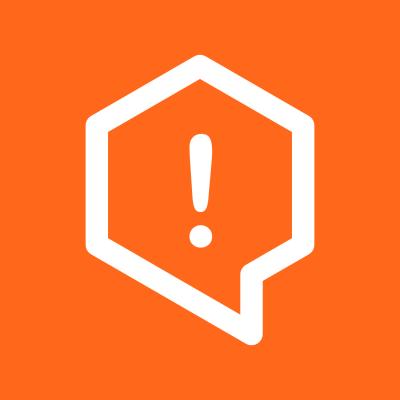
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
angular2-pipes
Advanced tools
Useful pipes for Angular2.
$ npm install ng2-pipes --save
imports
the NgPipesModule
in order to add all of the pipes, Or add a specific module such as NgArrayPipesModule
, NgStringPipesModule
, NgMathPipesModule
or NgBooleanPipesModule
.import {NgPipesModule} from 'ng2-pipes';
@NgModule({
// ...
imports: [
// ...
NgPipesModule
]
})
@Component({
// ..
providers: [ReversePipe]
})
export class AppComponent {
constructor(private reversePipe: ReversePipe) {
this.reversePipe.transform('foo'); // Returns: "oof"
}
// ..
}
Repeats a string n times
API: string | repeat: times: [separator|optional]
<p>{{ 'example' | repeat: 3: '@' }}</p> <!-- Output: "example@example@example" -->
Scans string and replace {i}
placeholders by equivalent member of the array
API: string | scan: [ARRAY]
<p>{{'Hey {0}, {1}' | scan: ['foo', 'bar']}}</p> <!-- Output: "Hey foo, bar" -->
Shortening a string by length and providing a custom string to denote an omission
API: string | shorten: length: [suffix|optional]: [wordBreak boolean|optional]
<p>{{'Hey foo bar' | shorten: 3: '...'}}</p> <!-- Output: "Hey..." -->
Strips a HTML tags from string and providing which tags should not be removed
API: string | stripTags: [ARRAY]
<p>{{'<a href="">foo</a> <p class="foo">bar</p>' | stripTags }}</p> <!-- Output: "foo bar" -->
<p>{{'<a href="">foo</a> <p class="foo">bar</p>' | stripTags: 'p'}}</p> <!-- Output: foo <p class="foo">bar</p> -->
Uppercase first letter of first word
<p>{{'foo bar' | ucfirst }}</p> <!-- Output: "Foo bar" -->
Uppercase first letter every word
<p>{{'foo bar' | ucwords }}</p> <!-- Output: "Foo Bar" -->
Strips characters from the beginning and end of a string (default character is space).
API: string | trim: [characters|optional]
<p>{{' foo ' | trim }}</p> <!-- Output: "foo" -->
<p>{{'foobarfoo' | trim: 'foo' }}</p> <!-- Output: "bar" -->
Strips characters from the beginning of a string (default character is space).
API: string | ltrim: [characters|optional]
<p>{{' foo ' | ltrim }}</p> <!-- Output: "foo " -->
<p>{{'foobarfoo' | ltrim: 'foo' }}</p> <!-- Output: "barfoo" -->
Strips characters from the end of a string (default character is space).
API: string | rtrim: [characters|optional]
<p>{{' foo ' | rtrim }}</p> <!-- Output: " foo" -->
<p>{{'foobarfoo' | rtrim: 'foo' }}</p> <!-- Output: "foobar" -->
Reverses a string
API: string | reverse
<p>{{'foo bar' | reverse }}</p> <!-- Output: "rab oof" -->
Returns array of diff between arrays
API: array | diff: [ARRAY]: [ARRAY]: ... : [ARRAY]
this.items = [1, 2, 3, 4];
<li *ngFor="let item of items | diff: [1, 2]"> <!-- Array: [3, 4] -->
Flattens nested array, passing shallow will mean it will only be flattened a single level
API: array | flatten: [shallow|optional]
this.items = [1,2,3,[4,5,6,[7,8,9],[10,11,12,13,[14],[15],[16, [17]]]]];
<li *ngFor="let item of items | flatten">
<!-- Array: [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17] -->
Slicing off the end of the array by n elements
API: array | initial: n
this.items = [first, second, third];
<li *ngFor="let item of items | initial: 1"> <!-- Array: [first, second] -->
Slicing off the start of the array by n elements
API: array | tail: n
this.items = [first, second, third];
<li *ngFor="let item of items | tail: 1"> <!-- Array: [second, third] -->
Returns the intersections of an arrays
API: array | intersection: [ARRAY]: [ARRAY]: ... : [ARRAY]
this.items = [1, 2, 3, 4, 5];
<li *ngFor="let item of items | intersection: [1, 2, 3]: [3, 6]"> <!-- Array: [3] -->
Reverses an array
API: array | reverse
this.items = [1, 2, 3];
<li *ngFor="let item of items | reverse"> <!-- Array: [3, 2, 1] -->
Removes un-truthy values from array
API: array | truthify
this.items = [null, 1, false, undefined, 2, 0, 3, NaN, 4, ''];
<li *ngFor="let item of items | truthify"> <!-- Array: [1, 2, 3, 4] -->
Removes un-truthy values from array
API: array | union: [ARRAY]
this.items = [1, 2, 3];
<li *ngFor="let item of items | union: [[4]]"> <!-- Array: [1, 2, 3, 4] -->
Removes duplicates from array
API: array | unique
this.items = [1, 2, 3, 1, 2, 3];
<li *ngFor="let item of items | unique"> <!-- Array: [1, 2, 3] -->
Returns array without specific elements
API: array | without: [ARRAY]
this.items = [1, 2, 3, 1, 2, 3];
<li *ngFor="let item of items | without: [1,3]"> <!-- Array: [2, 2] -->
Returns array of properties values
API: array | pluck: propertyName
this.items = [
{
a: 1,
b: {
c: 4
}
},
{
a: 2,
b: {
c: 5
}
},
{
a: 3,
b: {
c: 6
}
},
];
<li *ngFor="let item of items | pluck: 'a'"> <!-- Array: [1, 2, 3] -->
<li *ngFor="let item of items | pluck: 'b.c'"> <!-- Array: [4, 5, 6] -->
Returns randomly shuffled array
API: array | shuffle
this.items = [1, 2, 3, 4, 5, 6];
<li *ngFor="let item of items | shuffle"> <!-- Array: [4, 1, 6, 2, 5, 3] -->
Returns true if every elements of the array fits the predicate otherwise false
API: array | every: predicate
this.itemsOne = [1, 1, 1];
this.itemsTwo = [1, 1, 2];
this.itemsThree = [2, 2, 2];
this.predicate = (value: any, index: number, array: any[]): boolean => {
return value === 1;
};
<p>{{ itemsOne | every: predicate }}</p> <!-- Output: "true" -->
<p>{{ itemsTwo | every: predicate }}</p> <!-- Output: "false" -->
<p>{{ itemsThree | every: predicate }}</p> <!-- Output: "false" -->
Returns true if some elements of the array fits the predicate otherwise false
API: array | some: predicate
this.itemsOne = [1, 1, 1];
this.itemsTwo = [1, 1, 2];
this.itemsThree = [2, 2, 2];
this.predicate = (value: any, index: number, array: any[]): boolean => {
return value === 1;
};
<p>{{ itemsOne | some: predicate }}</p> <!-- Output: "true" -->
<p>{{ itemsTwo | some: predicate }}</p> <!-- Output: "true" -->
<p>{{ itemsThree | some: predicate }}</p> <!-- Output: "false" -->
Returns sample items randomly from array
API: array | sample: [amount | default = 1]
<p>{{ [1, 2, 3, 4] | sample }}</p> <!-- Output: "[2]" -->
<p>{{ [1, 2, 3, 4] | sample: 2 }}</p> <!-- Output: "[4, 3]" -->
Returns the minimum of a given array
API: array | min
<p>{{ [1, 2, 3, 1, 2, 3] | min }}</p> <!-- Output: "1" -->
Returns the maximum of a given array
API: array | max
<p>{{ [1, 2, 3, 1, 2, 3] | max }}</p> <!-- Output: "3" -->
Returns the sum of a given array
API: array | sum
<p>{{ [1, 2, 3, 4] | sum }}</p> <!-- Output: "10" -->
Returns percentage between numbers
API: number | percentage: [total | default = 100]: [floor | default = false]
<p>{{ 5 | percentage }}</p> <!-- Output: "5" -->
<p>{{ 5 | percentage: 160 }}</p> <!-- Output: "3.125" -->
<p>{{ 5 | percentage: 160: true }}</p> <!-- Output: "3" -->
Returns ceil of a number by precision
API: number | ceil: [precision | default = 0]
<p>{{ 42.123 | ceil }}</p> <!-- Output: "43" -->
<p>{{ 42.123 | ceil: 2 }}</p> <!-- Output: "42.13" -->
Returns floor of a number by precision
API: number | floor: [precision | default = 0]
<p>{{ 42.123 | floor }}</p> <!-- Output: "42" -->
<p>{{ 42.123 | floor: 2 }}</p> <!-- Output: "42.12" -->
Returns round of a number by precision
API: number | round: [precision | default = 0]
<p>{{ 42.4 | round }}</p> <!-- Output: "42" -->
<p>{{ 42.5 | round }}</p> <!-- Output: "43" -->
<p>{{ 42.123 | round: 2 }}</p> <!-- Output: "42.12" -->
Returns the square root of a number
API: number | sqrt
<p>{{ 9 | sqrt }}</p> <!-- Output: "3" -->
Returns the power of a number
API: number | pow: [power | default = 2]
<p>{{ 3 | pow }}</p> <!-- Output: "9" -->
<p>{{ 3 | pow: 3 }}</p> <!-- Output: "27" -->
Returns the degrees of a number in radians
API: number | degrees
<p>{{ 3.141592653589793 | degrees }}</p> <!-- Output: "180" -->
Returns the radians of a number in degrees
API: number | radians
<p>{{ 180 | radians }}</p> <!-- Output: "3.141592653589793" -->
Returns bytes with a unit symbol
API: number | bytes
<p>{{ 10 | bytes }}</p> <!-- Output: "10 B" -->
<p>{{ 1000 | bytes }}</p> <!-- Output: "1 KB" -->
<p>{{ 1000000 | bytes }}</p> <!-- Output: "1 MB" -->
<p>{{ 1000000000 | bytes }}</p> <!-- Output: "1 GB" -->
API: any | isNull
<p>{{ null | isNull }}</p> <!-- Output: "true" -->
<p>{{ 1 | isNull }}</p> <!-- Output: "false" -->
API: any | isDefined
<p>{{ 1 | isDefined }}</p> <!-- Output: "true" -->
<p>{{ undefined | isDefined }}</p> <!-- Output: "false" -->
API: any | isUndefined
<p>{{ 1 | isUndefined }}</p> <!-- Output: "false" -->
<p>{{ undefined | isUndefined }}</p> <!-- Output: "true" -->
API: any | isString
<p>{{ 1 | isString }}</p> <!-- Output: "false" -->
<p>{{ '' | isString }}</p> <!-- Output: "true" -->
API: any | isNumber
this.func = () => {};
this.num = 1;
<p>{{ num | isNumber }}</p> <!-- Output: "true" -->
<p>{{ func | isNumber }}</p> <!-- Output: "false" -->
API: any | isArray
this.arr = [1, 2];
this.num = 1;
<p>{{ num | isArray }}</p> <!-- Output: "false" -->
<p>{{ arr | isArray }}</p> <!-- Output: "true" -->
API: any | isObject
this.obj = {a: 1, b: 2};
this.num = 1;
<p>{{ num | isObject }}</p> <!-- Output: "false" -->
<p>{{ obj | isObject }}</p> <!-- Output: "true" -->
API: number | isGreaterThan: otherNumber
<p>{{ 1 | isGreaterThan: 1 }}</p> <!-- Output: "false" -->
<p>{{ 1 | isGreaterThan: 2 }}</p> <!-- Output: "false" -->
<p>{{ 2 | isGreaterThan: 1 }}</p> <!-- Output: "true" -->
API: number | isGreaterEqualThan: otherNumber
<p>{{ 1 | isGreaterEqualThan: 1 }}</p> <!-- Output: "true" -->
<p>{{ 1 | isGreaterEqualThan: 2 }}</p> <!-- Output: "false" -->
<p>{{ 2 | isGreaterEqualThan: 1 }}</p> <!-- Output: "true" -->
API: number | isLessThan: otherNumber
<p>{{ 1 | isLessThan: 1 }}</p> <!-- Output: "false" -->
<p>{{ 1 | isLessThan: 2 }}</p> <!-- Output: "true" -->
<p>{{ 2 | isLessThan: 1 }}</p> <!-- Output: "false" -->
API: number | isLessEqualThan: otherNumber
<p>{{ 1 | isLessEqualThan: 1 }}</p> <!-- Output: "true" -->
<p>{{ 1 | isLessEqualThan: 2 }}</p> <!-- Output: "true" -->
<p>{{ 2 | isLessEqualThan: 1 }}</p> <!-- Output: "false" -->
API: number | isEqualTo: otherNumber
<p>{{ 1 | isEqualTo: 1 }}</p> <!-- Output: "true" -->
<p>{{ 1 | isEqualTo: '1' }}</p> <!-- Output: "true" -->
<p>{{ 1 | isEqualTo: 2 }}</p> <!-- Output: "false" -->
<p>{{ 2 | isEqualTo: 1 }}</p> <!-- Output: "false" -->
API: number | isNotEqualTo: otherNumber
<p>{{ 1 | isNotEqualTo: 1 }}</p> <!-- Output: "false" -->
<p>{{ 1 | isNotEqualTo: '1' }}</p> <!-- Output: "false" -->
<p>{{ 1 | isNotEqualTo: 2 }}</p> <!-- Output: "true" -->
<p>{{ 2 | isNotEqualTo: 1 }}</p> <!-- Output: "true" -->
API: number | isIdenticalTo: otherNumber
<p>{{ 1 | isIdenticalTo: 1 }}</p> <!-- Output: "true" -->
<p>{{ 1 | isIdenticalTo: '1' }}</p> <!-- Output: "false" -->
<p>{{ 1 | isIdenticalTo: 2 }}</p> <!-- Output: "false" -->
<p>{{ 2 | isIdenticalTo: 1 }}</p> <!-- Output: "false" -->
API: number | isNotIdenticalTo: otherNumber
<p>{{ 1 | isNotIdenticalTo: 1 }}</p> <!-- Output: "false" -->
<p>{{ 1 | isNotIdenticalTo: '1' }}</p> <!-- Output: "true" -->
<p>{{ 1 | isNotIdenticalTo: 2 }}</p> <!-- Output: "true" -->
<p>{{ 2 | isNotIdenticalTo: 1 }}</p> <!-- Output: "true" -->
FAQs
Useful angular2 pipes
The npm package angular2-pipes receives a total of 80 weekly downloads. As such, angular2-pipes popularity was classified as not popular.
We found that angular2-pipes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.