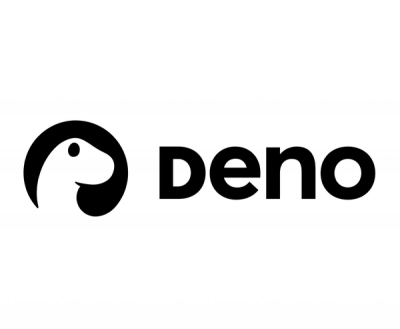
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A JavaScript client (for both Node and browser) for the Asana API v1.0.
Install with npm:
npm install asana --save
Include the latest release directly from GitHub.
<script src="https://github.com/Asana/node-asana/releases/download/<LATEST_RELEASE>/asana-min.js"></script>
OR
SCRIPT
tag.nodeify
which
takes a callback as parameter. For generators and streams, co and
highland also support promises respectively. Beyond that, other
major libraries such as mongoose, mocha, and elastic search (which uses
bluebird) also support promises.To do anything, you'll need always an instance of an Asana.Client
configured
with your preferred authentication method (see the Authentication section below
for more complex scenarios) and other options.
The most minimal example would be as follows:
var asana = require('asana');
var client = asana.Client.create().useAccessToken('my_access_token');
client.users.me().then(function(me) {
console.log(me);
});
All resources are exposed as properties of the Asana.Client
instance (e.g. client.workspaces
). See the developer documentation for docs on each of them.
This module supports authenticating against the Asana API with either a Personal Access Token or through OAuth 2.0.
var client = Asana.Client.create().useAccessToken('personal_access_token');
Authenticating through OAuth2 is preferred. There are many ways you can do this.
In all cases, you should create a Client
that contains your app information. The values in the below snippet should be substituted with the real properties from your application's settings.
var client = Asana.Client.create({
clientId: 123,
clientSecret: 'my_client_secret',
redirectUri: 'my_redirect_uri'
});
If you have a plain bearer token obtained somewhere else and you don't mind not having your token auto-refresh, you can authenticate with it as follows:
client.useOauth({
credentials: 'my_access_token'
});
If you obtained a refresh token (from a previous authorization), you can use it together with your client credentials to authenticate:
var credentials = {
// access_token: 'my_access_token',
refresh_token: 'my_refresh_token'
};
client.useOauth({
credentials: credentials
});
See examples/oauth/webserver
for a working example of this.
Whenever you ask for a collection of resources, you will receive a Collection
object which gives you access to a page of results at a time. You can provide
a number of results per page to fetch, between 1 and 100. If you don't provide
any, it defaults to 50.
client.tasks.findByTag(tagId, { limit: 5 }).then(function(collection) {
console.log(collection.data);
// [ .. array of up to 5 task objects .. ]
});
Additionally, Collection
has a few useful methods that can make them
more convenient to deal with.
To get the next page of a collection, you do not have to manually construct
the next request. The nextPage
method takes care of this for you:
client.tasks.findByTag(tagId).then(function(firstPage) {
console.log(firstPage.data);
collection.nextPage().then(function(secondPage) {
console.log(secondPage.data);
});
});
To automatically fetch a bunch of results and have the client transparently
request pages under the hood, use the fetch
method.:
client.tasks.findByTag(tagId).then(function(collection) {
// Fetch up to 200 tasks, using multiple pages if necessary
collection.fetch(200).then(function(tasks) {
console.log(tasks);
});
});
You can also construct a stream
from a collection. This will transparently
(and lazily) fetch the items in the collection in pages as you iterate
through them.
client.tasks.findByTag(tagId).then(function(collection) {
collection.stream().on('data', function(task) {
console.log(task);
});
});
In any request against the Asana API, there a number of errors that could
arise. Those are well documented in the Asana API Documentation, and
are represented as exceptions under the namespace Asana.errors
.
Various examples are in the repository under examples/
, but some basic
concepts are illustrated here.
var Asana = require('asana');
var util = require('util');
// Using the API key for basic authentication. This is reasonable to get
// started with, but Oauth is more secure and provides more features.
var client = Asana.Client.create().useBasicAuth(process.env.ASANA_API_KEY);
client.users.me()
.then(function(user) {
var userId = user.id;
// The user's "default" workspace is the first one in the list, though
// any user can have multiple workspaces so you can't always assume this
// is the one you want to work with.
var workspaceId = user.workspaces[0].id;
return client.tasks.findAll({
assignee: userId,
workspace: workspaceId,
completed_since: 'now',
opt_fields: 'id,name,assignee_status,completed'
});
})
.then(function(response) {
// There may be more pages of data, we could stream or return a promise
// to request those here - for now, let's just return the first page
// of items.
return response.data;
})
.filter(function(task) {
return task.assignee_status === 'today' ||
task.assignee_status === 'new';
})
.then(function(list) {
console.log(util.inspect(list, {
colors: true,
depth: null
}));
});
The code is thoroughly documented with JsDoc tags. The Official Asana Documentation is a great resource since this is just a thin wrapper for the API.
Feel free to fork and submit pull requests for the code! Please follow the existing code as an example of style and make sure that all your code passes lint and tests. For a sanity check:
git clone git@github.com:Asana/node-asana.git
cd node-asana
npm install
npm test
The specific Asana resource classes (Tag
, Workspace
, Task
, etc) are
generated code, hence they shouldn't be modified by hand. See the asana-api-meta repo for details.
Repo Owners Only. Take the following steps to issue a new release of the library.
master
branch and commit them.gulp bump-patch
, gulp bump-feature
, or gulp bump-release
git push origin master --tags
Travis CI will automatically build and deploy the tagged release.
FAQs
This_is_the_interface_for_interacting_with_the__Asana_Platform_httpsdevelopers_asana_com__Our_API_reference_is_generated_from_our__OpenAPI_spec__httpsraw_githubusercontent_comAsanaopenapimasterdefsasana_oas_yaml_
The npm package asana receives a total of 41,857 weekly downloads. As such, asana popularity was classified as popular.
We found that asana demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.