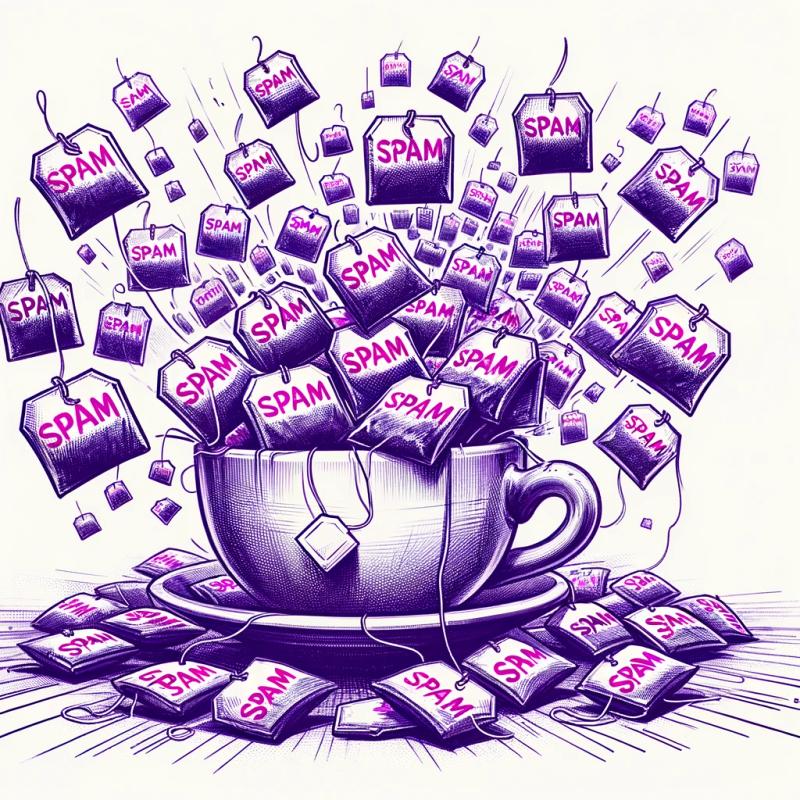
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
array.prototype.flatmap
Advanced tools
Package description
The array.prototype.flatmap package provides a polyfill for the flatMap method that was added to the Array prototype in ECMAScript 2019. This method allows you to map over an array and flatten the result by one level in a single operation. It is particularly useful for dealing with arrays of arrays or when applying a function that returns an array for each element.
Mapping and Flattening
This code demonstrates how to use flatMap to map over an array of arrays, doubling each element, and then flattening the result into a single array. The output would be [2, 4, 6, 8].
[[1, 2], [3, 4]].flatMap(x => x.map(y => y * 2))
Filtering and Mapping in One Step
This example shows how flatMap can be used to filter and map over an array simultaneously. It doubles the even numbers and removes the odd numbers, resulting in [4, 8].
[1, 2, 3, 4].flatMap(x => x % 2 === 0 ? [x * 2] : [])
Lodash's flatten method provides similar functionality to flatMap for flattening arrays, but it does not include mapping. You would need to chain it with map for similar effects, making array.prototype.flatmap more convenient for combined operations.
Underscore.js offers methods like _.flatten and _.map which can be combined to achieve similar results to flatMap. However, like lodash, this requires chaining multiple operations, whereas array.prototype.flatmap integrates both into one method.
Readme
An ESnext spec-compliant Array.prototype.flatMap
shim/polyfill/replacement that works as far down as ES3.
This package implements the es-shim API interface. It works in an ES3-supported environment and complies with the proposed spec.
Because Array.prototype.flatMap
depends on a receiver (the this
value), the main export takes the array to operate on as the first argument.
npm install --save array.prototype.flatmap
var flatMap = require('array.prototype.flatmap');
var assert = require('assert');
var arr = [1, [2], [], 3];
var results = flatMap(arr, function (x, i) {
assert.equal(x, arr[i]);
return x;
});
assert.deepEqual(results, [1, 2, 3]);
var flatMap = require('array.prototype.flatmap');
var assert = require('assert');
/* when Array#flatMap is not present */
delete Array.prototype.flatMap;
var shimmedFlatMap = flatMap.shim();
var mapper = function (x) { return [x, 1]; };
assert.equal(shimmedFlatMap, flatMap.getPolyfill());
assert.deepEqual(arr.flatMap(mapper), flatMap(arr, mapper));
var flatMap = require('array.prototype.flatmap');
var assert = require('assert');
/* when Array#flatMap is present */
var shimmedIncludes = flatMap.shim();
var mapper = function (x) { return [x, 1]; };
assert.equal(shimmedIncludes, Array.prototype.flatMap);
assert.deepEqual(arr.flatMap(mapper), flatMap(arr, mapper));
Simply clone the repo, npm install
, and run npm test
FAQs
An ES2019 spec-compliant `Array.prototype.flatMap` shim/polyfill/replacement that works as far down as ES3.
The npm package array.prototype.flatmap receives a total of 17,055,286 weekly downloads. As such, array.prototype.flatmap popularity was classified as popular.
We found that array.prototype.flatmap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.