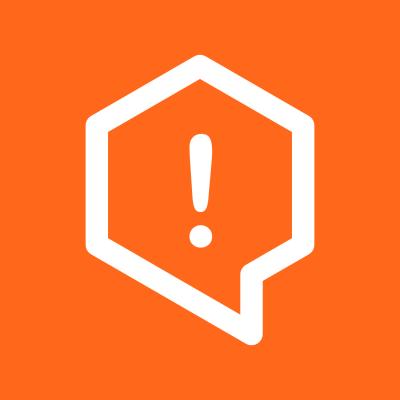
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
argv-to-object
Advanced tools
Maps command-line arguments to a configuration object.
$ npm install argv-to-object
var argv = require( 'argv-to-object' );
Maps command-line arguments to a configuration object
.
var map = {
'env': {
'keypath': 'argv',
'type': 'string',
'default': 'dev'
},
'port': {
'keypath': 'server.port',
'type': 'integer',
'default': 8080,
'alias': [
'p'
],
'radix': 10
},
'ssl': {
'keypath': 'server.ssl',
'type': 'boolean'
},
'loglevel': {
'keypath': 'logger.level',
'type': 'string',
'default': 'info'
}
};
// --env=test --port 7331 --ssl --loglevel debug
var out = argv( map );
/*
{
'env': 'test',
'server': {
'port': 7331,
'ssl': true
},
'logger': {
'level': 'debug'
}
}
*/
A command-line argument mapping must include a keypath
, which is a dot-delimited object
path. By default, this module parses a command-line argument value as a string
. The following types are supported:
The function
accepts the following options
:
parsers: an object
containing command-line argument parsers. Each key
should correspond to a defined type
, and each value
should be a function
which accepts a command-line argument value and any associated options
.
var map = {
'custom_type': {
'keypath': 'custom',
'type': 'custom',
... // => options
}
};
var parsers = {
'custom_type': custom
};
function custom( str, opts ) {
var v = parseInt( str, 10 );
if ( v !== v ) {
return new TypeError( 'invalid value. Value must be an integer. Value: `' + str + '`.' );
}
return v * 6;
}
// --custom_type=5
var out = argv( map, parsers );
/*
{
'custom': 30
}
*/
All command-line argument value types
support the following options
:
array
of command-line argument aliases.Except for boolean
, all command-line argument value types
support the following options
:
boolean
indicating whether a command-line argument can be provided multiple times. If true
, the command-line argument value is always an array
containing values of a specified type
. Default: false
.===
Coerce a command-line argument value to a string
.
var map = {
'str': {
'keypath': 'str',
'type': 'string'
}
};
// --str=beep
var out = argv( map );
/*
{
'str': 'beep'
}
*/
// --str=1234
var out = argv( map );
/*
{
'str': '1234'
}
*/
===
Coerce a command-line argument value to a number
.
var map = {
'num': {
'keypath': 'num',
'type': 'number'
}
};
// --num='3.14'
var out = argv( map );
/*
{
'num': 3.14
}
*/
// --num=bop
var out = argv( map );
// => throws
===
Coerce a command-line argument value to an integer
.
var map = {
'int': {
'keypath': 'int',
'type': 'integer'
}
};
// --int=2
var out = argv( map );
/*
{
'int': 2
}
*/
// --int=beep
var out = argv( map );
// => throws
The integer
type supports the following options
:
radix: an integer
on the interval [2,36]
. Default: 10
.
var map = {
'int': {
'keypath': 'int',
'type': 'integer',
'radix': 2
}
};
// --int=1
var out = argv( map );
/*
{
'int': 1
}
*/
// --int=2
var out = argv( map );
// => throws
===
Coerce a command-line argument value to a boolean
.
var map = {
'bool': {
'keypath': 'bool',
'type': 'boolean'
}
};
// --bool
var out = argv( map );
/*
{
'bool': true
}
*/
Prefixing --no-*
to a boolean
command-line argument will override a default
value.
var map = {
'bool': {
'keypath': 'bool',
'type': 'boolean',
'default': true
}
};
// --no-bool
var out = argv( map );
/*
{
'bool': false
}
*/
If a boolean
command-line argument does not have a default
value, using the --no-*
prefix is equivalent to not specifying the command-line argument.
var map = {
'bool': {
'keypath': 'bool',
'type': 'boolean'
}
};
// --no-bool
var out = argv( map );
/*
{}
*/
===
Parse a command-line argument value as a JSON object
. Note that a value must be valid JSON.
var map = {
'obj': {
'keypath': 'obj',
'type': 'object'
}
};
// --obj='{"beep":"boop"}'
var out = argv( map );
/*
{
'obj': {
'beep': 'boop'
}
}
*/
// --obj='[1,2,3,"4",null]'
var out = argv( map );
/*
{
'obj': [ 1, 2, 3, '4', null ]
}
*/
// --obj='{"beep:"boop"}'
var out = argv( map );
// => throws
===
Coerce a command-line argument to a Date
object.
var map = {
'date': {
'keypath': 'date',
'type': 'date'
}
};
// --date='2015-10-17'
var out = argv( map );
/*
{
'date': <Date>
}
*/
// --date=beep
var out = argv( map );
// => throws
===
Parse a command-line argument as a RegExp
.
var map = {
'regexp': {
'keypath': 're',
'type': 'regexp'
}
};
// --regexp='/\\w+/'
var out = argv( map );
/*
{
're': /\w+/
}
*/
// --regexp=beep
var out = argv( map );
// => throws
If a command-line argument does not exist and no default value is specified, the corresponding configuration keypath
will not exist in the output object
.
var map = {
'unset_argv': {
'keypath': 'a.b.c'
}
};
var out = argv( map );
// returns {}
var argv = require( 'argv-to-object' );
var map = {
'env': {
'keypath': 'env',
'default': 'dev'
},
'port': {
'keypath': 'server.port',
'type': 'integer',
'default': 8080,
'alias': [
'p'
],
'radix': 10
},
'ssl': {
'keypath': 'server.ssl',
'type': 'boolean'
},
'loglevel': {
'keypath': 'logger.level',
'type': 'string',
'default': 'info'
},
'num': {
'keypath': 'num',
'type': 'number'
},
'obj': {
'keypath': 'obj',
'type': 'object'
},
'arr': {
'keypath': 'arr',
'type': 'object'
},
'bool': {
'keypath': 'bool',
'type': 'boolean'
},
'nested': {
'keypath': 'a.b.c.d',
'type': 'object'
},
'date': {
'keypath': 'date',
'type': 'date'
},
'regex': {
'keypath': 're',
'type': 'regexp'
},
'mnum': {
'keypath': 'mnum',
'type': 'integer',
'multiple': true
}
};
// --env=test --ssl --p 7331 --num='3.14' --obj='{"hello":"world"}' --arr='[1,2,3,4]' --nested='{"beep":"boop"}' --date="2015-10-17" --regex '/\\w+/' --no-bool --mnum 1 --mnum=2 --mnum=3 --mnum 4
var out = argv( map );
/*
{
'env': 'test',
'server': {
'ssl': true,
'port': 7331
},
'logger': {
'level': 'info'
},
'num': 3.14,
'obj': {
'hello': 'world'
},
'arr': [ 1, 2, 3, 4 ],
'nested': {
'a': {
'b': {
'c': {
'd': {
'beep': 'boop'
}
}
}
}
},
'date': <Date>,
're': /\w+/,
'mnum': [ 1, 2, 3, 4 ]
}
*/
To run the example code from the top-level application directory,
$ node ./examples/index.js --env=test --ssl --p 7331 --num='3.14' --obj='{"hello":"world"}' --arr='[1,2,3,4]' --nested='{"beep":"boop"}' --date="2015-10-17" --regex '/\\w+/' --no-bool --mnum 1 --mnum=2 --mnum=3 --mnum 4
Unit tests use the Mocha test framework with Chai assertions. To run the tests, execute the following command in the top-level application directory:
$ make test
All new feature development should have corresponding unit tests to validate correct functionality.
This repository uses Istanbul as its code coverage tool. To generate a test coverage report, execute the following command in the top-level application directory:
$ make test-cov
Istanbul creates a ./reports/coverage
directory. To access an HTML version of the report,
$ make view-cov
Copyright © 2015. Athan Reines.
FAQs
Maps command-line arguments to a configuration object.
The npm package argv-to-object receives a total of 43 weekly downloads. As such, argv-to-object popularity was classified as not popular.
We found that argv-to-object demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.