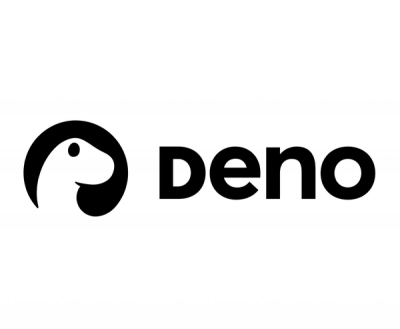
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
apple-reporter
Advanced tools
Promise-based Apple iTunes Connect Reporter for Node.js > 4.2.0.
Results are automagically ungzipped. In Robot.XML
mode (which is default), the XML is parsed into an object using xml2js. Errors result in a Promise rejection with best effort to set the code
and message
properties. Setting the code
is not possible for text mode. (code
defaults to -1)
npm i -S apple-reporter
yarn add apple-reporter
const AppleReporter = require('apple-reporter');
const reporter = new AppleReporter({
userid: 'gy',
password: 'sucks'
});
function getFinanceReport() {
return reporter.Finance.getStatus().then((status) => {
return reporter.Finance.getReport({
vendorNumber: 123456,
regionCode: 'US',
reportType: 'Financial',
fiscalYear: '2015',
fiscalPeriod: '02'
})
.then((report) => {
// do stuff with report...
})
.catch((err) => {
// uh-oh!
console.error('Unable to get Finance report!');
throw err;
});
}, (err) => {
console.error('Finance is down!');
throw err;
});
}
Refer to Apple's documentation for the specifications of each call. All Sales
-based functions are under reporter.Sales
, all Finance
-based functions are under reporter.Finance
options
(object)
baseUrl
: Base endpoint for the API (defaults to https://reportingitc-reporter.apple.com/reportservice
)financeUrl
: Finance endpoint URL (defaults to /finance/v1
)mode
: Either Normal
or Robot.XML
(defaults to Robot.XML
)password
: iTunes Connect account passwordsalesUrl
: Sales endpoint URL (defaults to /sales/v1
)userid
: iTunes Connect account user IDversion
: The API version (defaults to 1.0
)FAQs
Promise-based Apple iTunes Connect Reporter
The npm package apple-reporter receives a total of 27 weekly downloads. As such, apple-reporter popularity was classified as not popular.
We found that apple-reporter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.