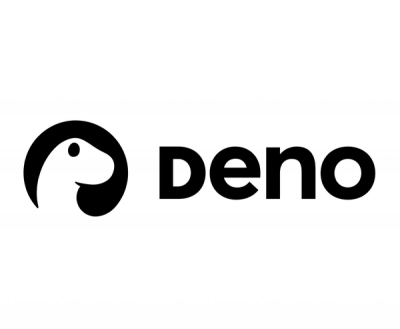
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
npm install appex
Appex is a nodejs web application and service framework built on top of the TypeScript programming language. Appex enables nodejs developers to expose typescript functions as http endpoints as well as generate meaningful service meta data for clients to consume.
Appex also provides a dynamic compilation environment for typescript to aid in development. Appex will effeciently manage compilation in the background without the need to restart the web server, or use addition modules.
## getting started ```javascript //---------------------------------------------- // file: program.ts //---------------------------------------------- export module services {// url: http://localhost:3000/services/message
export function message (context) {
context.response.write('hello typescript');
context.response.end();
}
} //---------------------------------------------- // file: app.js //----------------------------------------------
var appex = require('appex');
var runtime = appex.runtime ({ source : './program.ts', devmode : true });
require('http').createServer( runtime ).listen(3000);
<a name="getting_started_on_express" />
## getting started on express
```javascript
//----------------------------------------------
// file: program.ts
//----------------------------------------------
export module services {
// url: http://localhost:3000/services/message
export function message (context) {
context.response.write('hello typescript');
context.response.end();
}
}
//----------------------------------------------
// file: app.js
//----------------------------------------------
var express = require('express');
var appex = require('appex');
var app = express();
app.use( appex.runtime( { source:'./program.ts', devmode:true } ) );
app.get('/', function(req, res){
res.send('Hello World');
});
app.listen(3000);
## developing with appex
To enable development mode, set the devmode option to true on the runtime option parameter.
// enable dynamic compilations with the devmode option.
var runtime = appex.runtime ({ source : './program.ts', devmode : true });
Appex is built directly on top of the Microsoft TypeScript 0.9 compiler and leverages it for tight integration with the nodejs platform. By enabling the 'devmode' option will have the compiler effiecently rebuild typescript source code on each request made to the server.
Appex achieves performance in this regard by leveraging features introduced in TS 0.9 which allows incremental building / caching of typescript compilation units.
In addition to this, compilations are run in a background worker process to ensure they do interupt requests being served on the web process.
The benefit to this is that updates can be made to source files without needing to restart the web process. Additionally, syntactic errors made in typescript source code do not bring the web process. Everything stays running (excluding runtime errors).
Appex will output detailed syntax and type errors on the main process stdout stream, as well as a http response.
## functionsAppex supports two types of functions, http handler functions, and json handler functions.
Appex will only create http handlers for functions a specific signature, these are outlined below.
### http handler functionA http handler method can be created with the following function signature.
The return type is optional. http handler functions should complete the http request.
export function method(context:any) : void {
context.response.write('hello world');
context.response.end();
}
### json handler function
A json handler is a function which will accept HTTP POST'ed json strings and pass it to the function as a object. A json handler has three distinct arguments:
The return type is optional. json handler functions "must" call the callback to complete the request.
export function method(context:any, request:any, callback:(response:any) => void) : void {
callback(request); // echo the object back.
}
### public and private functions
Appex extends TypeScripts concept of visibility to include visibility over http. From this developers and control which functions are exported as http handlers.
Appex will create routes only for functions marked with export and for functions that reside withing modules with export.
Consider the following example:
module private_module {
export function public_method () {
// this function is exported, but as this module is
// not exported, neither is this method.
}
}
function private_function() {
// this method is private
}
export function public_function (context:any) {
private_function(); // ok
private_module.public_method(); // ok
}
which will result in a single route.
http://[host]:[port]/public_function
### the index function
Appex denotes that functions named 'index' route to the current module scope. As demonstrated below.
export function index(context) {}
export module blogs {
export function index (context) { }
export function get (context) { }
}
// results in the following routes
// http://[host]:[port]/
// http://[host]:[port]/blogs
// http://[host]:[port]/blogs/get
### routing with modules and functions
Appex creates url routing tables based on function name and module scope. For example consider the following...
export function index (context:any) { }
export function about (context:any) { }
export function contact (context:any) { }
export module services.customers {
export function insert(context:any) : void { }
export function update(context:any) : void { }
export function delete(context:any) : void { }
}
// results in the following routes
// http://[host]:[port]/
// http://[host]:[port]/about
// http://[host]:[port]/contact
// http://[host]:[port]/services/customers/insert
// http://[host]:[port]/services/customers/update
// http://[host]:[port]/services/customers/delete
## structuring projects
Appex leverages TypeScript's ability to reference source files with the element. Appex will traverse each source files references and include it as part of the compilation.
Developers can use this functionality to logically split source files into reusable components of functionality, as demonstrated below.
//---------------------------------------------------
// file: app.js
//---------------------------------------------------
var appex = require('appex');
var runtime = appex.runtime ({ source : './index.ts', devmode : true });
require('http').createServer( runtime ).listen(3000);
//---------------------------------------------------
// file: index.ts
//---------------------------------------------------
/// <reference path="pages.ts" />
/// <reference path="users.ts" />
//---------------------------------------------------
// file: pages.ts
//---------------------------------------------------
export function index (context) { /* handle request */ }
export function about (context) { /* handle request */ }
export function contact (context) { /* handle request */ }
//---------------------------------------------------
// file: users.ts
//---------------------------------------------------
export module users {
export function login (context) { /* handle request */ }
export function logout (context) { /* handle request */ }
}
## typescript resources
FAQs
develop nodejs web applications with typescript
The npm package appex receives a total of 5 weekly downloads. As such, appex popularity was classified as not popular.
We found that appex demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.