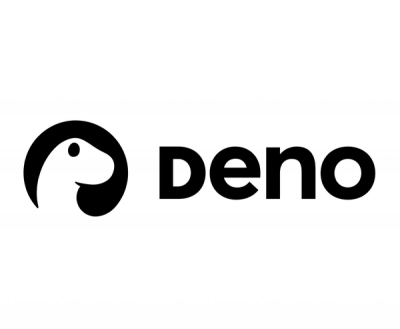
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
angular2-seed-advanced
Advanced tools
High-quality, modular starter (advanced seed) project for Angular 2 apps with statically typed build and AoT + NativeScript + Electron
This is an advanced seed project for Angular 2 apps based on Minko Gechev's angular-seed that expands on all of its great features to include core support for:
write_key
hereThe zen of multiple platforms. Chrome, Android and iPhone all running the same code. |
Programming Nirvana. Mac and Windows desktop both running the same code. |
frameworks
:
core
: foundation layer (decorators and low-level services)analytics
: analytics provided by Segment
i18n
: internationalization featureselectron
: Electron specific codesample
: Just a sample module providing some components and servicestest
: test specific code providing conveniences to make testing your code easier and fastertslint
options like "no-unused-variable": true
as the api hangs off a plain Object
instead of globals
iit
or ddescribe
but didn't import those or vice versa, used iit
leaving an unused it
now in your tests? yeah, tslint
will be all over you :/unused
variable warnings altogether in tests since you are always using a valid key from the shorthand Object
Advice: If your project is intended to target a single platform (i.e, web only), then angular-seed is likely more than suitable for your needs. However if your project goals are to target multiple platforms (web, native mobile and native desktop), with powerful out of the box library support and highly configurable/flexible testing options, then you might want to keep reading.
Additionally, this seed is intended to push a couple boundaries so if you see dependencies that are bleeding edge, this is intentional.
node v5.x.x or higher and npm 3 or higher.
To run the NativeScript app:
npm install -g nativescript
npm install -g typescript
git clone --depth 1 https://github.com/NathanWalker/angular-seed-advanced.git
cd angular-seed-advanced
# install the project's dependencies
$ npm install
# fast install (via Yarn, https://yarnpkg.com)
$ yarn install # or yarn
# watches your files and uses livereload by default
$ npm start
# api document for the app
# npm run build.docs
# to start deving with livereload site and coverage as well as continuous testing
$ npm run start.deving
# dev build
$ npm run build.dev
# prod build
$ npm run build.prod
Note that AoT compilation requires node v6.5.0 or higher and npm 3.10.3 or higher.
In order to start the seed with AoT use:
# prod build with AoT compilation
$ npm run build.prod.exp
When using AoT compilation, please consider the following:
Currently you cannot use custom component decorators with AoT compilation. This may change in the future but for now you can use this pattern for when you need to create AoT builds for the web:
import { Component } from '@angular/core';
import { BaseComponent } from '../frameworks/core/index';
// @BaseComponent({ // just comment this out and use Component from 'angular/core'
@Component({
// etc.
After doing the above, running AoT build via npm run build.prod.exp
will succeed. :)
BaseComponent
custom component decorator does the auto templateUrl
switching to use {N} views when running in the {N} app therefore you don't need it when creating AoT builds for the web. However just note that when going back to run your {N} app, you should comment back in the BaseComponent
. Again this temporary inconvenience may be unnecessary in the future.
npm install -g nativescript
You can make changes to files in src/client
or nativescript
folders. A symbolic link exists between the web src/client
and the nativescript
folder so changes in either location are mirrored because they are the same directory inside.
Create .tns.html
and .tns.css
NativeScript view files for every web component view file you have. You will see an example of the app.component.html
as a NativeScript view file here.
iOS: npm run start.ios
iOS (livesync emulator): npm run start.livesync.ios
iOS (livesync device): npm run start.livesync.ios.device
// or...
Android: npm run start.android
Android (livesync emulator): npm run start.livesync.android
Android (livesync device): npm run start.livesync.android.device
OR...
You can greatly reduce the final size of your NativeScript app by the following:
cd nativescript
npm i nativescript-dev-webpack --save-dev
Then you will need to modify your components to not use moduleId: module.id
and change templateUrl
to true relative app, for example:
before:
@BaseComponent({
moduleId: module.id,
selector: 'sd-home',
templateUrl: 'home.component.html',
styleUrls: ['home.component.css']
})
after:
@BaseComponent({
// moduleId: module.id,
selector: 'sd-home',
templateUrl: './app/components/home/home.component.html',
styleUrls: ['./app/components/home/home.component.css']
})
Then to build:
Ensure you are in the nativescript
directory when running these commands.
WEBPACK_OPTS="--display-error-details" tns build ios --bundle
WEBPACK_OPTS="--display-error-details" tns build android --bundle
Notice your final build will be drastically smaller. In some cases 120 MB -> ~28 MB. 👍
Mac: npm run start.desktop
Windows: npm run start.desktop.windows
Mac: npm run start.livesync.desktop
Windows: npm run start.livesync.desktop.windows
Mac: npm run build.desktop.mac
Windows: npm run build.desktop.windows
Linux: npm run build.desktop.linux
$ npm test
# Development. Your app will be watched by karma
# on each change all your specs will be executed.
$ npm run test.watch
# NB: The command above might fail with a "EMFILE: too many open files" error.
# Some OS have a small limit of opened file descriptors (256) by default
# and will result in the EMFILE error.
# You can raise the maximum of file descriptors by running the command below:
$ ulimit -n 10480
# code coverage (istanbul)
# auto-generated at the end of `npm test`
# view coverage report:
$ npm run serve.coverage
# e2e (aka. end-to-end, integration) - In three different shell windows
# Make sure you don't have a global instance of Protractor
# npm install webdriver-manager <- Install this first for e2e testing
# npm run webdriver-update <- You will need to run this the first time
$ npm run webdriver-start
$ npm run serve.e2e
$ npm run e2e
# e2e live mode - Protractor interactive mode
# Instead of last command above, you can use:
$ npm run e2e.live
You can learn more about Protractor Interactive Mode here
Default application server configuration
var PORT = 5555;
var LIVE_RELOAD_PORT = 4002;
var DOCS_PORT = 4003;
var APP_BASE = '/';
Configure at runtime
npm start -- --port 8080 --reload-port 4000 --base /my-app/
If you have different environments and you need to configure them to use different end points, settings, etc. you can use the files dev.ts
or prod.ts
in./tools/env/
. The name of the file is environment you want to use.
The environment can be specified by using:
$ npm start -- --env-config ENV_NAME
Currently the ENV_NAME
s are dev
, prod
, staging
, but you can simply add a different file "ENV_NAME.ts".
file in order to alter extra such environments.
A documentation of the provided tools can be found in tools/README.md.
src/client/assets/i18n/
[language code].json
(copy existing one and adapt the translation strings)src/client/app/frameworks/sample/services/app-config.spec.ts
src/client/app/frameworks/sample/services/app-config.ts
SUPPORTED_LANGUAGES
src/client/app/frameworks/i18n/components/lang-switcher.component.spec.ts
Please Note: The seed uses Angular's ChangeDetectionStrategy.OnPush
by default which requires some understanding of immutability and one-way data flows. Please check out the following resources to learn more:
If you experience issues with changes not occuring in your views, you can disable this by commenting out these lines. The seed uses OnPush
by default because it provides optimal performance and if you decide to turn it off while developing your application, you can always turn it back on when you're ready to refactor your data services to utilize OnPush
properly.
There’s actually only a few things to keep in mind when sharing code between web/mobile. The seed does take care of quite a few of those things but here’s a brief list:
OpaqueTokens
which is a fancy name for something quite simple. Learn more here. A great example of how to integrate 2 different plugins (1 for web, 1 for {N}) and share all the code exists in this wiki article: How to integrate Firebase across all platforms written by the awesome Scott Lowe.WindowService
provided by the seed instead. This includes usage of alert
, confirm
, etc. For example:If you were thinking about doing: alert('Something happened!');
, Don't.
Instead inject WindowService
:
constructor(private win: WindowService) {}
public userAction() {
if (success) {
// do stuff
} else {
this.win.alert('Something happened!');
}
}
This ensures that when the same code is run in the {N} app, the native dialogs
module will be used.
The advice I like to give is:
Code with web mentality first. Then provide the native capability using Angular’s
{provide: SomeWebService, useClass: SomeNativeService }
during bootstrap.
There are some cases where you may want to use useValue
vs. useClass
, and other times may need to use useFactory
. Read the Angular docs here to learn more about which you may need for your use case.
Several branches exist with certain features integrated:
NOTE: This should be done first before you start making any changes and building out your project. Not doing so will likely result in dificulty when trying to merge in upstream changes later.
npm run git.setup
- This will initialize git
as well as setup upstream
properly.git remote add origin ...your private repo...
npm run git.prepare
- This will prepare git to handle the mergenpm run git.merge
- This will fetch upstream and run the first merge (*Important)upstream
and your first commit) added.unknown option --allow-unrelated-histories
either upgrade git to 2.9+ or use npm run git.merge.legacy
git add .; git commit -m'ready'
. Yes, you will be committing all those conflicts, which actually are not a problem in this 1 time case.git
setup and ready to develop your application as well as merge in upstream changes in the future.npm install
(and all other usage docs in this README
apply)framework
for your application in src/client/app/frameworks
to build your codebase out. Say your app is called AwesomeApp
, then create awesomeapp
and start building out all your components and services in there. Create other frameworks as you see fit to organize.i18n
, remove ng2-translate
as dependency root package.json
and nativescript/package.json
. Then remove any references to i18n
throughout.npm run git.merge.preview
- This will fetch upstream
and show you how the merge would lookunknown option --allow-unrelated-histories
either upgrade git to 2.9+ or use npm run git.merge.legacy.preview
npm run git.merge
- This will actually do the mergeunknown option --allow-unrelated-histories
either upgrade git to 2.9+ or use npm run git.merge.legacy
You can read more about syncing a fork here.
If you have any suggestions to this workflow, please post here.
Please see the CONTRIBUTING file for guidelines.
mgechev | ludohenin | NathanWalker | d3viant0ne | Shyam-Chen | tarlepp |
Nightapes | TheDonDope | nareshbhatia | kiuka | hank-ehly | daniru |
jesperronn | njs50 | vyakymenko | robstoll | sasikumardr | aboeglin |
sfabriece | JakePartusch | ryzy | markwhitfeld | m-abs | eppsilon |
jvitor83 | gkalpak | ivannugo | pgrzeszczak | natarajanmca11 | natarajanmca11 |
jerryorta-dev | domfarolino | larsthorup | ouq77 | LuxDie | admosity |
irsick | amedinavalencia | troyanskiy | tsm91 | juristr | JohnCashmore |
JayKan | devanp92 | evanplaice | hAWKdv | Kaffiend | JunaidZA |
c-ice | markharding | ojacquemart | tiagomapmarques | TuiKiken | gotenxds |
edud69 | turbohappy | karlhaas | kbrandwijk | robbatt | Bigous |
ip512 | Green-Cat | Yonet | divramod | daixtrose | taguan |
bbarry | yassirh | sonicparke | brendanbenson | brian428 | briantopping |
ckapilla | cadriel | dszymczuk | dmurat | peah90 | dstockhammer |
dwido | dcsw | totev | nosachamos | ericli1018 | koodikindral |
amaltsev | Falinor | hpinsley | NN77 | isidroamv | jeffbcross |
isidroamv | jeffbcross | Jimmysh | Drane | johnjelinek | fourctv |
JunusErgin | justindujardin | lihaibh | Brooooooklyn | tandu | inkidotcom |
daixtrose | mjwwit | oferze | ocombe | gdi2290 | typekpb |
philipooo | pidupuis | redian | robertpenner | Sjiep | RoxKilly |
sclausen | heavymery | tjvantoll | tapas4java | gitter-badger | valera-rozuvan |
vincentpalita | Yalrafih | arnaudvalle | billsworld | blackheart01 | butterfieldcons |
danielcrisp | jgolla | omerfarukyilmaz | pbazurin-softheme | rossedfort | savcha |
sebfag | ultrasonicsoft | urmaul |
MIT
FAQs
High-quality, modular starter (advanced seed) project for Angular 2 apps with statically typed build and AoT + NativeScript + Electron
The npm package angular2-seed-advanced receives a total of 4 weekly downloads. As such, angular2-seed-advanced popularity was classified as not popular.
We found that angular2-seed-advanced demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.