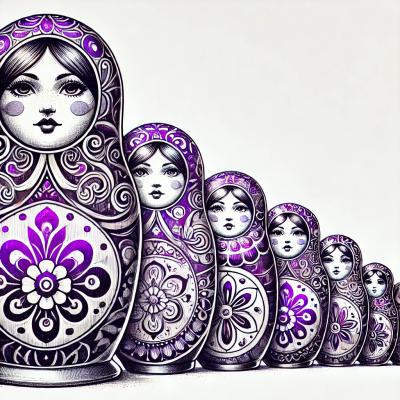
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
appium-chromedriver
Advanced tools
The appium-chromedriver npm package is a Node.js wrapper around the Chromedriver binary, which is used to automate Chrome-based browsers for testing purposes. It is primarily used in conjunction with Appium to facilitate automated testing of web applications on mobile devices.
Install Chromedriver
This feature allows you to install the Chromedriver binary required for automating Chrome-based browsers. The code sample demonstrates how to set up Chromedriver using the appium-chromedriver package.
const Chromedriver = require('appium-chromedriver');
const driver = new Chromedriver();
driver.setupChromedriver().then(() => console.log('Chromedriver installed successfully'));
Start Chromedriver
This feature allows you to start the Chromedriver server. The code sample demonstrates how to start Chromedriver using the appium-chromedriver package.
const Chromedriver = require('appium-chromedriver');
const driver = new Chromedriver();
driver.start().then(() => console.log('Chromedriver started successfully'));
Stop Chromedriver
This feature allows you to stop the Chromedriver server. The code sample demonstrates how to stop Chromedriver using the appium-chromedriver package.
const Chromedriver = require('appium-chromedriver');
const driver = new Chromedriver();
driver.stop().then(() => console.log('Chromedriver stopped successfully'));
Get Chromedriver Status
This feature allows you to get the status of the Chromedriver server. The code sample demonstrates how to retrieve the status using the appium-chromedriver package.
const Chromedriver = require('appium-chromedriver');
const driver = new Chromedriver();
driver.status().then(status => console.log('Chromedriver status:', status));
The selenium-webdriver package is a popular tool for automating web browsers. It provides a high-level API for controlling browsers and is widely used for web application testing. Unlike appium-chromedriver, which is focused on mobile automation, selenium-webdriver supports a broader range of browsers and platforms.
WebdriverIO is a powerful automation framework for web and mobile applications. It provides a rich set of features for browser automation and integrates well with various testing frameworks. Compared to appium-chromedriver, WebdriverIO offers more extensive support for different browsers and testing environments.
Nightwatch is an end-to-end testing framework for web applications and websites. It uses the W3C WebDriver API to perform browser automation and provides a simple syntax for writing tests. While appium-chromedriver is focused on mobile automation, Nightwatch is designed for web application testing and offers built-in support for various browsers.
Node.js wrapper around Chromedriver
This module is written using Traceur which is essentially ECMAscript6 along with the proposed await
command for es7.
import Chromedriver from 'appium-chromedriver';
// 'sync'-like await/Promise usage
async function runSession() {
let driver = new Chromedriver();
const desiredCaps = {browserName: 'chrome'};
await driver.start(desiredCaps);
let status = await driver.sendCommand('/status', 'GET');
await driver.stop();
}
// EventEmitter usage
function runSession2() {
let driver = new Chromedriver();
const desiredCaps = {browserName: 'chrome'};
driver.start(desiredCaps);
driver.on(Chromedriver.EVENT_CHANGED, function (msg) {
if (msg.state === Chromedriver.STATE_ONLINE) {
driver.sendCommand('/status', 'GET').then(function (status) {
driver.stop();
});
}
});
driver.on(Chromedriver.EVENT_ERROR, function (err) {
// :-(
});
}
Here's what the Chromedriver state machine looks like:
Here are the events you can listen for:
Chromedriver.EVENT_ERROR
: gives you an error objectChromedriver.EVENT_CHANGED
: gives you a state change object, with a state
property that can be one of:
Chromedriver.STATE_STOPPED
Chromedriver.STATE_STARTING
Chromedriver.STATE_ONLINE
Chromedriver.STATE_STOPPING
We use Gulp for building/transpiling.
npm run watch
npm test
FAQs
Node.js wrapper around chromedriver.
The npm package appium-chromedriver receives a total of 303,967 weekly downloads. As such, appium-chromedriver popularity was classified as popular.
We found that appium-chromedriver demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.