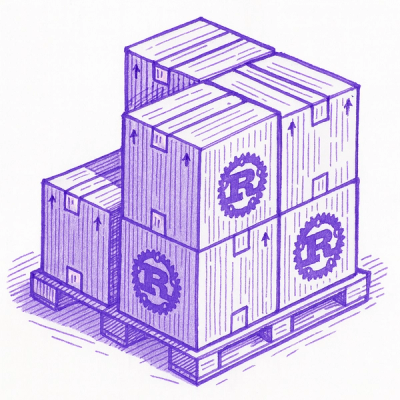
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Simple Node.js class for performing HTTP requests.
npm install api-http
var APIHTTP = require('api-http');
var facebook = new APIHTTP('https://graph.facebook.com/v2.5/');
facebook.get('me')
.then(function (person) {
console.log(person.first_name);
});
Arguments: [path, query]
Example Usage:
var api = require('api-http')('http://example.com/');
// http://example.com/users?online=true
api.get('users', {online: true});
Arguments: [path, body, query]
Example Usage:
api.post('customers', {
firstName: 'John',
lastName: 'Smith'
});
Create a new APIHTTP client scoped with an OAuth Bearer access token:
Example:
facebook.token('2348923984324').get('me')
Create a new APIHTTP client scoped with a Basic Access Authorization header:
Example:
api.basic('Aladdin', 'open sesame').get('something/x/y')
Scope the client to an OAuth Bearer access token. This is an alternative to using the .token(accessToken)
method, which would be more convenient if you are using different access tokens for multiple requests.
Function: [res]
Transform responses with a 4xx or 5xx HTTP status.
The default implementation will try to pick up the error message to set .status
, .name
, and .code
automatically.
Example:
var client = new APIHTTP('http://localhost:8080', {
error: function rejectHandler(res) {
throw new Error(res.message);
}
})
Function: [res]
Transform responses with 2xx HTTP status codes.
If you wanted, you could pass the result through camelize or something.
Default implementation:
function success(res) {
return res.data;
}
Function: []
Function which returns the headers for the request.
In general, you will never need to pass this option unless you are overriding the authentication logic. If you wanted to set a custom user-agent, or add a correlation ID, subclass this method and don't forget to call super()
.
Note: All of the options simply set keys on this
which means you can easily subclass APIHTTP.
FAQs
Simple Node.js class for performing HTTP API requests.
The npm package api-http receives a total of 17 weekly downloads. As such, api-http popularity was classified as not popular.
We found that api-http demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.