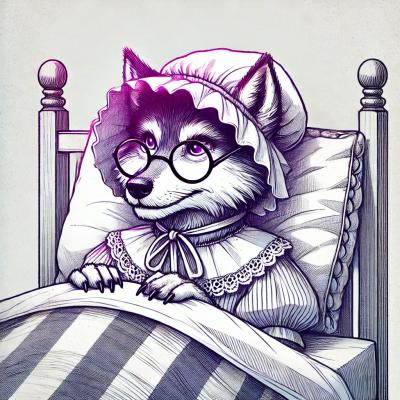
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
appium-idb
Advanced tools
The appium-idb package is a Node.js wrapper for interacting with the iOS Development Bridge (idb), which is a tool developed by Facebook for automating iOS devices. It is primarily used in the context of Appium, a popular automation framework for mobile applications, to facilitate various device and app management tasks.
Install an App
This feature allows you to install an iOS application on a connected device using its UDID. The code sample demonstrates how to connect to the device, install the app, and then disconnect.
const { Idb } = require('appium-idb');
(async () => {
const idb = new Idb({ udid: 'your-device-udid' });
await idb.connect();
await idb.installApp('/path/to/your/app.ipa');
await idb.disconnect();
})();
Launch an App
This feature allows you to launch an installed iOS application on a connected device using its bundle identifier. The code sample shows how to connect to the device, launch the app, and then disconnect.
const { Idb } = require('appium-idb');
(async () => {
const idb = new Idb({ udid: 'your-device-udid' });
await idb.connect();
await idb.launchApp('com.example.yourapp');
await idb.disconnect();
})();
List Installed Apps
This feature allows you to list all the applications installed on a connected iOS device. The code sample demonstrates how to connect to the device, retrieve the list of installed apps, and then disconnect.
const { Idb } = require('appium-idb');
(async () => {
const idb = new Idb({ udid: 'your-device-udid' });
await idb.connect();
const apps = await idb.listApps();
console.log(apps);
await idb.disconnect();
})();
Record Screen
This feature allows you to record the screen of a connected iOS device. The code sample shows how to connect to the device, start the screen recording, and then disconnect.
const { Idb } = require('appium-idb');
(async () => {
const idb = new Idb({ udid: 'your-device-udid' });
await idb.connect();
await idb.recordScreen('/path/to/save/recording.mp4');
await idb.disconnect();
})();
The node-ios-device package provides a Node.js interface for interacting with iOS devices. It allows you to list connected devices, install and uninstall apps, and perform other device management tasks. Compared to appium-idb, it offers a more general-purpose interface for iOS device management but lacks some of the specific automation features provided by appium-idb.
The ios-deploy package is a command-line tool for deploying iOS apps to physical devices. It supports installing, launching, and debugging apps on iOS devices. While it is similar to appium-idb in terms of app deployment capabilities, it is more focused on command-line usage rather than providing a programmatic interface for automation.
The appium-xcuitest-driver package is an Appium driver for automating iOS applications using Apple's XCUITest framework. It provides a comprehensive set of features for iOS app automation, including app installation, launching, and interaction. Compared to appium-idb, it is more focused on end-to-end automation of iOS apps using the XCUITest framework.
appium-idb is NodeJS wrapper over iOS Device Bridge (idb) set of utilities made by Facebook. Read https://www.fbidb.io for more details.
Use the following commands to setup idb and its dependencies:
brew tap facebook/fb
brew install idb-companion
pip3.6 install fb-idb
const idb = IDB({
udid: deviceUdid,
});
await idb.connect();
const deviceInfo = await idb.describeDevice();
await idb.disconnect();
Check https://github.com/appium/appium-idb/blob/master/lib/idb.js on the list of supported IDB options. udid
option is mandatory and can be both Simulator or real device id. It is mandatory to call connect
method before invoking idb instance methods (this will trigger idb companion and idb daemon processes if necessary). Calling disconnect
will stop the previously started companion processes.
Go through the modules in https://github.com/appium/appium-idb/tree/master/lib/tools to get the full list of supported commands.
npm run watch
npm test
FAQs
iOS Debug Bridge interface. NodeJS wrapper of https://www.fbidb.io
We found that appium-idb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.