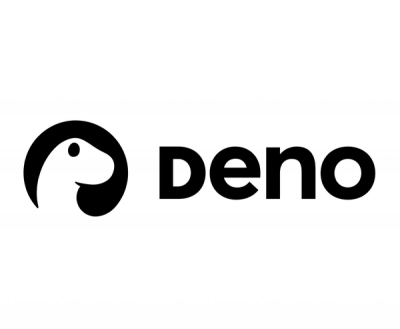
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Object relational mapping (ORM) framework for API Builder.
This software is pre-release and not yet ready for usage. Please don't use this just yet while we're working through testing and finishing it up. Once it's ready, we'll make an announcement about it.
Please see the CHANGELOG for the latest changes.
There are 4 main components to the ORM framework:
To define a model, you must give a set of fields and a connector.
var User = orm.Model.define('user',{
fields: {
name: {
type: String,
default: 'Jeff',
}
},
connector: Connector
});
The first argument is the name of the model. The second argument is the definition of the model.
The following are Model field properties:
Name | Description |
---|---|
type | the column type (such as String, Number, etc) |
required | if true, the field is required |
The model has several instance methods:
Name | Description |
---|---|
extend | create a new Model class from the current Model |
create | create a new Model instance |
update | update a Model instance |
remove | remove a Model instance |
removeAll | remove all Model instances |
find | find one or more Models |
findOne | find one Model from a primary key |
findAll | find all Model |
A model can have custom functions by defining them in the definition as a property. They will automatically be available on the model instance.
var User = orm.Model.define('user',{
fields: {
name: {
type: String,
required: true,
default: 'jeff'
}
},
connector: Connector,
// implement a function that will be on the Model and
// available to all instances
getProperName: function() {
// this points to the instance when this is invoked
return this.name.charAt(0).toUpperCase() + this.name.substring(1);
}
});
User.create(function(err,user){
console.log(user.getProperName());
});
One you've defined a model, you can then use it to create an Instance of the Model.
User.create({name:'Nolan'}, function(err,user){
// you now have a user instance
});
Instances has several methods for dealing with the model.
Name | Description |
---|---|
get | get the value of a field property |
set | set a value or a set of values (Object) |
isUnsaved | returns true if the instance has pending changes |
isDeleted | returns true if the instance has been deleted and cannot be used |
update | save any pending changes |
remove | remove this instance (delete it) |
getPrimaryKey | return the primary key value set by the Connector |
In addition to get
and set
, you can also use property accessors to get field values.
console.log('name is',user.name);
user.name = 'Rick';
If the Connector returns more than one Model instance, it will return it as a Collection, which is a container of Model instances.
A collection is an array and has additional helper functions for manipulating the collection.
You can get the length of the collection with the length
property.
To create a connector, you can either inherit from the Connector
class using utils.inherit
or extend:
var MyConnector = orm.Connector.extend({
constructor: function(){
},
findOne: function(Model, id, callback) {
}
});
Once you have created a Connector class, you can create a new instance:
var connector = new MyConnector({
url: 'http://foobar.com'
});
This software is licensed under the Apache 2 Public License. However, usage of the software to access the Appcelerator Platform is governed by the Appcelerator Enterprise Software License Agreement.
FAQs
API Builder ORM
The npm package api-orm receives a total of 4 weekly downloads. As such, api-orm popularity was classified as not popular.
We found that api-orm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.