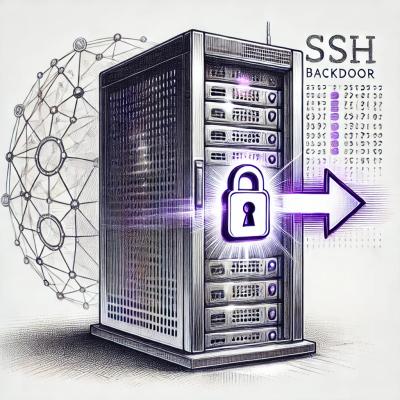
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
The purpose of this library is to consolidate the behaviours of various database drivers into a minimal and consistent API. See the design document for a thorough overview of the planned API.
Things it does:
driver://user:pass@host/database
pool.query("SELECT 1", function (err, results) { ... })
Things it will do soon:
Things it might do:
Things it will never do:
.first
or .fetchAll
Creating a connection:
var anyDB = require('any-db')
, conn = anyDB.createConnection('postgres://user:pass@localhost/dbname')
Simple queries with callbacks are exactly what you'd expect:
conn.query("SELECT * FROM my_table LIMIT 10", function (err, result) {
for (var i in result.rows) {
console.log("Row " + i + ": %j", rows[i])
}
})
If no callback is provided, the query object returned will emit the following events:
var query = conn.query('SELECT * FROM my_table')
query.on('fields', function (fields) { /* fields is an array of field names */ })
query.on('row', function (row) { /* row is plain object */ })
query.on('end', function () { /* always emitted when results are exhausted */ })
query.on('error', function () { /* emitted on errors :P */ })
To use bound parameters simply pass an array as the second argument to query:
conn.query('SELECT * FROM users WHERE gh_username = $1', ['grncdr'])
You can also use named parameters by passing an object instead:
conn.query('SELECT * FROM users WHERE gh_username = $username', {username: 'grncdr'})
Any-db doesn't do any parameter escaping on it's own, so you can use any advanced parameter escaping features of the underlying driver exactly as though any-db wasn't there.
You can create a connection pool with anyDB.createPool
:
var pool = anyDB.createPool('postgres://user:pass@localhost/dbname', {
min: 5, // Minimum connections
max: 10, // Maximum connections
onConnect: function (conn, ready) {
/*
perform any necessary connection setup before calling ready(err, conn)
*/
},
reset: function (conn, ready) {
/*
perform any necessary reset of connection state before the connection can
be re-used. The default callback does conn.query("ROLLBACK", ready)
*/
}
})
A connection pool has a query
method that acts exactly like the one on
connections, but the underlying connection is returned to the pool when the
query completes.
Both connections and pools have a begin
method that starts a new transaction
and returns a Transaction
object. Transaction objects behave much like
connections, but instead of an end
method, they have commit
and rollback
methods. Additionally, an unhandled error emitted by a transaction query will
cause an automatic rollback of the transaction before being re-emitted by the
transaction itself.
Here's an example where we stream all of our user ids, check them against an external abuse-monitoring service, and flag or delete users as necessary, if for any reason we only get part way through, the entire transaction is rolled back and nobody is flagged or deleted:
var tx = pool.begin()
tx.on('error', finished)
/*
Why query with the pool and not the transaction?
Because it allows the transaction queries to begin executing immediately,
rather than queueing them all up behind the initial SELECT.
*/
pool.query('SELECT id FROM users').on('row', function (user) {
if (tx.state() == 'rolled back') return
abuseService.checkUser(user.id, function (err, result) {
if (err) return tx.handleError(err)
// Errors from these queries will propagate up to the transaction object
if (result.flag) {
tx.query('UPDATE users SET abuse_flag = 1 WHERE id = $1', [user.id])
} else if (result.destroy) {
tx.query('DELETE FROM users WHERE id = $1', [user.id])
}
})
}).on('error', function (err) {
tx.handleError(err)
}).on('end', function () {
tx.commit(finished)
})
function finished (err) {
if (err) console.error(err)
else console.log('All done!')
}
MIT
FAQs
Database-agnostic connection pooling, querying, and result sets
The npm package any-db receives a total of 1,675 weekly downloads. As such, any-db popularity was classified as popular.
We found that any-db demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.