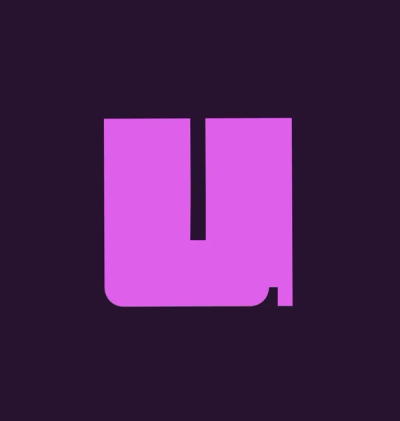
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
ao-deploy-beta
Advanced tools
A package for deploying AO contracts.
npm install ao-deploy --save-dev
pnpm add ao-deploy --save-dev
yarn add ao-deploy --dev
bun add ao-deploy --dev
Usage: ao-deploy [options] <contractOrConfigPath>
Deploy AO contracts using a CLI.
Arguments:
contractOrConfigPath Path to the main contract file or deployment configuration.
Options:
-V, --version output the version number
-n, --name [name] Specify the process name. (default: "default")
-w, --wallet [wallet] Path to the wallet JWK file.
-l, --lua-path [luaPath] Specify the Lua modules path seperated by semicolon.
-d, --deploy [deploy] List of deployment configuration names, separated by commas.
-s, --scheduler [scheduler] Scheduler to be used for the process. (default: "_GQ33BkPtZrqxA84vM8Zk-N2aO0toNNu_C-l-rawrBA")
-m, --module [module] Module source for spawning the process.
-c, --cron [interval] Cron interval for the process (e.g. 1-minute, 5-minutes).
-t, --tags [tags...] Additional tags for spawning the process.
-p, --process-id [processId] Specify process Id of existing process.
--concurrency [limit] Concurrency limit for deploying multiple processes. (default: "5")
--retry-count [count] Number of retries for deploying contract. (default: "10")
--retry-delay [delay] Delay between retries in milliseconds. (default: "3000")
-h, --help display help for command
ao-deploy process.lua -n tictactoe -w wallet.json --tags name1:value1 name2:value2
Here is an example using a deployment configuration:
// aod.config.ts
import { defineConfig } from 'ao-deploy'
const wallet = 'wallet.json'
const luaPath = './?.lua;./src/?.lua'
const config = defineConfig({
contract_1: {
luaPath,
name: `contract-1`,
contractPath: 'contract-1.lua',
wallet,
},
contract_2: {
luaPath,
name: `contract-2`,
contractPath: 'contract-2.lua',
wallet,
},
contract_3: {
luaPath,
name: `contract-3`,
contractPath: 'contract-3.lua',
wallet,
}
})
export default config
Deploy all specified contracts:
ao-deploy aod.config.ts
Deploy specific contracts:
ao-deploy aod.config.ts --deploy=contract_1,contract_3
[!Note] A wallet is generated and saved if not passed.
Retrieve the generated wallet path:
node -e "const path = require('path'); const os = require('os'); console.log(path.resolve(os.homedir(), '.aos.json'));"
To deploy a contract, you need to import and call the deployContract
function from your script. Here is a basic example:
import { deployContract } from 'ao-deploy'
async function main() {
try {
const { messageId, processId } = await deployContract(
{
name: 'demo',
wallet: 'wallet.json',
contractPath: 'process.lua',
tags: [{ name: 'Custom', value: 'Tag' }],
retry: {
count: 10,
delay: 3000,
},
},
)
const processUrl = `https://ao_marton.g8way.io/#/process/${processId}`
const messageUrl = `${processUrl}/${messageId}`
console.log(`\nDeployed Process: ${processUrl} \nDeployment Message: ${messageUrl}`)
}
catch (error: any) {
console.log(`Deployment failed!: ${error?.message ?? 'Failed to deploy contract!'}\n`)
}
}
main()
The deployContract
function accepts the following parameters within the DeployArgs object:
name
(optional): The process name to spawn. Defaults to "default".contractPath
: The path to the contract's main file.module
(optional): The module source to use. Defaults to fetching from the AOS's GitHub repository.scheduler
(optional): The scheduler to use for the process. Defaults to _GQ33BkPtZrqxA84vM8Zk-N2aO0toNNu_C-l-rawrBA
.tags
(optional): Additional tags to use for spawning the process.cron
(optional): The cron interval for the process, e.g., "1-minute", "5-minutes". Use format interval-(second, seconds, minute, minutes, hour, hours, day, days, month, months, year, years, block, blocks, Second, Seconds, Minute, Minutes, Hour, Hours, Day, Days, Month, Months, Year, Years, Block, Blocks)
wallet
(optional): The wallet path or JWK itself (Autogenerated if not passed).retry
(optional): Retry options with count
and delay
properties. By default, it will retry up to 10
times with a 3000
milliseconds delay between attempts.luaPath
(optional): The path to the Lua modules seperated by semicolon.processId
(optional): The process id of existing process.To deploy contracts, you need to import and call the deployContracts
function from your script. Here is a basic example:
import { deployContracts } from 'ao-deploy'
async function main() {
try {
const results = await deployContracts(
[
{
name: 'demo1',
wallet: 'wallet.json',
contractPath: 'process1.lua',
tags: [{ name: 'Custom', value: 'Tag' }],
retry: {
count: 10,
delay: 3000,
},
},
{
name: 'demo2',
wallet: 'wallet.json',
contractPath: 'process2.lua',
tags: [{ name: 'Custom', value: 'Tag' }],
retry: {
count: 10,
delay: 3000,
},
}
],
2
)
results.forEach((result, idx) => {
if (result.status === 'fulfilled') {
const { processId, messageId } = result.value
const processUrl = `https://ao_marton.g8way.io/#/process/${processId}`
const messageUrl = `${processUrl}/${messageId}`
console.log(`\nDeployed Process: ${processUrl} \nDeployment Message: ${messageUrl}`)
}
else {
console.log(`Failed to deploy contract!: ${result.reason}\n`)
}
})
}
catch (error: any) {
console.log(`Deployment failed!: ${error?.message ?? 'Failed to deploy contract!'}\n`)
}
}
main()
👤 Pawan Paudel
Contributions, issues and feature requests are welcome! \ Feel free to check issues page.
Give a ⭐️ if this project helped you!
Copyright © 2024 Pawan Paudel.
FAQs
A package for deploying AO contracts
The npm package ao-deploy-beta receives a total of 16 weekly downloads. As such, ao-deploy-beta popularity was classified as not popular.
We found that ao-deploy-beta demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.