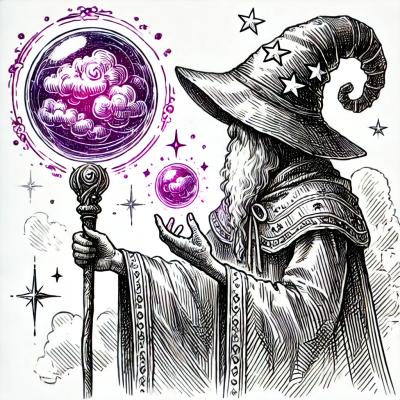
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
api-doc-validator
Advanced tools
Parameter in brackets means it's optional, like [CODE]
. Parameters with pipe sign |
means or
, like json-schema|OBJECT_NAME
.
@url METHOD path
/**
* @url POST /path/:param
*/
Validate parameters of @url
path
@params [OBJECT_NAME =] json-schema|OBJECT_NAME
/**
* @url GET /users/:id
* @params {
* id: number,
* }
* @call users.get(id)
*/
or with OBJECT_NAME
assing for future use
/**
* @url GET /users/:id
* @params User = {
* id: number,
* }
*/
or use external schema as root schema
/**
* @url GET /users/:id
* @params User
*/
or extend external schema
/**
* @url GET /users/:id
* @params {
* ...User,
* name: string,
* }
*/
Validate @url
query parameters
@query [OBJECT_NAME =] json-schema|OBJECT_NAME
/**
* @url GET /users
* @query {
* id: number,
* }
* @call users.get(id)
*/
Example of valid request GET /users?id=1
Names of fields in @params
and query
should be different to use them in @call
/**
* @url GET /users/:id
* @params {
* id: number,
* }
* @query {
* name: string,
* }
* @call users.get(id, name)
*/
@body [OBJECT_NAME =] json-schema|OBJECT_NAME
/**
* @body {
* id: number,
* name: string,
* }
*/
Response http code and validation of response body.
@response [CODE] [OBJECT_NAME =] json-schema|OBJECT_NAME
Response for 200
code
/**
* @response {
* id: number,
* name: string,
* }
*/
Validators for different codes of same request
/**
* @response 200 {
* id: number,
* name: string,
* }
* @response 500 {
* message: string,
* }
*/
Define new schema for future usage
@schema OBJECT_NAME = json-schema|OBJECT_NAME
/**
* @schema User = {
* id: number,
* name: string,
* }
*/
or just to make shorter schema name
/**
* @schema User = SomeVeryLongSchemaName
*/
or even condition
/**
* @schema Product = {properties: {price: {minimum: 100}}} ? ExpensiveProduct : CheapProduct
*/
You should provide valid js code of method call. This code will be used in your API tests.
@call object-method-call
/**
* @call object.method(param1, param2)
*/
HTTP request method.
GET|POST|PUT|DELETE|HEAD|OPTIONS
HTTP response code like 200 or 500 etc. Default is 200.
URL pathname
. For path parsing used path-to-regexp lib.
Parameters like :id
can be used in @call
as parameter of method call with same name.
/**
* @url GET /users/:id(\d+)
* @call users.get(id)
*/
Any valid js object name like objectName
or with field of any deep objectName.fieldName.field
Object that describes how validate another object. For object validation used ajv lib with few modifications for less code writing.
Default ajv schema
schema = {
id: {
type: "number",
// extra fields for number: maximum, minimum, exclusiveMaximum, exclusiveMinimum, multipleOf
},
name: {
type: "string",
// extra fields for string: maxLength, minLength, pattern, format
},
enabled: {
type: "boolean",
// no extra fields
},
list: {
type: "array",
// extra fields for array: maxItems, minItems, uniqueItems, items, additionalItems, contains
},
user: {
type: "object",
// extra fields for object: maxProperties, minProperties, required, properties, patternProperties,
// additionalProperties, dependencies, propertyNames
},
enumOfStrings: {
type: "string",
enum: ["user", "guest", "owner"]
},
}
Simplified description of schema
schema = {
id: number,
name: string,
enabled: boolean,
listOfObjects: [{
id: number,
type: string,
}],
listOfNumbers: [{
type: "number"
}],
// which means list is array of numbers
user: {
id: number,
type: string,
},
enumOfStrings: "user" || "guest" || "owner",
}
So, if any object in a schema (including root) has field type
with one of the string values
"number"
, "integer"
, "string"
, "boolean"
, "array"
, "object"
or "null"
than it means this object is validator.
In any other cases this object will be converted to "object"
validator. Example
schema = {
days: [number],
list: [{
id: number,
type: string,
}],
user: {
id: number,
type: string,
},
parent: {
type: "object",
},
}
Will be converted to
schema = {
days: {
type: "array",
items: {
type: "number"
}
},
list: {
type: "array",
items: {
type: "object",
required: ["id", "type"],
properties: {
id: {
type: "number"
},
type: {
type: "string"
},
}
}
},
user: {
type: "object",
required: ["id", "type"],
properties: {
id: {
type: "number"
},
type: {
type: "string"
},
}
},
parent: {
type: "object",
},
}
By default, all fields in an object are required. To make field optional just put it in brackets.
schema = {
id: number,
[name]: string,
}
schema = {
type: "object",
required: ["id"],
properties: {
id: {type: "number"},
name: {type: "string"},
},
}
Instead of short number
validator you can use one of following number patterns as value of object field.
int
number without floating-pointpositive
positive number including 0
negative
negative number excluding 0
id
number more than 0
schema = {
id: id,
price: positive,
list: [int],
}
Will be converted to
schema = {
id: {
type: "number",
minimum: 1,
},
price: {
type: "number",
minimum: 0,
},
list: {
type: "array",
items: {
type: "integer",
}
},
}
Instead of short string
validator you can use one of following string patterns as value of object field.
date
full-date according to RFC3339.time
time with optional time-zone.date-time
date-time from the same source (time-zone is optional, in ajv it's mandatory)date-time-tz
date-time with time-zone requireduri
full URI.uri-reference
URI reference, including full and relative URIs.uri-template
URI template according to RFC6570email
email address.hostname
host name according to RFC1034.ipv4
IP address v4.ipv6
IP address v6.regex
tests whether a string is a valid regular expression by passing it to RegExp constructor.uuid
Universally Unique IDentifier according to RFC4122.schema = {
id: uuid,
email: email,
created_at: date-time,
days: [date],
}
Will be converted to
schema = {
id: {
type: "string",
format: "uuid",
},
email: {
type: "string",
format: "email",
},
created_at: {
type: "string",
format: "date-time",
},
days: {
type: "array",
items: {
type: "string",
format: "date",
}
},
}
Using OBJECT_NAME
you can inject external schema in current schema.
/**
* @url GET /users/:id
* @response User = {
* id: number,
* name: string,
* }
*/
/**
* @url POST /users
* @body {
* action: 'update' || 'delete',
* user: User,
* }
*/
Instead of anyOf
you can use ||
operator
schema = {
data: User || Account || {type: "object"}
}
will be
schema = {
type: "object",
properties: {
data: {
anyOf: [
{/* schema of User */},
{/* schema of Account */},
{type: "object"},
]
}
}
}
Instead of allOf
you can use &&
operator
schema = {
data: User && Account && {type: "object"}
}
will be
schema = {
type: "object",
properties: {
data: {
allOf: [
{/* schema of User */},
{/* schema of Account */},
{type: "object"},
]
}
}
}
To extend you can use object spread operator
User = {
id: number,
data: string,
}
UserExtra = {
name: string,
created_at: date,
}
schema = {
user: {
...User,
...UserExtra,
data: undefined, // remove field
created_at: date-time, // overwrite field
},
}
will be
schema = {
type: "object",
properties: {
user: {
type: "object",
properties: {
id: {type: "number"},
name: {type: "string"},
created_at: {type: "string", format: "date-time"},
}
},
},
}
Uniq sample of JavaScript code with some method call of some object, which will be generated for testing purposes.
In method call you can use named parameters from @url
/**
* @url GET /users
* @call users.get()
*/
/**
* @url GET /users/:id
* @call users.get(id)
*/
/**
* @url POST /users/:id/settings
* @call users.setSettings(id)
*/
/**
* @url POST /users/:id/settings
* @call users.settings.update(id)
*/
There can be any number of nested objects and any method name. Only names of parameters should be equal.
with npx
npx adv -c path/to/config.json
or add it to package.json
to "scripts":
section
"scripts": {
"adv": "adv"
}
and run npm run adv -c path/to/config.json
Parameters:
-c, --config <path> path to config json file
-t, --tests <path> generate validator for tests
-h, --host <address> host for tests requests
--help display help for command
include
array of paths to files relative to config path, glob pattern usedexclude
array of paths to files to be excludedhost
base url address for tests requeststests
path for output file of tests requests{
"include": [
"src/**"
],
"exclude": [
"src/tests"
],
"host": "https://api.openweathermap.org",
"tests": "output/tests.js"
}
FAQs
api doc and validator
The npm package api-doc-validator receives a total of 4 weekly downloads. As such, api-doc-validator popularity was classified as not popular.
We found that api-doc-validator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.