnabled]="true" (onHoverRatingChange)="ctrl.onHoverRatingChange($event)"></star-rating-comp>
MIT © Michael Hladky
FAQs
Angular Star Rating is a fully featured and configurable Angular UI component.
The npm package angular-star-rating receives a total of 1,329 weekly downloads. As such, angular-star-rating popularity was classified as popular.
We found that angular-star-rating demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.