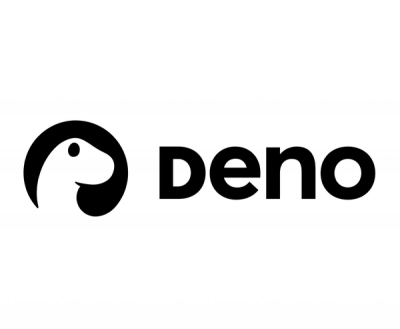
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
arctic
Library for handling OAuth 2.0 with built-in providers. Light weight, fully-typed, runtime-agnostic. Built using oslo
. For a more flexible OAuth 2.0 client, see oslo/oauth2
.
npm install arctic
See OAuth 2.0 providers for instructions.
See OAuth 2.0 providers with PKCE for instructions.
Most providers require the client_id
and client_secret
. You may also optionally pass scope
. For OIDC clients, openid
and profile
scope are always included.
import { GitHub } from "arctic";
const github = new GitHub(clientId, clientSecret, {
scope: ["user:email"] // etc
});
Some providers also require the redirect URI.
import { Google } from "arctic";
const redirectURI = "http://localhost:3000/login/google/callback";
const google = new Google(clientId, clientSecret, redirectURI);
Generate state using generateState()
and store it as a cookie. Use it to create an authorization URL with createAuthorizationURL()
and redirect the user to it.
import { generateState } from "arctic";
const state = generateState();
const url = await github.createAuthorizationURL(state);
// store state as cookie
setCookie("state", state, {
secure: true, // set to false in localhost
path: "/",
httpOnly: true,
maxAge: 60 * 10 // 10 min
});
return redirect(url);
Compare the state, and use validateAuthorizationCode()
to validate the authorization code. This returns an object with an access token, and a refresh token if requested. If the code is invalid, it will throw an AccessTokenRequestError
.
import { OAuth2RequestError } from "arctic";
const code = request.url.searchParams.get("code");
const state = request.url.searchParams.get("state");
const storedState = getCookie("state");
if (!code || !storedState || state !== storedState) {
// 400
throw new Error("Invalid request");
}
try {
const tokens = await github.validateAuthorizationCode(code);
} catch (e) {
if (e instanceof OAuth2RequestError) {
const { message, description, request } = e;
}
// unknown error
}
See also:
Most providers require the client_id
and client_secret
. You may also optionally pass scope
. For OIDC clients, openid
and profile
scope are always included.
import { GitHub } from "arctic";
const github = new GitHub(clientId, clientSecret, {
scope: ["user:email"] // etc
});
Some providers also require the redirect URI.
import { Google } from "arctic";
const redirectURI = "http://localhost:3000/login/google/callback";
const github = new GitHub(clientId, clientSecret, redirectURI);
When using the PKCE flow, state
is not necessary. Generate a code verifier using generateCodeVerifier()
, and store it as a cookie. Use them to create an authorization URL with createAuthorizationURL()
and redirect the user to it.
import { generateState, generateCodeVerifier } from "arctic";
const codeVerifier = generateCodeVerifier();
const url = await github.createAuthorizationURL(state, codeVerifier);
// store code verifier as cookie
setCookie("code_verifier", state, {
secure: true, // set to false in localhost
path: "/",
httpOnly: true,
maxAge: 60 * 10 // 10 min
});
return redirect(url);
Use validateAuthorizationCode()
to validate the authorization code with the code verifier. This returns an object with an access token, and a refresh token if requested. If the code is invalid, it will throw an AccessTokenRequestError
.
import { OAuth2RequestError } from "arctic";
const code = request.url.searchParams.get("code");
const codeVerifier = request.url.searchParams.get("code_verifier");
try {
const tokens = await github.validateAuthorizationCode(code, codeVerifier);
} catch (e) {
if (e instanceof OAuth2RequestError) {
// see https://oslo.js.org/reference/oauth2/OAuth2RequestError/
const { request, message, description } = e;
}
// unknown error
}
See also:
Some providers expose getUser()
to get the current user from an access token. This will throw an RequestError
if the access token is invalid.
import { RequestError } from "arctic";
try {
const user = await github.getUser(tokens.accessToken);
} catch (e) {
if (e instanceof RequestError) {
// get fetch Request and Response
const { request, response } = e;
}
// unknown error
}
Some providers expose refreshAccessToken()
to get a new access token from a refresh token. This will throw an OAuth2RequestError
if the refresh token is invalid.
import { OAuth2RequestError } from "arctic";
try {
const tokens = await google.refreshAccessToken(refreshToken);
} catch (e) {
if (e instanceof OAuth2RequestError) {
// see https://oslo.js.org/reference/oauth2/OAuth2RequestError/
const { request, message, description } = e;
}
// unknown error
}
FAQs
OAuth 2.0 clients for popular providers
The npm package arctic receives a total of 47,419 weekly downloads. As such, arctic popularity was classified as popular.
We found that arctic demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.