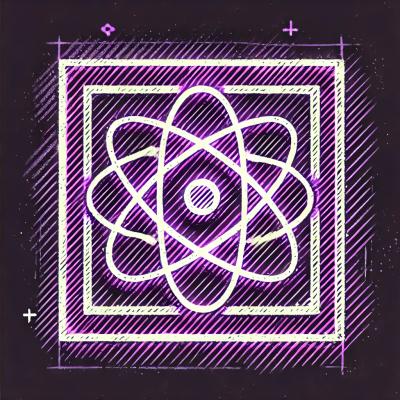
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Node.js project
Version: 0.1.3
Inspired by the extremly well-known argp C library, this module parses GNU-style command-line options. Help, usage and version messages are automatically generated and line-wrapped at 80 columns. The module checks for errors, can be easily adapted to your needs thanks to its evented system and it also works when Node.js is in debug mode. The module is uncached and each property is deleted once all the input parameters have been parsed, so there's no memory footprint.
This module it's made for you if you want:
A common configuration looks like this:
//If you can, don't cache the module
var argv = require ("argp")
.description ("Sample app.")
.email ("a@b.c")
.body ()
//The object and argument definitions and the text of the --help message
//are configured at the same time
.text (" Arguments:")
.argument ("arg", { description: "Sample argument" })
.text ("\n Options:")
.option ({ short: "o", long: "opt", description: "Sample option" })
.help ()
.version ("v1.2.3")
.argv ();
console.log (argv);
/*
$ node script.js
{
opt: false,
help: false,
version: false,
arg: false
}
$ node script.js --help
Usage: script [options] [arguments]
Sample app.
Arguments:
arg Sample argument
Options:
-o, --opt Sample option
-h, --help Display this help message and exit
-v, --version Output version information and exit
Report bugs to <a@b.c>.
*/
If you have a package.json
file you can take from it the description, email and version using the readPackage()
function. Take into account that this function calls a synchronous fs
operation. Doesn't really matter because this module is one of the first things you're going to execute in your program.
var argv = require ("argp")
//If no path is provided, it tries to read the "./package.json" file
.readPackage ("path/to/package.json")
.body ()
.text (" Arguments:")
.argument ("arg", { description: "Sample argument" })
.text ("\n Options:")
.option ({ short: "o", long: "opt", description: "Sample option" })
.help ()
.argv ();
npm install argp
Quick examples with no configuration
If an option has not been defined the type of the value is converted automatically from a string to a number, boolean, null or undefined.
By default the parser doesn't allow undefined arguments and options because you typically want to have an absolute control over the calls to your program in order to avoid unexpected behaviours. Allowing undefined arguments and options is as simple as this:
var argv = require ("argp")
.allowUndefinedArguments ()
.allowUndefinedOptions ()
.argv ();
$ node script.js -a -b -c
{ a: true, b: true, c: true }
$ node script.js a b c
{ a: true, b: true, c: true }
$ node script.js -abc null
{ a: true, b: true, c: null }
$ node script.js --a --b 1 --d=e
{ a: true, b: 1, d: "e" }
$ node script.js --no-a b
{ a: false, b: true }
$ node script.js --a -- -b --c d
{ a: true, "-b": true, "--c": true, d: true }
Considerations:
By default the options are flags. If the option requires a value, the metavar
property must be defined. This property is a string and can be seen when the --help and --usage messages are printed.
$ node script.js --help
...
o, --opt=STR Sample option
...
Where STR
is the metavar
property.
By default, the value of the options is a string. Configure the type
property if the value is a number, boolean (rarely used, use a flag instead) or array (comma-separated values and multiple assignments).
Each option has an id which is used to store the value into the final object. This id is the long name. If the long name has not been defined then the id is the short name.
.option ({ short: "a", long: "aa" })
//{ aa: false }
.option ({ long: "aa" })
//{ aa: false }
.option ({ short: "a" })
//{ a: false }
Mandatory options (aka required
) are not implemented because options are optional. Use a command if you need mandatory parameters.
Common properties between flags and options with value:
Flags:
negate - Boolean
If true, the flag is negated.
.option ({ short: "o", long: "opt", negate: true })
$ node script.js
{ opt: true }
$ node script.js --opt
{ opt: true }
$ node script.js --no-opt
{ opt: false }
$ node script.js -o
{ opt: false }
Options with value:
aliases - Array
An alias it's a long-name option that points to another option.
.body ()
.option ({ long: "name", aliases: ["foo", "bar"] })
.help ()
.usage ()
$ node script.js --foo
{ name: true }
$ node script.js --usage
Usage: script [--name|--foo|--bar] [-h|--help] [--usage]
$ node script.js --help
Usage: script [options]
--name, --foo, --bar
-h, --help Display this help message and exit
--usage Display a short usage message and exit
The options()
function returns an object with all the configured options:
{
name: { ... },
foo: { ... },
bar: { ... },
help: { ... },
usage: { ... }
}
Where name
, foo
and bar
point to the same object:
.on ("start", function (){
var options = this.options ();
assert.ok (options.name === options.foo && options.name === options.bar);
})
choices - Array
The input value must be one of these choices. If the option is optional
, the choices
property is ignored.
.option ({ long: "opt", metavar: "NUM", type: Number, choices: [1, 2, 3] })
$ node script.js --opt=1
{ opt: 1 }
$ node script.js --opt=7 # Error!
When default
and choices
are configured in the same option the default value doesn't need to match any choice:
.option ({ long: "opt", metavar: "STR", default: "d", choices: ["a", "b", "c"] })
$ node script.js
{ opt: "d" }
default - Object
The default value.
.option ({ long: "name", metavar: "STR", default: "bar", optional: true })
$ node script.js
{ name: "bar" }
$ node script.js --name
{ name: "bar" }
$ node script.js --name foo
{ name: "foo" }
metavar - String
Must be configured if the option requires a value. The string is used when the --help and --usage messages are printed.
.option ({ long: "name", metavar: "STR" })
$ node script.js --help
...
--name=STR
...
optional - Boolean
If true, the value is optional. Default is false. If the option doesn't receive any value the default value is set and it depends on the default
and type
properties.
Types and default values:
String: null
Number: 0
Array: []
Boolean: false
.option ({ long: "name1", metavar: "STR", optional: true })
.option ({ long: "name2", metavar: "NUM", optional: true, type: Number })
.option ({ long: "name3", metavar: "ARR", optional: true, type: Array })
//Boolean type is rarely used, use a flag instead
.option ({ long: "name4", metavar: "BOOL", optional: true, type: Boolean })
$ node script.js --name1 --name2 --name3 --name4
{ name1: null, name2: 0, name3: [], name4: false }
$ node script.js --name1 foo --name2 12 --name3 -12.34,foo,true --name3 true --name4 true
{ name1: "foo", name2: 12, name3: [-12.34, "foo", true], name4: true }
reviver - Function
The function is executed when the option is parsed. It is similar to the reviver of the JSON.parse()
function. This is the right place where you can validate the input data and fail()
if it's not valid. For example, if the option requires a number you can validate the range here.
.option ({ long: "name", metavar: "STR", reviver: function (value){
return value + "bar";
}})
$ node script.js --name foo
{ name: "foobar" }
.option ({ long: "opt", metavar: "NUM", type: Number,
reviver: function (value){
//"value" is already a number
if (value < 1 || value > 3){
this.fail ("Option 'opt': invalid range");
}
return value;
}})
type - String | Number | Boolean | Array
The type of the value. Default is String.
If the type is an Array, comma-separated values are automatically stored into an array and each element is converted to the type it represents. Multiple assignments are also supported.
.option ({ long: "name", metavar: "ARR", type: Array })
$ node script.js --name 1,true,foo
{ name: [1, true, "foo"] }
$ node script.js --name 1 --name true --name foo
{ name: [1, true, "foo"] }
$ node script.js --name 1,2 --name true,false --name foo,bar
{ name: [1, 2, true, false, "foo", "bar"] }
Example: options.js.
An argument is an individual name like login
, reset
, build
, etc. They are basically flags.
Properties:
Note: help
and trailing
properties can be also configured but they are related with the commands.
.argument ("arg1")
.argument ("arg2", { description: "foo" })
.argument ("arg3", { description: "bar", hidden: true })
$ node script.js arg1
{ arg1: true, arg2: false, arg3: false }
$ node script.js --help
...
arg1 foo
arg2 bar
...
Example: arguments.js.
A command is an argument followed by other arguments and options. NPM is an example:
npm config set <key> [<value>]
npm install [<package>...] -g
config
is a command and set
an argument with 2 trailing arguments: minimum 1, maximum 2.
install
is a command with infinite trailing arguments: minimum 0, maximum Infinity. -g
is an option which only applies to the install
command.
If you have a very few commands you can configure them in the same file (commands.js example), but you typically want to modularize them, one command per file. Then you should check the npm.js example.
The commands are configured exactly the same way as the Argp
instance with only one difference: argument()
accepts 2 new properties:
synopsis - String
The string replaces the argument name in the --help and --usage messages.
.argument ("set", { description: "Sample argument" });
/*
...
set Sample argument
...
*/
.argument ("set", { synopsis: "set <key> [<value>]", description: "Sample argument" });
/*
...
set <key> [<value>] Sample argument
...
*/
trailing - Object
Configures how many trailing arguments must follow this argument.
There are 3 properties: eq
, min
and max
. eq
cannot be used with min
or max
. If min
and max
are being used, by default min
is 0 and max
is Infinity. A trailing
object without any of these 3 properties defaults to min
0 and max
Infinity.
Some examples:
2 trailing arguments required: cmd arg <x> <x>
.
.argument ("arg", { trailing: { eq: 2 } })
1 required, 1 optional: cmd arg <x> [<x>]
.
.argument ("arg", { trailing: { min 1, max: 2 } })
1 optional: cmd arg [<x>]
.
.argument ("arg", { trailing: { max: 1 } })
1 required, infinite optional: cmd arg <x> [<x>...]
.
.argument ("arg", { trailing: { min: 1 } })
Infinite: cmd arg [<x>...]
.
.argument ("arg", { trailing: {} })
No trailing arguments: cmd arg
.
.argument ("arg")
Multiple arguments with trailing in the same line. Argument arg1
with 1 required, and argument arg2
with infinite trailing arguments: cmd arg1 <x> arg2 [<y>...]
.
.argument ("arg1", { trailing: { eq: 1 } })
.argument ("arg2", { trailing: {} })
The module returns an instance of Argp
. It inherits from an EventEmitter.
The parser follows the GNU-style rules: -a
, -abc
, --a
, --no-a
, --a=b
, --
, etc. Long option abbreviation is also supported.
If you don't want to configure anything simply require the module, allow undefined arguments and options and call to argv()
.
var argv = require ("argp")
.allowUndefinedArguments ()
.allowUndefinedOptions ()
.argv ();
Note: If no configuration is provided you cannot join a short name with its value in the same token, eg: -Dvalue
, all the characters following a dash, -
, are interpreted as individual flags.
Events
With the event system you can fully adapt this module to yours needs. Examples: to-upper-case.js, mkdir.js.
Methods
Objects
Emitted when an argument is found.
Parameters:
Emitted when all the options and arguments have been parsed. The following 3 functions can be cached, they are safe to use at any time, they won't introduce any memory leak.
Parameters:
Emitted when an option is found.
Parameters:
Emitted just before the parser begins to read the input data.
Parameters:
Argp#allowUndefinedArguments() : Argp
Allows undefined arguments.
Argp#allowUndefinedOptions() : Argp
Allows undefined options.
Returns the configured arguments. Look at the internal-data.js example for further details.
Parses the process.argv
array. The module is removed from the cache and all its properties are deleted, so even if you store the module in a variable (var argp = require ("argp")
) and forget to free it (argp = null
) you won't introduce any memory leak, just an empty object ({}
) will remain in memory.
You can also pass an array with the arguments and options, but then the module won't be freed, so you can reuse the configuration.
var argp = require ("argp");
//Configuration
argp
.description ()
...
var argv;
argv = argp.argv (["-a", "--b", ...]);
argv = argp.argv (["c", "d", ...]);
Returns a Body
instance.
Sets the maximum line length. By default lines are wrapped at 80 columns.
Argp#command(name[, configuration]) : Command
Configures a command. A command it's like a new fresh cli program. It behaves exactly like an Argp
. See Configuring commands.
Returns the configured commands.
Look at the internal-data.js example for further details.
Sets a description. The description is printed at the start of the --help message, after the "Usage" lines.
Sets a contact email. The email is printed at the end of the --help message.
Sets the exit code when the process finishes due to an error. Default is 1.
Argp#fail(str[, code]) : undefined
Prints a message to the stderr and exits with code code
, the one configured with exitStatus() or 1.
Returns de main Argp
instance. It's a no-op function, just for a better visual organization when configuring commands.
Look at the npm.js example for further details.
Argp#options([filter]) : Object
Returns the configured options. filter
is an object which can be used to return the options with a short name or with a long name.
.options ()
.options ({ short: true })
.options ({ long: true })
Look at the internal-data.js example for further details.
Argp#readPackage([path]) : Argp
Reads a package.json
file and configures the parser with the description, email and version. If no path is provided it tries to read the ./package.json
path.
Changes the "Usage" line from the --help and --usage messages. usages
is an array of strings.
Look at the custom-usages.js example for further details.
If sort()
is enabled, the options are parsed before the arguments, if not, the options and arguments are parsed in the same order they come.
The Body
instance is returned by Argp#body(). All the following functions print a message in the same order they are configured, this allows you to fully customize the --help message very easily.
Look at fully-descriptive-help.js for further details.
Methods
Body#argument(name[, configuration]) : Body
Defines an argument. See Configuring arguments.
Same as Argp#argv().
Body#columns(column1, column2) : Body
Prints a line with 2 columns. The first column shouldn't contain line breaks (\n
). This functionality is used to print the options and arguments.
Body#command(name[, configuration]) : Command
Same as Argp#command().
Enables the -h, --help
option. The short option can be disabled using the options
parameter.
.help ({ short: false })
$ node script.js --help
...
--help Display this help message and exit
...
Same as Argp#main().
Defines an option. See Configuring options.
Body#text(str[, prefix]) : Body
Prints a text message. By default it's line-wrapped at 80 columns and supports multilines (line breaks, \n
). The prefix
is mainly used to indent the text with some spaces.
Enables the --usage
option.
Body#version(str[, options]) : Body
Enables the -v, --version
option. str
is the text to print when the option is called. The short option can be disabled using the options
parameter.
.version ("v1.2.3", { short: false })
$ node script.js --help
...
--version Output version information and exit
...
FAQs
Command-line option parser
The npm package argp receives a total of 419 weekly downloads. As such, argp popularity was classified as not popular.
We found that argp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.