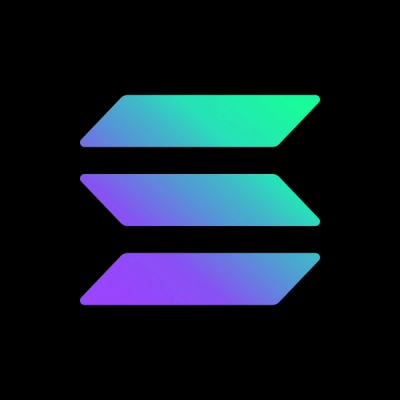
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
angular2-redux
Advanced tools
Wrapper components for using Redux in an Angular2 application.
A full Angular 2 Redux demo can be found here: https://github.com/InfomediaLtd/angular2-redux-example
This library includes a wrapper for the redux store. Create the wrapper AppStore by passing in the Redux appStore:
new AppStore(reduxAppStore);
All redux's store methods are supported, with the additional benefit of getting the state passed into subscribers. Here is an example of a sample component using dependency injection to get the store, subscribe to it and clean up the subscription when it is destroyed:
export class SomeComponent implements OnDestroy {
private unsubscribe:()=>void;
constructor(appStore:AppStore) {
this.unsubscribe = appStore.subscribe(state => {
// do something with state
});
}
public ngOnDestroy() {
// unsubscribe when the component is destroyed
this.unsubscribe();
}
}
Creating the app store in a factory is recommended. This will support redux (and the redux dev tools) inside Angular2's zone and means that the app store will only be created after angular is bootstrapped:
import {AppStore} from "angular2-redux";
import {bootstrap} from "angular2/platform/browser";
// create factory to be called once angular has been bootstrapped
const appStoreFactory = () => {
let reduxAppStore;
// create redux store
// ...
return new AppStore(reduxStore);
};
// bootstrap angular
bootstrap(MyAppComponent,[provide(AppStore, { useFactory: appStoreFactory })]);
Another option is to use the provided factory, by passing in your reducers (one or more) and optional middlewares. This factory supports the thunk middleware and the redux dev tools Chrome extension out of the box. To turn on the dev tools pass the debug param in the URL query string (http://...?debug=true)
Simple creation with a single reducer:
import {AppStore,createAppStoreFactory} from "angular2-redux";
//...
// create app store factory
const appStoreFactory = createAppStoreFactory(counterReducer);
bootstrap(MyAppComponent,[provide(AppStore, { useFactory: appStoreFactory })]);
Creation with more options: multiple reducers, additional middleware, debugging
import {AppStore,createAppStoreFactoryWithOptions} from "angular2-redux";
//...
// my logger middleware
const loggerMiddleware = store => next => action => {
console.log('dispatching', action);
return next(action);
}
// create app store factory
const appStoreFactory = createAppStoreFactoryWithOptions({
reducers:{reducer1,reducer2},
additionalMiddlewares:[loggerMiddleware],
debug:true // accepts a function as well
});
bootstrap(MyAppComponent,[provide(AppStore, { useFactory: appStoreFactory })]);
The Actions is intended to be subclassed by action creators. It lets you bind the app store dispatch method to any action. You can use this helper function to avoid having a local property for the app store and the actions inside your Angular components.
Assuming MyActions class extends Actions you can use it like this:
@Component({
selector: 'my-component',
template: '<button (click)="someAction()">Decrement</a>'
})
export class MyComponent {
private someAction;
constructor(myActions:MyActions) {
this.someAction = myActions.createDispatcher(myActions.someAction);
}
}
If you want your actions pure or decoupled from the app store (in case you're using multiple app stores), you can pass the app store in the createDispatcher function:
constructor(myActions:MyActions, appStore:AppStore) {
this.someAction = myActions.createDispatcher(myActions.someAction, appStore);
}
### Usage example
A usage example is included in this project here: [https://github.com/InfomediaLtd/angular2-redux/tree/master/app](https://github.com/InfomediaLtd/angular2-redux/tree/master/app)
To run the included example you need [jspm](http://jspm.io/) and [live-server](https://www.npmjs.com/package/live-server):
```sh
jspm install
live-server
When updating code please follow these commands for checking in
git add *
npm run commit
git push origin master
FAQs
This project was generated with [Angular CLI](https://github.com/angular/angular-cli) version 6.0.1.
The npm package angular2-redux receives a total of 2 weekly downloads. As such, angular2-redux popularity was classified as not popular.
We found that angular2-redux demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.