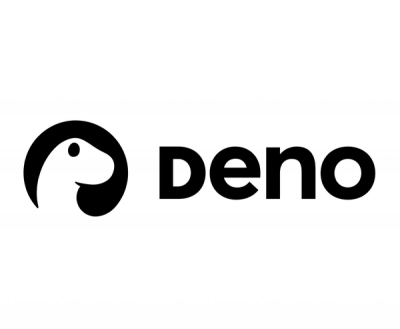
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A small, fast and scalable barebones react state-management library using an easy to understand flux-like principles.
Arachnoid is a small, fast and scalable solution for react based state-management library, using a simplified, easy to understand flux-like principles.
It helps you write applications that are fast and scalable throughout the project, where you can create decentralized and well-modularized states that guarantees consistent behavior throughout the scope of its usage, all the while maintaining its elegant simplicity.
You should have react setup and running
Using vite
npm create vite
Using CRA
npm create react-app
Install Arachnoid using npm
npm install arachnoid
Or Yarn
yarn add arachnoid
import { createStore } from "arachnoid"
const useCountStore = createStore({
state: {
count: 0,
},
actions: {
increment: (get, set) => {
set((state) => ({
...state,
count: state.count + 1,
})
)
}
}
});
Use the hook anywhere, no providers are needed. Dispatch the actions and et'voila! The component will re-render on the changes.
const Test = () => {
const instance1 = useCountStore();
return (
<h1 onClick={() => instance1.dispatch('increment')}>
Hello {instance1.getState().count}
</h1>
)
}
To use deal with asynchronous function, we can use the function createAsyncAction
and use it along with regular archanoid actions.
import { createStore, createAsyncAction } from "arachnoid";
const useAsyncStateStore = createStore({
state: {
todo: [],
},
actions: {
asyncFetch: (get, set, { num }) => createAsyncAction(async ({ num }) => {
const res = await fetch(`https://jsonplaceholder.typicode.com/todos/${num}`);
return await res.json();
}, (asyncResponse) => {
const { data, isLoading, isError } = asyncResponse;
if (!isLoading && !isError) {
set(state => ({
todo: [...state.todo, ...data],
}))
}
}, { num })
}
})
The data returned by the async function can be accessed from asyncResponse
inside the callback function. We can pass any additional require as a payload while dispatching.
We can then dispatch this asynchronous action like we always do!!
const Test = () => {
const instance1 = useAsyncStateStore();
return (<>
<h1 onClick={() => instance1.dispatch('asyncFetch')}>
Add Todo
</h1>
<ol>
{
instance1.getState().todo.map(ele=>(<li>ele.title</li>));
}
</ol>
</>)
}
Arachnoid provides bare-bones middleware support for its stores using createArachnoidMiddleware
function.
import { createStore, createArachnoidMiddleware } from "arachnoid";
const middleware1 = createArachnoidMiddleware((get, set, action)=>{
console.log (`${action.name} has been called with payload ${JSON.stringify(action.payload)}`);
})
export const store = createStore({
state: {
count: 0,
},
actions: {
increment: (get, set) => {
set((state) => ({
...state,
count: state.count + 1,
})
)
}
}
}, [middleware1]);
We can prevent certain actions from using middlewares by passsing ignore actions array to createArrachnoidMiddleware
.
import { createStore, createArachnoidMiddleware } from "arachnoid";
const middleware1 = createArachnoidMiddleware((get, set, action)=>{
console.log (`${action.name} has been called with payload ${JSON.stringify(action.payload)}`);
}, ["decrement"])
export const store = createStore({
state: {
count: 0,
},
actions: {
increment: (get, set) => {
set((state) => ({
...state,
count: state.count + 1,
})
)
},
decrement: (get, set) => {
set((state) => ({
...state,
count: state.count - 1,
})
)
}
}
}, [middleware1]);
Here all the actions except decrement will execute the middleware1
.
Arachnoid providers bare-bones support for listeners subscribing to the state changes.
We can define listeners while creating the store.
const store = createStore({
state: {
count: 0,
},
actions: {
increment: (get, set) => {
set({
...get(),
count: get().count + 1,
})
}
},
listeners: {
test: (get)=>{
console.table(get())
}
}
});
In here the listener test
will listen to all the state changes, and execute itself then.
OR
We can also subscribe or unsubscribe to a listener inside a component.
const Test = () => {
const instance1 = store();
useEffect(()=>{
instance.subscribe("test2", get=>console.log(get()))
instance.unsubscribe('test');
}, [])
const handleClick = () => instance2.dispatch('increment')
return (
<h1 onClick={handleClick}>
Hello {instace1.getState().count} {instance2.getState().count}
</h1>
)
}
See also the list of contributors who participated in this project.
FAQs
Unknown package
The npm package arachnoid receives a total of 1 weekly downloads. As such, arachnoid popularity was classified as not popular.
We found that arachnoid demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.