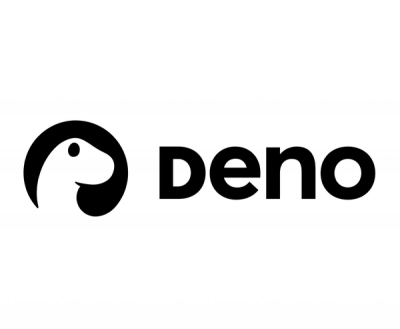
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Node client for Aptoma Smooth Storage
This module is installed via npm:
$ npm install ass-client
var ass = require('ass-client')({
httpUrl: 'http://ass.com',
httpsUrl: 'https://ass.com',
accessToken: 'secret',
username: 'foobar'
});
ass.uploadImage('my.jpg').then(function (res) {
console.log('Image uploaded', res.body);
var signedUrl = ass.createImageUrl(res.body.id, { resize: { width: 10, height: 10 } });
//create a signed url for the uploaded image in a different size
console.log('Smaller image', url);
})
Create ASS API instance
Parameters
opts: Object, Create ASS API instance
opts.httpUrl: String, Create ASS API instance
opts.httpsUrl: String, Create ASS API instance
opts.accessToken: String, Create ASS API instance
opts.username: String, Create ASS API instance
Get default headers
Parameters
add: Object, headers
Returns: Object,
Upload file to endpoint
Parameters
endpoint: String, Upload file to endpoint
file: String | stream.Readable, full path to the file or stream.Readable
Returns: Promise, Resolves with response object
Upload a file to /files endpoint
Parameters
file: String | stream.Readable, full path to the file or stream.Readable
Returns: Promise, Resolves with response object
Upload a
Parameters
file: String | stream.Readable, full path to the file or stream.Readable
Returns: Promise, Resolves with response object
Make a post request to an endpoint
Parameters
endpoint: String, Make a post request to an endpoint
Returns: Promise, Resolves with response object
Make a get request to an endpoint
Parameters
endpoint: String, Make a get request to an endpoint
Returns: Promise, Resolves with response object
Get full url to ASS endpoint, defaults to https url
Parameters
endpoint: String, Get full url to ASS endpoint, defaults to https url
http: Boolean, if we should return http url
Returns: String,
Create a signed url from image id and actions
Parameters
id: Integer, image id
actions: Object, Create a signed url from image id and actions
Returns: String,
Create signature for a image or file url
Parameters
url: String, Create transformation signature
Returns: String,
FAQs
Aptoma Smooth Storage client
The npm package ass-client receives a total of 4 weekly downloads. As such, ass-client popularity was classified as not popular.
We found that ass-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.